需要2个jar包 com.google.zxing 下的core.jar 和 javase.jar
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.1</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId><artifactId>javase</artifactId>
<version>3.3.1</version>
</dependency>
java 代码
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Map;
import com.google.common.collect.Maps;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class QRCodeUtil {
private static final int width = 300;// 默认二维码宽度
private static final int height = 300;// 默认二维码高度
private static final String format = "png";// 默认二维码文件格式
private static final Map<EncodeHintType, Object> hints = Maps.newHashMap();// 二维码参数
static {
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");// 字符编码
// 容错等级 L、M、Q、H 其中 L 为最低, H 为最高
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.MARGIN, 2);// 二维码与图片边距
}
/**
* 返回一个 BufferedImage 对象
*
* @param content
* 二维码内容
*/
public static BufferedImage toBufferedImage(String content)
throws WriterException, IOException {
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height, hints);
return MatrixToImageWriter.toBufferedImage(bitMatrix);
}
/**
* 将二维码图片输出到一个流中
*
* @param content
* 二维码内容
* @param stream
* 输出流
*/
public static void writeToStream(String content, OutputStream stream)
throws WriterException, IOException {
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height, hints);
MatrixToImageWriter.writeToStream(bitMatrix, format, stream);
}
/**
* 生成二维码图片文件
*
* @param content
* 二维码内容
* @param path
* 文件保存路径
*/
public static void createQRCode(String content, String path)
throws WriterException, IOException {
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height, hints);
// toPath() 方法由 jdk1.7 及以上提供
MatrixToImageWriter.writeToPath(bitMatrix, format, new File(path).toPath());
}
public static void main(String[] args) {
try {
createQRCode("https://www.baidu.com", "D://1111.png");
} catch (Exception e) {
e.printStackTrace();
}
}
}
@RequestMapping("getQRCode.do")
public void getQRCodeImg(HttpServletRequest request, HttpServletResponse response) {
// 响应头
response.setDateHeader("expires", 0);
response.setHeader("Cache-control", "no-store,no-cache,must-revalidate");
response.addHeader("Cache-Control", "post-check=0,pre-check=0");
response.setHeader("pragma", "no-cache");
response.setContentType("image/jpeg");
String content= "www.baidu.com";
try {
// 响应流中绘制二维码
QRCodeUtil.writeToStream(content, response.getOutputStream());
} catch (Exception e) {
e.printStackTrace();
}
}
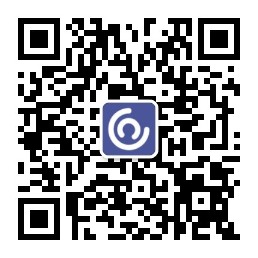
jsp
<body>
<img class="mt20 mb30" alt="二维码" src="/getQRCode.do"/>
</body>
-------------------------------------------------------这是分割线----------------------------------------------------------------
在二维码中插入logo图片
/**
* 生成二维码*
* @param content 二维码中需要写入的内容
* @param imgPath 二维码中间logo图片的地址
* @param output 输出流
* @param needCompress logo图片是否需要压缩
* @throws Exception
*/
public static void encode(String content, String imgPath, OutputStream output, boolean needCompress)
throws Exception {
BufferedImage image = createImage(content, imgPath, needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
/**
* 创建二维码
*/
private static BufferedImage createImage(String content, String imgPath, boolean needCompress) throws Exception {
Hashtable hints = new Hashtable();hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
// 设置字符集
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
// 设置边框
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, QRCODE_WIDTH,
QRCODE_HEIGHT, hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
//如果logo图片路径为空,则生成的二维码无logo
if (imgPath == null || "".equals(imgPath)) {
return image;
}
QRCodeCreator.insertImage(image, imgPath, needCompress);
return image;
}
/**
* 二维码中插入LOGO图片
*/
private static void insertImage(BufferedImage source, String imgPath, boolean needCompress) throws Exception {
File file = new File(imgPath);
if (!file.exists()) {
System.err.println("" + imgPath + " 该文件不存在!");
return;
}
Image src = ImageIO.read(new File(imgPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (needCompress) {
// 压缩LOGO
if (width > WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); // 绘制缩小后的图
g.dispose();
src = image;
}
// 插入LOGO
Graphics2D graph = source.createGraphics();
int x = (QRCODE_WIDTH - width) / 2;
int y = (QRCODE_HEIGHT - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
-------------------------------------------------------又是分割线^_^------------------------------------------
public void createWxQRCode() {
int width = 280;
int height = 280;
// 二维码的图片格式
Hashtable hints = new Hashtable();
// 内容所使用编码
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");
BufferedImage image = null;
ServletOutputStream out = null;
try {
// 二维码信息
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height,hints);
bitMatrix = deleteWhite(bitMatrix);
// 创建二维码
image = toBufferedImage(matrix);
try {
out = response.getOutputStream();
ImageIO.write(image, "gif", out);
image.flush();
out.flush();
} finally {
if (out != null) {
try {
out.close();
} catch (Exception e) {
}
}
}
} catch (Exception e) {
}
}
/**
* 去掉白边
*/
public static BitMatrix deleteWhite(BitMatrix matrix) {
int[] rec = matrix.getEnclosingRectangle();
int resWidth = rec[2] + 1;
int resHeight = rec[3] + 1;
BitMatrix resMatrix = new BitMatrix(resWidth, resHeight);
resMatrix.clear();
for (int i = 0; i < resWidth; i++) {
for (int j = 0; j < resHeight; j++) {
if (matrix.get(i + rec[0], j + rec[1]))
resMatrix.set(i, j);
}
}
return resMatrix;
}
public static BufferedImage toBufferedImage(BitMatrix matrix) {
int width = matrix.getWidth();
int height = matrix.getHeight();
BufferedImage image = new BufferedImage(width, height,
BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, matrix.get(x, y) ? BLACK : WHITE);
}
}
return image;
}
以上是本人亲测成功的,如有疑问请提出,共同进步,谢谢,逐渐完善。。。