元素添加方法
*注意两者之间的区别:prepend()方法,添加的位置是元素的第一个子元素
*before()、after()方法添加的兄弟节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
div{
width: 300px;
height: 200px;
border:1px solid red;
}
</style>
<script src="jquery-1.12.2.js"></script>
<script>
$(function () {
//点击按钮,创建元素
$("#btn").click(function () {
//把创建的span标签这个子元素直接插入到div中第一个子元素的前面
$("#dv").prepend($("<span>this is span</span>"));
//主动的方式
//$("<span>this is span</span>").prependTo($("#dv"));
//把元素添加到div的后面的位置,是div的下一个兄弟元素了
//$("#dv").after($("<span>this is span</span>"));
//把元素添加到div的前面的位置,是div的上一个兄弟元素了
//$("#dv").before($("<span>this is span</span>"));
});
});
</script>
</head>
<body>
<input type="button" value="创建一个按钮" id="btn"/>
<div id="dv">
<p>这是一个p</p>
</div>
<p>这是div后面的第一个子元素</p>
</body>
</html>
元素的移除
*empty()只是清空,remove()方法是自杀。。。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
div{
width: 200px;
height: 100px;
border: 2px solid red;
}
</style>
<script src="jquery-1.12.2.js"></script>
<script>
//页面加载
$(function () {
//点击按钮
$("#btn").click(function () {
//从父级元素中移除子级元素---移除所有的子元素
//$("#dv").html("");
//清空父元素中的子元素
$("#dv").empty();//清空
//想要干掉谁,直接找到这个元素,调用这个方法就可以了
//$("#dv").remove();//自杀---
});
});
</script>
</head>
<body>
<input type="button" value="移除元素" id="btn"/>
<div id="dv">
<p>这是一个p</p>
<span>这是一个span</span>
</div>
</body>
</html>
案例:权限的设置
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
</style>
<script src="jquery-1.12.2.js"></script>
<script>
//页面加载
$(function () {
$("#toright").click(function () {
$('#se1>option:selected').appendTo($('#se2'));
});
$("#toleft").click(function () {
$('#se2>option:selected').appendTo($('#se1'));
});
$("#toallright").click(function () {
$('#se1>option').appendTo($('#se2'));
});
$("#toAllLeft").click(function () {
$('#se2>option').appendTo($('#se1'));
});
});
</script>
</head>
<body>
<div style="margin-left:500px;margin-top:20px;">
<select multiple="multiple" style="float:left;width: 60px;height: 100px;" id="se1">
<option>添加</option>
<option>删除</option>
<option>修改</option>
<option>查询</option>
<option>打印</option>
</select>
<div style="width: 50px;float: left;">
<input type="button" name="name" value=">" style="width:50px;" id="toRight">
<input type="button" name="name" value="<" style="width:50px;" id="toLeft">
<input type="button" name="name" value=">>" style="width:50px;" id="toAllRight">
<input type="button" name="name" value="<<" style="width:50px;" id="toAllLeft">
</div>
<select multiple="multiple" style="float:left;width: 60px;height: 100px;" id="se2">
</select>
</div>
</body>
</html>
value属性的获取和设置
*select那个标签选中的方式有点怪异。。。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.2.js"></script>
<script>
$(function () {
$("#btn").click(function () {
//点击按钮,获取文本框的value属性,和设置
console.log($("#txt").val());
$("#txt").val("今天天气一点也不好");
//点击按钮设置单选框的value属性
//$("#r2").val("哦天啊"); 设置标签的value属性
//$("#ck3").val("改了");
//获取文本域中的value值
console.log($("#tt2").val()); 这两种方式都可以获取文本域中的值
console.log($("#tt2").text());
//设置文本域中的文本内容--可以设置的
$("#tt2").val("小苏好猥琐哦,哈哈");
//推荐使用下面的方式-----jQuery的写法
$("#tt2").text("好神奇哦"); 文本推荐方法
//为select标签中value属性是5的这个option标签选中
//$("#s1").val(5);//选中的意思,
});
});
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn"/>
<input type="text" value="今天天气很好" id="txt" /><br/>
<input type="radio" value="1" name="sex"/>男
<input type="radio" value="2" name="sex" id="r2"/>女
<input type="radio" value="3" name="sex"/>保密
<br/>
<input type="checkbox" value="1" />吃饭
<input type="checkbox" value="2" />睡觉
<input type="checkbox" value="3" id="ck3"/>打豆豆
<input type="checkbox" value="4" />打铅球
<br/>
<textarea name="tt" id="tt2" cols="30" rows="10">
hello world!
