与线程pthread相关:
一、POSIX 多线程程序设计:https://blog.csdn.net/future_fighter/article/details/3865071#pthreads_overvie
Pthreads API中的函数可以非正式的划分为三大类:
(1)线程管理(Thread management): 第一类函数直接用于线程:创建(creating),分离(detaching),连接(joining)等等。包含了用于设置和查询线程属性(可连接,调度属性等)的函数。
(2)互斥量(Mutexes): 第二类函数是用于线程同步的,称为互斥量(mutexes),是"mutual exclusion"的缩写。Mutex函数提供了创建,销毁,锁定和解锁互斥量的功能。同时还包括了一些用于设定或修改互斥量属性的函数。
例程如下:
用四个线程计算a[i]*b[i]积的和dotstr.sum,四个线程分别计算 i的取值为 i =[0...99], [100...199], [200...299], [300...399]
#include <pthread.h>
#include <stdio.h>
#include <malloc.h>
#include <iostream>
using namespace std;
typedef struct
{
double *a;
double *b;
double sum;
int veclen;
} DOTDATA;
#define NUMTHRDS 4
#define VECLEN 10
DOTDATA dotstr;
pthread_t callThd[NUMTHRDS];
pthread_mutex_t mutexsum;
void *dotprod(void *arg)
{
int i, start, end, len ;
long offset;
double mysum, *x, *y;
offset = (long)arg;
len = dotstr.veclen;
start = offset*len;
end = start + len;
x = dotstr.a;
y = dotstr.b;
mysum = 0;
for (i=start; i<end ; i++)
{
mysum += (x[i] * y[i]);
}
pthread_mutex_lock (&mutexsum);
dotstr.sum += mysum;
cout << "dotstr.sum = " << dotstr.sum << endl;
pthread_mutex_unlock (&mutexsum);
pthread_exit((void*) 0);
}
int main (int argc, char *argv[])
{
long i;
double *a, *b;
void *status;
pthread_attr_t attr;
/* Assign storage and initialize values */
a = (double*) malloc (NUMTHRDS*VECLEN*sizeof(double));
b = (double*) malloc (NUMTHRDS*VECLEN*sizeof(double));
for (i=0; i<VECLEN*NUMTHRDS; i++)
{
a[i]=1.0;
b[i]=a[i];
}
dotstr.veclen = VECLEN;
dotstr.a = a;
dotstr.b = b;
dotstr.sum=0;
pthread_mutex_init(&mutexsum, NULL);
/* Create threads to perform the dotproduct */
pthread_attr_init(&attr);
pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_JOINABLE);
for(i=0; i<NUMTHRDS; i++)
{
pthread_create( &callThd[i], &attr, dotprod, (void *)i);
}
pthread_attr_destroy(&attr);
/* Wait on the other threads */
for(i=0; i<NUMTHRDS; i++)
{
pthread_join( callThd[i], &status);
}
/* After joining, print out the results and cleanup */
printf ("Sum = %f /n", dotstr.sum);
free (a);
free (b);
pthread_mutex_destroy(&mutexsum);
pthread_exit(NULL);
}
(3)条件变量(Condition variables):第三类函数处理共享一个互斥量的线程间的通信,基于程序员指定的条件。这类函数包括指定的条件变量的创建,销毁,等待和受信(signal)。设置查询条件变量属性的函数也包含其中。
二、参考https://blog.csdn.net/hyp1977/article/details/51505744
所有的线程都是平级的,根本不存在主线程和子线程。下文所述为了方便,将在main函数中的线程看做主线程,其它线程看成子线程,特此说明。先考虑以下代码:
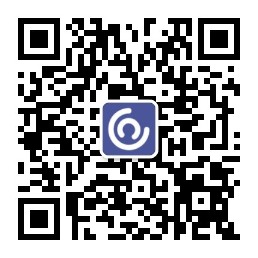
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void* thrd_start_routine(void* v)
{
sleep(10);
printf("created thread\n");
}
int main()
{
pthread_t thrdid;
pthread_create(&thrdid, NULL, &thrd_start_routine, NULL);
sleep(5);
printf("main thread\n");
return 0;
}
运行,结果是5s休眠后,打印“main thread";程序退出
之前误认为线程和进程一样,主线程退出,子线程退出。再考虑以下代码:
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void* thrd_start_routine(void* v)
{
sleep(10);
printf("created thread\n");
}
int main()
{ pthread_t thrdid;
pthread_create(&thrdid, NULL, &thrd_start_routine, NULL);
sleep(5);
printf("main thread\n");
pthread_exit(NULL);
printf("main exit\n");
return 0;
}
结果输出 main thread ;5s后输出 created thread;注意u,此处的main exit并不输出
相较于上一个程序,只加了一句 pthread_exit(NULL);
原因:
之前一个程序是在打印主线程之后,程序return,间接调用了exit()函数,因为一个线程调用exit函数,导致整个进程的退出,系统回收所有的资源,当然所有 的线程都退出了。
在主线程退出时,要想系统并不回收进程的所有资源,可以调用pthread_exit();然后等其他线程终止退出。