You are given N points in a 2-dimensional cartesian coordinate system. We call point A is in the control of point B if and only if point A lies inside or on the border of the rectangle diagonaled by the segment between point B and the origin. Formally, A is in the control of B iff. 0 <= xA <= xB and 0 <= yA <= yB where (xA, yA) and (xB, yB) are the cartesian coordinates of point A and B, respectively.
For each of the given point, please calculate the number of points given that are in control of the point.
Input
The first line of input file contains a single integer N (1 <= N <= 100’000), indicating the number of points.
Each of the next N lines contains two space-separated integers x and y (1 <= x, y <= 1’000’000’000), indicating the coordinate of the point.
Output
Your output should contain N lines. The i-th line should contain a single integer, indicating the number of points given that are in control of the i-th point given in the input.
Notes
For the sample test case given, point (1, 1) and point (3, 2) are in the control of point (3, 2); point (1, 1) and point (2, 3) are in the control of point (2, 3); point (1, 1) is in the control of point (1, 1).
测试用例
in:
3
3 2
1 1
2 3
out:
2↵
1↵
2↵
本来想用归并的,然而周五老师讲了一下树状数组发现这东西挺好用的(真香),于是就用了树状数组。首先对x离散化,然后对y优先于x进行排序,将离散化的x插入树状数组里面再进行求和。最后处理下重复点的情况,对y优先于x优先于ans(结果)排序,将相同坐标的点的答案统一变成答案大的。最后按id排序输出结果。
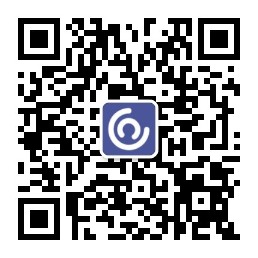
#include<stdio.h>
#include<stdlib.h>
#define N 100005
typedef struct node
{
int x;
int y;
int unix;
int id;
int ans;
}point;
typedef struct temp
{
int val;
int id;
}Temp;
point p[N];
Temp a[N];
int n,unia[N],sum[N];
int cmp1(const void *a,const void *b)
{
Temp A=*(struct temp *)a;
Temp B=*(struct temp *)b;
return A.val-B.val;
}
int cmp2(const void *a,const void *b)
{
point A=*(struct node *)a;
point B=*(struct node *)b;
if(A.y!=B.y)
return A.y-B.y;
return A.x-B.x;
}
int cmp3(const void *a,const void *b)
{
point A=*(struct node *)a;
point B=*(struct node *)b;
return A.id-B.id;
}
int cmp4(const void *a,const void *b)
{
point A=*(struct node *)a;
point B=*(struct node *)b;
if(A.y!=B.y)
return A.y-B.y;
else if (A.x!=B.x)
return A.x-B.x;
return B.ans-A.ans;
}
int lowbit(int index)
{
return index&(-index);
}
void update(int index,int d)
{
while(index<=n)
{
sum[index]=sum[index]+d;
index=index+lowbit(index);
}
}
int getsum(int index)
{
int res=0;
while(index>0)
{
res=res+sum[index];
index=index-lowbit(index);
}
return res;
}
int main()
{
scanf("%d",&n);
for(int i=0;i<n;i++)
{
scanf("%d%d",&p[i].x,&p[i].y);
p[i].id=i;
p[i].ans=0;
a[i].val=p[i].x;
a[i].id=i;
unia[i]=0;
}
qsort(a,n,sizeof(a[0]),cmp1);
unia[a[0].id]=1;
int cnt=1;
for(int i=1;i<n;i++)
{
if(a[i].val==a[i-1].val)
unia[a[i].id]=unia[a[i-1].id];
else
unia[a[i].id]=++cnt;
}
for(int i=0;i<n;i++)
p[i].unix=unia[p[i].id];
qsort(p,n,sizeof(p[0]),cmp2);
for(int i=0;i<n;i++)
{
update(p[i].unix,1);
p[i].ans=getsum(p[i].unix);
}
qsort(p,n,sizeof(p[0]),cmp4);
for(int i=0;i<n-1;i++)
{
if(p[i].x==p[i+1].x&&p[i].y==p[i+1].y)
p[i+1].ans=p[i].ans;
}
qsort(p,n,sizeof(p[0]),cmp3);
for(int i=0;i<n;i++)
printf("%d\n",p[i].ans);
return 0;
}