</textarea>
<select id="s1">
<option value="1">妲己</option>
<option value="2">王昭君</option>
<option value="3">西施</option>
<option value="4">貂蝉</option>
<option value="5">小乔</option>
<option value="6">大乔</option>
<option value="7">武则天</option>
</select>
</body>
</html>
元素选中的问题
//DOM中操作
//document.getElementById("r3").checked=true;
//jQuery操作
//$("#r3").get(0).checked=true;//DOM的写法
获取:
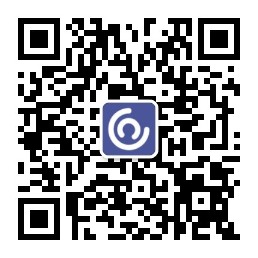
*注:attr方法针对单选框或者是复选框的是否选中问题,操作起来很麻烦,几乎不用,后面说
//获取是否被选中了
//console.log($("#r3").attr("checked")); 未选中默认为 undefined
//同样也可以通过这种方式来进行值的设置
//$("#r3").attr("checked",true);//设置
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
$("#btn").click(function () {
选择性别之后,点击选中
if($("#r3").attr("checked")==undefined){
//undefined
$("#r3").attr("checked",true);
console.log("哈哈");
}else{
//checked--->选中了
$("#r3").attr("checked",false);
console.log("嘎嘎");
}
});
});
</script>
</head>
<body>
<input type="button" value="选中" id="btn"/><br/>
请选择小苏的性别:
<input type="radio" value="1" name="sex"/>男
<input type="radio" value="2" name="sex"/>女
<input type="radio" value="3" name="sex" id="r3"/>人妖
</body>
</html>
自定义属性
* .attr(参数1,参数2);-----设置某个属性的值
* .attr(参数1);-----获取某个属性的值
*
* 参数1:属性的名字
* 参数2:属性的值
*
* .attr方法主要是操作元素的自定义属性的,但是也可以操作元素的自带的属性(html中本身就有的属性),但是:操作元素的选中的ckeched的时候,不是很合适!
* 操作元素的选中的checked的时候,推荐使用prop方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
$("#btn").click(function () {
//$("#uu>li").attr("score",100);
$("#ak").attr("href","http://www.baidu.com");
$("#ak").text("百度");
});
});
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn"/>
<a id="ak"></a>
<ul id="uu">
<li>小白</li>
<li>小黑</li>
<li>小红</li>
<li>小绿</li>
</ul>
</body>
</html>
设置或者是获取元素的选中的问题
* 推荐使用prop()方法
* .prop("属性",值); 值一般是布尔类型----->目前这么写代码是设置选中
* .prop("属性");-----获取这个元素是否选中
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
//点击按钮
$("#btn").click(function () {
// $("#ck7").attr("checked",true);
//获取复选框是否选中
//console.log($("#ck7").prop("checked"));
$("#ck7").prop("checked", true);
});
});
</script>
</head>
<body>
<input type="button" value="选中/不选中" id="btn"/>
<input type="checkbox" value="1" name="play"/>吃饭
<input type="checkbox" value="6" name="play"/>打铅球
<input type="checkbox" value="7" name="play" id="ck7"/>打小苏
</body>
</html>
案例:选中和不选中切换
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
//点击按钮
$("#btn").click(function () {
//点一下,选中了,再点,就不再选中了,
if($("#ck").prop("checked")){
//选中了,就应该变成不是选中的状态
$("#ck").prop("checked",false);
}else{
$("#ck").prop("checked",true);
}
});
});
</script>
</head>
<body>
<input type="button" value="选中/不选中" id="btn"/>
<input type="checkbox" value="1" name="play" id="ck" />玩游戏
</body>
</html>
案例:全选/全不选
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
* {
padding: 0;
margin: 0;
}
.wrap {
width: 300px;
margin: 100px auto 0;
}
table {
border-collapse: collapse;
border-spacing: 0;
border: 1px solid #c0c0c0;
}
th,
td {
border: 1px solid #d0d0d0;
color: #404060;
padding: 10px;
}
th {
background-color: #09c;
font: bold 16px "微软雅黑";
color: #fff;
}
td {
font: 14px "微软雅黑";
}
tbody tr {
background-color: #f0f0f0;
}
tbody tr:hover {
cursor: pointer;
background-color: #fafafa;
}
</style>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
//thead标签中的复选框----全选和全不选
$("#j_cbAll").click(function () {
//直接设置tbody中复选框的选中状态和当前点击的复选框的选中状态一致
