C#字符串 String
C#中可以使用字符数组来表示字符串,但是,更常见的是使用string关键字来表示字符串变量。string关键字是System.String类的别名。
创建String对象
- 通过给String变量指定一个字符串
- 通过使用String类的构造函数
- 通过使用字符串串联运算符(+)
- 通过检索属性或调用一个返回字符串的方法
- 通过格式化方法来转换一个值或对象为它的字符串表示形式
在Visual Studio中新建C#控制台应用程序chapter09_001
在Main方法中添加如下代码:
//处理 姓名
string fname, lname;
Console.WriteLine("请输入姓:");
fname = Console.ReadLine();
Console.WriteLine("请输入名字:");
lname = Console.ReadLine();
//通过使用字符串串联运算符(+)
string fullname = fname + lname;
Console.WriteLine("姓名:"+fullname);
Console.WriteLine("请输入地址信息(按省市区街道明细分开录入,按q退出):");
string[] addr = new string[5];
for (int i = 0; i < addr.Length; i++) {
string temp = Console.ReadLine();
if (temp == "q")
{
break;
}
else {
addr[i] = temp;
}
}
//通过格式化方法来转换一个值或对象为它的字符串表示形式
string fulladdr = String.Join(".", addr);
Console.WriteLine("家庭地址:" + fulladdr);
//通过给String变量指定一个字符串
string school = "西安交通大学";
//通过转化值的格式指定一个字符串
DateTime inTime = new DateTime(2016,09,01);
String str_in = String.Format("入学时间:{0:D}", inTime);
Console.WriteLine("学校:"+school+" "+str_in);
//通过构造函数指定一个字符串
char[] leeters = { '踏', '踏', '实', '实', '做', '事', ',', '清', '清', '白', '白', '做', '人','。' };
string str = new string(leeters);
Console.WriteLine("座佑铭:"+str);
Console.ReadKey();
编译运行中要根据提示录入相关的信息运行后的结果如下:
String 类属性
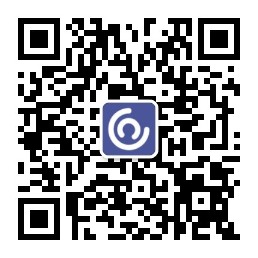
Chars:在当前String对象中获取Char对象的指定位置。
比如:
string str = "hello";
char ch1 = str[0]; // h
char ch2 = str[1]; // e
Length:在当前String对象中获取字符数。
String类的方法
public static int Compare(String strA,String strB)
比较两个指定string对象,并返回一个表示它们在排列顺序中相对位置的整数。这个方法是区分大小写的。
public static int Compare(String strA,String strB,bool ignoreCase)
比较两个指定string对象,并返回一个表示它们在排列顺序中相对位置的整数。当最后一个参数为真时,这个方法是不区分大小写的。
public static string Concat(String strA,String strB)
连接两个string对象并返回结果
public static string Concat(String strA,string strB,string strC)
连接三个String对象并返回结果
public static string Concat(String strA,string strB,string strC,string strD)
连接四个String对象并返回结果
public bool Contains(string value)
返回一个表示指定string对象是否出现在字符串中的值
public static string Copy(stirng str)
创建一个与指定字符串具有相同值的新string对象
public void CopyTo(int sourceIndex,char[] destination,int destinationIndex,int count)
从string对象的指定位置开始复制指定数量的字符到Unicode字符数组中指定位置
public bool EndsWith(string value)
判断string对象的结尾是否匹配指定字符串
public bool Equals(string value)
判断当前的string对象是否与指定string对象具有相同的值
public static bool Equals(string strA,string strB)
判断两个指定的string对象是否具有相同的值
public static string Format(string format,Object arg0)
指定指定符串中一个或多个格式项替换为指定对象的字符串表示形式
public int IndexOf(char value)
返回指定Unicode字符在当前字符串中第一次出现的索引,索引从0开始
public int IndexOf(string value)
返回指定字符串在该实例中第一出现的索引,索引从0开始
public int IndexOf(char value,int startIndex)
返回指定Unicode字符从该字符串中指定字符位置开始搜索第一出现的索引,索引从0开始
public int IndexOf(string value,int startIndex)
返回指定字符串从该字符串中指定字符位置开始搜索第一出现的索引,索引从0开始
public int IndexOfAny(char[] anyOf)
返回一个指定Unicode字符数组中任意字符在该实例中第一次出现的索引,索引从0开始
public int IndexOfAny(char[] anyOf,int startIndex)
返回一个指定的Unicode字符数组中任意字符从该实列中指定字符位置开始搜索第一次出现的索引,索引从0开始
public string Insert(int startIndex,string value)
返回一个新的字符串,其中指定的字符串被插入在当前string对象的指定索引位置
