BufferedInputStream和BufferedOutputStream
缓冲流字节的文件Copy
Test2.java 文件代码:
//缓冲字节流的文件Copy
public class Test1BufferedOutputStream {
public static void main(String[] args) throws IOException {
FileInputStream fis=new FileInputStream("fis.txt");
//缓冲字节输入流
BufferedInputStream bis=new BufferedInputStream(fis);//将低级流放入到高级流中
FileOutputStream fos=new FileOutputStream("fosCopy.txt");
//缓冲字节输出流
BufferedOutputStream bos=new BufferedOutputStream(fos);
int d=0;
while((d=bis.read())!=-1){//-1读取到文件末尾
bos.write(d);
}
bos.flush();//flush强制将缓冲区数据写出
bis.close();
bos.close();
}
}
字符输入流InputStreamReader
Test3.java 文件代码:
//字符输入流 InputStreamReader
public class Test3Reader {
public static void main(String[] args) throws IOException {
// TODO Auto-generated method stub
FileInputStream fis=new FileInputStream("osw.txt");
InputStreamReader isr=new InputStreamReader(fis,"GBK");
int chs=-1;
while((chs=isr.read())!=-1){//isr.read()以字符为单位进行读取,一个字符一个字符读
System.out.print((char)chs);
}
isr.close();
}
}
PrintWriter字符输出流
下面是一个例子:
Test4.java 文件代码:
//缓冲字符输出流
//output<-->write input<-->read
public class Test4PrintWriter {
public static void main(String[] args) throws IOException {
PrintWriter pw=new PrintWriter("pw.txt");//高级流 封装好的
pw.println("你好");
pw.println("我好");
pw.print("大家好");
pw.close();
//缓冲字符输出流
BufferedWriter bw=new BufferedWriter(new OutputStreamWriter(new FileOutputStream("bw.txt")));
bw.write("才是真的好");
bw.close();
}
}
BufferedReader
//缓冲字符输入流 BufferedReader
public class Test5BufferedReader {
public static void main(String[] args) throws IOException {
FileInputStream fis=new FileInputStream("pw.txt");//字节输入流 低级流
InputStreamReader isr=new InputStreamReader(fis);//字符输入流 高级流
BufferedReader br=new BufferedReader(isr);//缓冲字符输入流 高级流
String str=null;
while((str=br.readLine())!=null){//readLine() 一行一行读,读到文件末尾为null
System.out.println(str);
}
br.close();
}
}
Emp类
//员工类
//可以将对象转换成字节序列,写到文件中
public class Emp implements Serializable{//Serializable只能申明可序列化,里面没有常量和抽象方法
private String name;
private String gender;
private int age;
private double salary;
public Emp(String name, String gender, int age, double salary) {
super();
this.name = name;
this.gender = gender;
this.age = age;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + age;
result = prime * result + ((gender == null) ? 0 : gender.hashCode());
result = prime * result + ((name == null) ? 0 : name.hashCode());
long temp;
temp = Double.doubleToLongBits(salary);
result = prime * result + (int) (temp ^ (temp >>> 32));
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Emp other = (Emp) obj;
if (age != other.age)
return false;
if (gender == null) {
if (other.gender != null)
return false;
} else if (!gender.equals(other.gender))
return false;
if (name == null) {
if (other.name != null)
return false;
} else if (!name.equals(other.name))
return false;
if (Double.doubleToLongBits(salary) != Double
.doubleToLongBits(other.salary))
return false;
return true;
}
@Override
public String toString() {
return "Emp [name=" + name + ", gender=" + gender + ", age=" + age
+ ", salary=" + salary + "]";
}
}
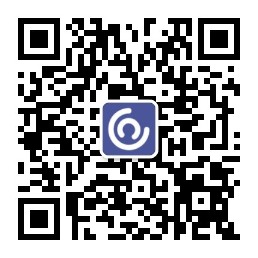
ObjectOutputStream对象输出流
public class Test6ObjectOutputStream{
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
Emp emp=new Emp("张三", "男", 20, 10000);
FileOutputStream fos=new FileOutputStream("emp.obj");
ObjectOutputStream oos=new ObjectOutputStream(fos);
//将对象转换成字节序列后输出
oos.writeObject(emp);
System.out.println("序列化完毕");
oos.close();
}
}
ObjectInputStream对象输入流
//对象输入流 读取字节并转换为对应的对象
public class Test7ObjectInputStream {
public static void main(String[] args) throws IOException, ClassNotFoundException {
FileInputStream fis=new FileInputStream("emp.obj");
ObjectInputStream ois=new ObjectInputStream(fis);
Emp emp=(Emp) ois.readObject();//readLine()返回Object,需要强制转换为Emp类型 向下造型
System.out.println("反序列化完毕");
System.out.println(emp);
ois.close();
}
}
java中常见的运行异常
//Java中常见异常
public class Test8Exception {
//Java单元测试
@Test
public void NumberFormatException(){//数字格式异常
int num=Integer.parseInt("一百");//不可以
}
@Test
public void ClassCastException(){//类型转换异常
Object obj="hello";
Integer i=(Integer) obj;
}
@Test
public void ArithmeticException(){//数学异常
System.out.println(5/0);//在数学中0不能作为分母
}
@Test
public void ArrayIndexOutOfBoundsException(){//数组下标越界异常
byte[] bytes="".getBytes();//把空字符串变成字节数组
System.out.println(bytes[0]);//数组里没有元素
}
@Test
public void NullPointerException(){//空指针异常
String str=null;
System.out.println(str.length());//看点前面的引用是否为空
}
}
try-catch捕获异常
//使用try-catch捕获异常
/*try{
* 可能出现的异常代码块
* }catch(Exception e){//列举代码中可能出现的异常
* 当出现异常后,在此处处理
* }
*/
public class Test9Exception {
public static void main(String[] args) {
// TODO Auto-generated method stub
try{
String str=null;
System.out.println("这句话出现异常:"+str.length());
System.out.println("字符串下标越界异常:"+str.charAt(0));
}catch(NullPointerException e){
System.out.println("在此处理异常");
}catch(StringIndexOutOfBoundsException e){
System.out.println("字符串下标越界");
}
//try-catch捕获完对应的异常后,后续的代码还会执行
System.out.println("下面这句话还能执行到吗");
}
}
finally关键字
//finally关键字
public class Test11Exception {
public static void main(String[] args) {//main方法JVM调用
try{
String str=null;
if(str==null){
return;//返回数据 结束方法
}
System.out.println(str.length());
}catch(Exception e){
e.printStackTrace();
}finally{//此代码块中代码一定会执行,一般在此代码块中执行关闭流操作
System.out.println("finally中代码");
}
System.out.println("会不会执行");
}
}
try-catch捕获异常
//使用try-catch捕获异常
/*try{
* 可能出现的异常代码块
* }catch(Exception e){//列举代码中可能出现的异常
* 当出现异常后,在此处处理
* }
*/
public class Test9Exception {
public static void main(String[] args) {
// TODO Auto-generated method stub
try{
String str=null;
System.out.println("这句话出现异常:"+str.length());
System.out.println("字符串下标越界异常:"+str.charAt(0));
}catch(NullPointerException e){
System.out.println("在此处理异常");
}catch(StringIndexOutOfBoundsException e){
System.out.println("字符串下标越界");
}
//try-catch捕获完对应的异常后,后续的代码还会执行
System.out.println("下面这句话还能执行到吗");
}
}