$("#j_tb").find("input").prop("checked",$(this).prop("checked"));
});
//每个复选框都要注册点击事件
$("#j_tb").find("input").click(function () {
var ckLength=$("#j_tb").find("input").length;
var checkedLenth=$("#j_tb :checked").length;
$("#j_cbAll").prop("checked",checkedLenth==ckLength);
});
});
</script>
</head>
<body>
<div class="wrap">
<table>
<thead>
<tr>
<th>
<input type="checkbox" id="j_cbAll"/>
</th>
<th>课程名称</th>
<th>所属学院</th>
</tr>
</thead>
<tbody id="j_tb">
<tr>
<td>
<input type="checkbox"/>
</td>
<td>JavaScript</td>
<td>前端与移动开发学院</td>
</tr>
<tr>
<td>
<input type="checkbox"/>
</td>
<td>css</td>
<td>前端与移动开发学院</td>
</tr>
<tr>
<td>
<input type="checkbox"/>
</td>
<td>html</td>
<td>前端与移动开发学院</td>
</tr>
<tr>
<td>
<input type="checkbox"/>
</td>
<td>jQuery</td>
<td>前端与移动开发学院</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
设置元素宽高的两种方式
*数值属性的设置,一定要注意获取的属性值的数据类型。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
div{
width: 200px;
height: 100px;
background-color: darkorchid;
}
</style>
<script src="jquery-1.12.1.js"></script>
<script>
//元素的宽和高,jQuery中封装了方法
/*
* .width()可以获取也可以设置元素的宽
* .height()可以获取也可以设置元素的高
*
*
* */
//把获取元素计算后的样式属性值的兼容代码:写两遍
$(function () {
$("#btn").click(function () {
//点击按钮,设置div的宽和高为原来的2倍
//.css方法获取的宽和高实际上是字符串类型
//获取div的宽和高
// var w=$("#dv").css("width");
// var h=$("#dv").css("height");
// //设置div的宽和高
// $("#dv").css("width",(parseInt(w)*2)+"px");
// $("#dv").css("height",(parseInt(h)*2)+"px");
//先获取
// var w=$("#dv").width();
// var h=$("#dv").height();
// console.log(w);
// $("#dv").css("width",w*2);
// $("#dv").css("height",h*2);
// $("#dv").width(300);
// $("#dv").height(400);
});
});
</script>
</head>
<body>
<input type="button" value="设置元素的宽和高" id="btn" />
<div id="dv"></div>
</body>
</html>
设置元素的位置
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
*{
margin: 0;
padding: 0;
}
div{
width: 200px;
height: 100px;
background-color: indianred;
position: absolute;
left:100px;
top:200px;
}
</style>
<script src="jquery-1.12.1.js"></script>
<script>
//点击按钮,设置div的left和top的值是原来的2倍
$(function () {
$("#btn").click(function () {
//获取left和top ---获取的仍然是字符串类型
// var l=$("#dv").css("left");
// var t=$("#dv").css("top");
// //console.log(l);
// var left1=parseInt(l)*2;
// var top1=parseInt(t)*2;
// $("#dv").css("left",left1+"px");
// $("#dv").css("left",top1+"px");
//该方法获取的是一个对象,该对象中有两个属性,left和top
//left和top包含left和margin-left和top和margin-top的值
//console.log($("#dv").offset().left);
$("#dv").css("left",$("#dv").offset().left*2);
$("#dv").css("top",$("#dv").offset().top*2);
$("#dv").offset({"left":500,"top":250});
});
});
/*
* 可以设置,也可以获取
* .width()是元素的宽
* .height()是元素的高
*
* .offset()--->获取的是对象,可以设置,也可以获取
* .offset({"left":值,"top":值});设置
*
*
* */
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn"/>
<div id="dv"></div>
</body>
</html>
scroll系列:卷取值的获取
/*
* DOM中的
* scrollLeft:向左卷曲出去的距离的值
* scrollTop:向上卷曲出去的距离的值
* scrollWidth:元素中内容的实际的宽
* scrollHeight:元素中内容的实际的高
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
div{
width: 300px;
height: 200px;
border: 1px solid red;
overflow: auto;
}
</style>
<script src="jquery-1.12.1.js"></script>
<script>
//div滚动上下滚动条的时候,一直获取他的向上卷曲出去的值
$(function () {
$("#dv").scroll(function () {
console.log($(this).scrollTop()); 其他的值获取方式类似
});
});
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn"/>
<div id="dv">
hello world!
...more...
