Farmer John knows that an intellectually satisfied cow is a happy cow who will give more milk. He has arranged a brainy activity for cows in which they manipulate an M × N grid (1 ≤ M ≤ 15; 1 ≤ N ≤ 15) of square tiles, each of which is colored black on one side and white on the other side.
As one would guess, when a single white tile is flipped, it changes to black; when a single black tile is flipped, it changes to white. The cows are rewarded when they flip the tiles so that each tile has the white side face up. However, the cows have rather large hooves and when they try to flip a certain tile, they also flip all the adjacent tiles (tiles that share a full edge with the flipped tile). Since the flips are tiring, the cows want to minimize the number of flips they have to make.
Help the cows determine the minimum number of flips required, and the locations to flip to achieve that minimum. If there are multiple ways to achieve the task with the minimum amount of flips, return the one with the least lexicographical ordering in the output when considered as a string. If the task is impossible, print one line with the word "IMPOSSIBLE".
Input
Line 1: Two space-separated integers: M and N
Lines 2.. M+1: Line i+1 describes the colors (left to right) of row i of the grid with N space-separated integers which are 1 for black and 0 for whiteOutput
Lines 1.. M: Each line contains N space-separated integers, each specifying how many times to flip that particular location.
Sample Input
4 4 1 0 0 1 0 1 1 0 0 1 1 0 1 0 0 1
Sample Output
0 0 0 0 1 0 0 1 1 0 0 1 0 0 0 0
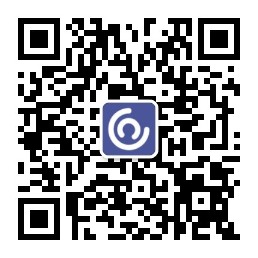
给一个N行M列的矩阵,值分别为0和1,每次你可以选择将一个变成相反状态,同时,它周围的四个数也会变为相反状态。
问:最少翻转多少次,可以将所有值都变成0
多个解,输出翻转次数最少的(若有次数相同解,输出字典序小的)
若无解,输出”IMPOSSIBLE”
思路
对于每个点,只能有两种操作,翻或不翻,若暴力所有可能性,需要2^(M*N)次操作,显然不可行
所以有了这个法子。
先枚举第一行的所有可能性(2^M),搜索或位运算均可
然后,对坐标(i, j)来说,如果(i-1, j)不为0,那么(i, j)必然需要翻转。
重复进行上操作由2至N
此时,最后一行也已翻转完毕,如果最后一行全为0,得出结果
第一行的所有结果中取最小值
代码
//难点在于通过对第一层的判定,从而推导出下一城的结果所以复杂度为O(15*(2^15) ) #include "bits/stdc++.h" using namespace std; int f[20][20],g[20][20]; int ans[20][20]; int minn=0x3f3f3f3f; bool judge(int n,int m) { for(int i=1;i<=m;i++) { if(( f[n][i]+f[n][i-1]+f[n][i+1]+f[n-1][i]+g[n][i])&1) return false; } return true; } void dfs(int n,int m,int k,int num) { int cnt=0; if(minn<num) { return; } if(k>n) { if(judge(n,m)&&minn>num) { memcpy(ans,f,sizeof(f)); minn = num; } return; } for(int i=1;i<=m;i++) { if((f[k-2][i]+f[k-1][i-1]+f[k-1][i+1]+f[k-1][i]+g[k-1][i])&1) { f[k][i]=1; cnt++; } else f[k][i]=0; } dfs(n,m,k+1,num+cnt); } //这段代码可以有意识的记忆,通过递归获取每一种可能性,但是用循环也是可以写的 /* int f[20]; for(int i=1;i<=(1<<n);i++) { for(int j=0;j<=14;j++) { if(i>>j&1) { f[j]=1; } else f[j]=0; } } */ void todfs(int n,int m,int k,int num) { if(k>m) { dfs(n,m,2,num); return; } f[1][k]=0;//没有反转 todfs(n,m,k+1,num); f[1][k]=1; todfs(n,m,k+1,num+1); f[1][k]=1; } int main() { int n,m; cin>>n>>m; for(int i=1;i<=n;i++) { for(int j=1;j<=m;j++) { cin>>g[i][j]; } } todfs(n,m,1,0); if(minn==0x3f3f3f3f) cout<<"IMPOSSIBLE"<<endl; else { for(int i=1;i<=n;i++) { for(int j=1;j<=m;j++) cout<<ans[i][j]<<' '; cout<<endl; } } return 0; }