catalog
1、面向对象
2、类的继承(续):直接继承与间接继承
3、类方法、静态方法、属性方法
4、getitem
5、反射
6、_new_\_metaclass_
7、异常处理
1、面向对象
扫描二维码关注公众号,回复:
4037806 查看本文章
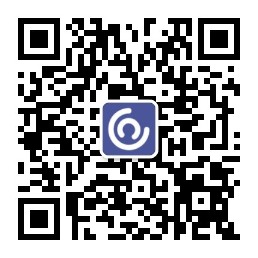
面向对象编程
class类(新式类:class xx(obj):,经典类 class xx:)
构造函数 __init__(self,ret1,ret2...) 在实例化时做一些类的初始化工作
析构函数 __del__ (self): 在实例释放或销毁的时候,通常做一些收尾工作,如关闭打开的临时文件、关闭数据库等
类变量
实例变量(静态属性)
方法(动态属性)
object对象
encapsulation 封装
私有方法
私有属性
Inheritance 继承
继承
继承、组合
多继承
python2x上
新式类 广度优先(从左到右,先走同级)
经典类 深度优先(竖向查询,先走最深级)
python3x上
都是广度优先
Polymorphism 多态
同一个接口多种实现
把一个类变成一个具体对象的过程叫实例化
实例想调用类里面的方法是把自己变成变量传给类,所以def(self)中的self即是定义的变量
实例变量的作用域就是实例本身
当实例变量与类变量名相同时,先找实例变量。
2、类的继承

1 class People(object): 2 def __init__(self,name): 3 self.name = name 4 def talk(self): 5 print('%s is talking...'% self.name) 6 7 p1 = People('alex') 8 9 #直接继承 10 class Men(People): 11 def __init__(self,name,age) 12 super(Men,self).__init__(self,name) 13 self.age = age 14 15 #间接继承 16 class Women(Object) 17 def __init__(self,name,obj) 18 self.name = name 19 self.people = obj 20 def talk(self): 21 print('This is inherit name [%s]'% self.people.name) 22 print('This is defined name[%s]'% self.name) 23 24 25 w1 = Women('wtl',p1) 26 w1.talk() 27 28 >>>>>>this is inherit name alex 29 >>>>>>this is defined name wtl 30 31
3、静态方法、类方法、属性方法
类方法:
@classmethod
只能访问类变量,不能访问实例变量
静态方法:
@staticmethod
静态方法,使该方法变为类下的一个函数,与类没什么太大关系
属性方法:
@property
@setter
@deleter
使一个方法变为属性
作用:隐藏过程细节,对客户友好。
特殊的运用:
def __call__(self,*args,**kwargs):#可以给实例传参数
code
def __str__(self):#将值返回给实例的门牌号
code
# print(Dog.__doc__) # __doc__输出类的描述信息
# print(Dog.__module__) # 描述类从哪里导入的
# print(Dog.__class__) #描述类本身
#
# print(Dog.__dict__) # 如果对象是类,打印类里面的所有属性,不包括实例属性
# print(d1.__dict__) # 如果对象是实例化对象,输出实例化对象的属性

1 class People(object): 2 name = 'Jinx' 3 def __init__(self,name): 4 self.name = name 5 self.__obj =None 6 7 @staticmethod #静态方法,传入完全不相干的参数 8 def eat(name,food): 9 print('%s is eating the %s'%(name,food)) 10 @staticmethod #传入self,在调用时需要把自己传进去 11 def sleep(self): 12 pritn('%s is sleeping.....'% self.name) 13 14 @classmethod#类方法,只能调用类的变量 15 def play(self): 16 print('%s is plaing...'% self.name) 17 18 @property #属性方法 19 def cof(self): 20 print('%s is eating with %s'%(self.name,self.__obj) 21 @cof.setter 22 def cof(self,food): 23 print('%s want to eat %s'%(self.name,food)) 24 self.__obj = food 25 @cof.deleter 26 def cof(self): 27 del self.__obj 28 print('删完了') 29 30 def __call__(self,*args,**kwargs): #可以给实例后面跟参数 31 print('call running:',args,kwargs) 32 33 def __str__(self): #给门牌号返回一个值 34 print('<obj>:',self.name) 35 36 37 p = People('alex') 38 p.eat('Jinx','regou') 39 p.sleep(p) 40 p.play() 41 p.cof 42 p.cof = 'kaochang' 43 del p.cof 44 d(1,2,3,'age' = 22,'salary' = 10000) 45 print(d)
4、getitem、setitem、delitem

1 class Dog(object): 2 '''描述狗的特性''' 3 name = 'right' 4 def __init__(self,name): 5 self.name = name 6 self.__obj = None 7 self.data = {} 8 9 def __getitem__(self, key): 10 print('in the getitem:',key) 11 return self.data.get(key) 12 13 def __setitem__(self, key, value): 14 print('Setitem:',key,value) 15 self.data[key] = value 16 17 18 def __delitem__(self, key): 19 print('__delitem__',key) 20 21 22 d1 = Dog('alex') 23 d1['age'] = 20 24 print(d1.data)
5、反射
setattr() # 创建方法
getattr() # 获取方法
delattr() # 删除方法 delattr(p,p.name)

1 class People(object): 2 def __init__(self,name,age): 3 self.name = name 4 self.age = age 5 def talk(self): 6 print('%s is talking'% self.name) 7 8 p = People('alex',20) 9 10 def eat(self): 11 print('eating with %s'% self.name) 12 13 choice = input('>>>:').strip() 14 15 # 方法的反射 16 if hasattr(p,choice): #如果存在,则运行函数 17 getattr(p,choice)() 18 else: # 如果不存在,将一个已有的函数创建为方法 19 setattr(p,choice,eat) #把eat这个函数付给choice,新建了一个choice的方法。 20 getattr(p,choice)(d) 21 22 #属性的反射 23 if hasattr(p,choice): 24 getattr(p,choice) 25 else: 26 setattr(p,choice,'rink') # choice为一个变量名,‘rink’为变量的值付给变量名 27 print(getattr(p,choice))
6、__new__ / __metaclass__
new方法,在创造实例的时候先于init实行。
必须要有return,这个return作用是:1、告诉要实例化的类 2、调用哪个类的new来实例化
参考:https://blog.csdn.net/ll83477/article/details/76419318

1 class A(object): 2 def __new__(cls, *args, **kwds): 3 print("one") 4 print ("A.__new__", args, kwds) 5 return object.__new__(B) 6 def __init__(cls, *args, **kwds): 7 print("two") 8 print ("A.__init__", args, kwds) 9 class B(object): 10 def __new__(cls, *args, **kwds): 11 print("three") 12 print(cls) 13 print(B) 14 print("B.__new__", args, kwds) 15 return object.__new__(cls) 16 def __init__(cls, *args, **kwds): 17 print ('four') 18 print ("B.__init__", args, kwds) 19 class C(object): 20 def __init__(cls, *args, **kwds): 21 print ("five") 22 print ("C.__init__", args, kwds) 23 print(C()) 24 print( "=====================") 25 print (A()) 26 print ("=====================") 27 print (B())
7、异常处理
异常处理
写法一
try:
code
except Error1 as e:
print e
except Error2 as e:
print e
...
except Exception as e: #Exception(所有的预判情况)但是通常放在最后处理没有预判的情况
print(未知错误,e)
else: #else 当程序正常运行时进行的操作
print(一切正常...)
finaly: #finaly 不管程序异常与否都继续往下走
code
写法2:(不建议使用)
try:
code
except (Error1,Error2....) as f:
print e
else:
print(一切正常...)
finaly:
code

1 AttributeError 试图访问一个对象没有的树形,比如foo.x,但是foo没有属性x 2 IOError 输入/输出异常;基本上是无法打开文件 3 ImportError 无法引入模块或包;基本上是路径问题或名称错误 4 IndentationError 语法错误(的子类) ;代码没有正确对齐 5 IndexError 下标索引超出序列边界,比如当x只有三个元素,却试图访问x[5] 6 KeyError 试图访问字典里不存在的键 7 KeyboardInterrupt Ctrl+C被按下 8 NameError 使用一个还未被赋予对象的变量 9 SyntaxError Python代码非法,代码不能编译(个人认为这是语法错误,写错了) 10 TypeError 传入对象类型与要求的不符合 11 UnboundLocalError 试图访问一个还未被设置的局部变量,基本上是由于另有一个同名的全局变量, 12 导致你以为正在访问它 13 ValueError 传入一个调用者不期望的值,即使值的类型是正确的 14 15 常用异常

1 a = 'alex' 2 b = [1,2,3] 3 tyr: 4 a[1] 5 b[10] 6 except KeyError as e: 7 print('关键字错误',e) 8 except ValueError as e: 9 print('值错误',e) 10 except Exception as e: 11 print('未知错误',e) 12 else: 13 print('go ahead') 14 finally: 15 print('不想理你')
抛出异常

1 #1\ 2 class SQError(Exception): 3 def __init__(self,msg): 4 self.mag = msg 5 try: 6 raise SQError('数据库处理错误') 7 except SQError as e: 8 print(e) 9 10 #2\ 11 # 自定义异常错误 12 class MyError(ValueError): 13 ERROR = ("-1", "没有该用户!") 14 15 16 # 抛出异常测试函数 17 def raiseTest(): 18 # 抛出异常 19 raise MyError(MyError.ERROR[0], # 异常错误参数1 20 MyError.ERROR[1]) # 异常错误参数2 21 22 23 # 主函数 24 if __name__ == '__main__': 25 try: 26 raiseTest() 27 except MyError as msg: 28 print("errCode:", msg.args[0]) # 获取异常错误参数1 29 print("errMsg:", msg.args[1]) # 获取异常错误参数2