</div>
</body>
</html>
为元素绑定事件的5中写法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
//为按钮绑定鼠标进入,鼠标离开,点击事件
//第一种写法
// $("#btn").mouseenter(function () {
// $(this).css("backgroundColor","red");
// });
// $("#btn").mouseleave(function () {
// $(this).css("backgroundColor","green");
// });
// $("#btn").click(function () {
// alert("啊~我被点了");
// });
//第二种写法:链式编程
// $("#btn").mouseenter(function () {
// $(this).css("backgroundColor","red");
// }).mouseleave(function () {
// $(this).css("backgroundColor","green");
// }).click(function () {
// alert("啊~我被点了");
// });
//第三种写法:bind方法绑定事件
// $("#btn").bind("click",function () {
// alert("哦买雷电嘎嘎闹");
// });
// $("#btn").bind("mouseenter",function () {
// $(this).css("backgroundColor","red");
// });
// $("#btn").bind("mouseleave",function () {
// $(this).css("backgroundColor","green");
// });
//第四种写法:链式编程
// $("#btn").bind("click",function () {
// alert("哦买雷电嘎嘎闹");
// }).bind("mouseenter",function () {
// $(this).css("backgroundColor","red");
// }).bind("mouseleave",function () {
// $(this).css("backgroundColor","green");
// });
//第五种写法:使用键值对的方式绑定事件
// $("#btn").bind({"click":function () {
// alert("哦买雷电嘎嘎闹");
// },"mouseenter":function () {
// $(this).css("backgroundColor","red");
// },"mouseleave":function () {
// $(this).css("backgroundColor","green");
// }});
});
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn" />
</body>
</html>
为元素绑定多个事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<script src="jquery-1.12.1.js"></script>
<script>
$(function () {
//为按钮绑定多个相同事件 链式编程绑定多个事件,可以避免覆盖
// $("#btn").click(function () {
// console.log("小苏好猥琐哦");
// }).click(function () {
// console.log("小苏好美啊");
// }).click(function () {
// console.log("天冷了,注意身体");
// });
// $("#btn").bind("click",function () {
// console.log("哈哈,我又变帅了");
// }).bind("click",function () {
// console.log("hello world!");
// });
//bind方法绑定多个相同的事件的时候,如果使用的是键值对的方式,只能执行最后一个
$("#btn").bind({"click":function () {
console.log("oh my god!");
},"click":function () {
console.log("you jump i jump");
}});
});
//bind方法内部是调用的另一个方法绑定的事件
</script>
</head>
<body>
<input type="button" value="显示效果" id="btn"/>
</body>
</html>
另类的绑定事件:父元素被点击,子元素绑定事件。。。
* 对象.事件名字(事件处理函数);---$("#btn").click(function(){});
* 对象.bind("事件名字",事件处理函数);---$("#btn").bind("click",function(){});
* 父级对象.delegate("子级元素","事件名字",事件处理函数);---->$("#dv").delegate("p","click",function(){});
*
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>title</title>
<style>
div{
width: 200px;
height: 100px;
border: 2px solid green;
}
p{
width: 150px;
height: 50px;
border: 1px solid red;
cursor: pointer;
}
</style>
<script src="jquery-1.12.1.js"></script>
<script>
//点击按钮为div中的p标签绑定事件
$(function () {
$("#btn").click(function () {
//为父级元素绑定事件,父级元素代替子级元素绑定事件
//子级元素委托父级绑定事件
//父级元素调用方法,为子级元素绑定事件
$("#dv").delegate("p","click",function () {
alert("啊!~,被点了");
});
});
});
</script>
</head>
<body>
<input type="button" value="为div绑定事件" id="btn" />
<div id="dv">
<p>这是p</p>
</div>
</body>
</html>
案例:固定导航栏jQuery实现
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>防腾讯固定导航栏</title>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
.main {
width: 1000px;
margin: 0 auto;
}
/*.top {
height: 171px;
}
.nav {
height: 86px;
}*/
</style>
<script src="jquery-1.12.2.js"></script>
<script>
$(function () {
//浏览器的滚动条上下移动的时候,设置页面中的nav的div固定在页面的顶部或者是回到原来的位置
//为浏览器注册滚动的事件
$(window).scroll(function () {
//获取页面的向上卷曲出去的距离的值
//如果向上卷曲出去的距离的值大于或者是等于第一个div的高度
if($(this).scrollTop()>=$(".top").height()){
//设置导航栏固定在页面的顶部
$(".nav").css("position","fixed");
//设置导航栏在顶部的值是0
$(".nav").css("top",0);
//设置.main的div的位置,不是直接跳上去的
$(".main").css("marginTop",$(".nav").height());
}else{
//恢复
$(".nav").css("position","static");
$(".main").css("marginTop",0);
}
});
});
</script>
</head>
<body>
<div class="top">
<img src="images/top.png" />
</div>
<div class="nav">
<img src="images/nav.png" />
</div>
<div class="main">
<img src="images/main.png" />
</div>
</body>
</html>