public static bool IsNullOrEmpty(string value)
指示指定字符串是否为null或者是否为一个空的字符串
public static string Join(string separator,params stirng[] vale)
连接一个字符串数组中的所有元素,使用指定的分隔符来分隔每一个元素
public int LastIndexOf(char value)
返回指定Unicode字符在当前string对象中最后一次出现的索引位置,索引从0开始
public int LastIndexOf(string value)
返回指定字符串在当前string对象中最后一次出的索引位置,索引从0开始
public string Remove(int startIndex)
移除当前字例中的所有字符,从指定的位置开始,一直到最后一个位置为止,并返回结果字符串
public string Remove(int startIndex,int count)
从当前字符串中指定位置开始移除指定数量的字符,并返回结果字符串
public string Replace(char oldChar,char newChar)
把当前string对象中,所有指定的Unicode字符替换为另一个指定的Unicode字符,并返回新的字符串
public string Replace(string oldValue,string newValue)
把当前string对象中,所有指定的字符串替换为另一个指定的字符串,并返回新的字符
public string[] Split(params char[] separator)
返回一个字符串数组,包含当前的string对象中的子字符串,子字符串是使用指定的Unicode字符数组中的元素进行分隔
public string[] Split(char[] separator,int count)
返回一个字符串数组,包含当前的string对象中的子字符串,子字符串是使用指定Unicode字符数组中的元素进行分隔的,后面的int参数是表示返回子字符串的最大数目
public bool StartWith(stirng value)
判断字符串实例开头是否匹配指定的字符串
public string Substring(int startIndex)
返回字符串从某个索引位置一直到结果的子字符串
public string Substring(int startIndex,int length)
返回字符串从某个索引位置开始并指定长度的子字符串
public char[] ToCharArray()
返回一个带有当前string对象中所有字符的Unicode字符数组
public char[] ToCharArray(int startIndex,int length)
返回一个带有当前string对象中所有字符的Unicode字符数组,从指定的索引开始,直到指定的长度为止
public string ToLower()
把字符串转为小写并返回
public string ToUpper()
把字符串转为大写并返回
public string Trim()
移除当前string对象中所有前导空白符和后置空白符
在Visual Studio中新建C#控制台应用程序Chapter09_002,验证字符串的属性和方法的使用
新建项目后,在Main方法中加入如下代码:
string str1 = "Test";
string str2 = "Text";
//使用Compare来进行两个字符串的比较
Console.WriteLine("-----------------使用Compare来进行两个字符串的比较--------------------");
if (String.Compare(str1, str2) == 0)
Console.WriteLine(str1 + " 与 " + str2 + " 字符串相同");
else
Console.WriteLine(str1 + " 与 " + str2 + " 字符串不相同");
Console.ReadKey();
编译运行后的结果如下:
使用Contains方法测试字符串中是否包含指定字符串
在Main方法中添加如下代码:
//使用Contains来测试字符串中是否包含指定字符串
Console.WriteLine("-----------------使用Contains来测试字符串中是否包含指定字符串--------------------");
string str = "My name is xiesheng";
if (str.Contains("name"))
Console.WriteLine("字符串:【{0}】中含有name", str);
else
Console.WriteLine("字符串:【{0}】中不含有name", str);
编译运行结果如下:
使用Substring方法来获取子字符串
在Main方法中添加如下代码:
//使用Substring方法来获取子字符串
Console.WriteLine("-----------------使用Substring方法来获取子字符串--------------------");
string substr = str.Substring(3);
Console.WriteLine("原字符串:{0},取得的子串:{1}", str, substr);
编译运行后的结果如下:
使用Join方法来连接成字符串
在Main方法中添加如下代码:
//使用Join来连接字符串
Console.WriteLine("-----------------使用Join来连接字符串--------------------");
string[] strArray = new string[] {
"My name is xiesheng",
"I\'m from China",
"I graduated from Xi'an Jiao Tong University",
"My major is computing science and technology"
};
string desStr = String.Join("\n", strArray);
Console.WriteLine(desStr);
编译运行后的结果如下:
在Main方法中添加如下代码:
//测试属性
Console.WriteLine("-----------------字符串属性测试--------------------");
string name = "xiesheng";
Console.WriteLine("字符串:【{0}】,第一个字符是:{1}", name, name[0]);
Console.WriteLine("字符串:【{0}】,总字符长度为:{1}", name, name.Length);
编译运行后结果如下: