21. 合并两个有序链表
将两个有序链表合并为一个新的有序链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。
示例:
输入:1->2->4, 1->3->4
输出:1->1->2->3->4->4
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* ll = new ListNode(-1);
ll->next = NULL;
ListNode* mv = ll;
while (l1 != NULL && l2 != NULL) {
if (l1->val <= l2->val) {
mv->next = l1;
l1 = l1->next;
} else {
mv->next = l2;
l2 = l2->next;
}
mv = mv->next;
}
if (l1 != NULL){
mv->next = l1;
} else if(l2 != NULL){
mv->next = l2;
}
return ll->next;
}
};
22. 括号生成
给出 n 代表生成括号的对数,请你写出一个函数,使其能够生成所有可能的并且有效的括号组合。
例如,给出 n = 3,生成结果为:
[
"((()))",
"(()())",
"(())()",
"()(())",
"()()()"
]
class Solution {
public:
vector<string> generateParenthesis(int n) {
vector<string> res;
match("",res,n,0,0);
return res;
}
void match(string s,vector<string>& res,int n,int left,int right) {
if (right == n) {
res.push_back(s);
}
if (left < n){
match(s+"(",res,n,left+1,right);
}
if (left > right) {
match(s+")",res,n,left,right+1);
}
}
};
23. 合并K个排序链表
合并 k 个排序链表,返回合并后的排序链表。请分析和描述算法的复杂度。
示例:
输入:
[
1->4->5,
1->3->4,
2->6
]
输出: 1->1->2->3->4->4->5->6
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* mergeKLists(vector<ListNode*>& lists) {
ListNode* dummyHead = new ListNode(-1);
ListNode* res = merge(dummyHead, dummyHead, lists)->next;
delete dummyHead;
return res;
}
public:
ListNode* merge(ListNode* head, ListNode* tail, vector<ListNode*>& lists){
int minIndex = findMinHead(lists);
if (minIndex == -1){
tail->next = NULL;
return head;
}
tail->next = lists[minIndex];
lists[minIndex] = lists[minIndex]->next;
return merge(head, tail->next, lists);
}
public:
int findMinHead(vector<ListNode*>& lists){
int minIndex = -1;
for(int i=0; i<lists.size(); i++){
if(lists[i] == NULL){
lists.erase(lists.begin()+i);
i--;
continue;
}
if(minIndex == -1 || lists[i]->val < lists[minIndex]->val){
minIndex = i;
}
}
return minIndex;
}
};
24. 两两交换链表中的节点
给定一个链表,两两交换其中相邻的节点,并返回交换后的链表。
扫描二维码关注公众号,回复:
3379490 查看本文章
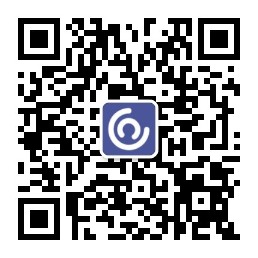
示例:
给定1->2->3->4
, 你应该返回2->1->4->3
.
说明:
- 你的算法只能使用常数的额外空间。
- 你不能只是单纯的改变节点内部的值,而是需要实际的进行节点交换。
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
ListNode* dummyHead = new ListNode(-1);
dummyHead->next = head;
head = dummyHead;
while (head->next != NULL && head->next->next != NULL){
ListNode* firstNode = head->next;
ListNode* secondNode = firstNode->next;
head->next = secondNode;
firstNode->next = secondNode->next;
secondNode->next = firstNode;
head = firstNode;
}
return dummyHead->next;
}
};
25. k个一组翻转链表
给出一个链表,每 k 个节点一组进行翻转,并返回翻转后的链表。
k 是一个正整数,它的值小于或等于链表的长度。如果节点总数不是 k 的整数倍,那么将最后剩余节点保持原有顺序。
示例 :
给定这个链表:1->2->3->4->5
当 k = 2 时,应当返回: 2->1->4->3->5
当 k = 3 时,应当返回: 3->2->1->4->5
说明 :
- 你的算法只能使用常数的额外空间。
- 你不能只是单纯的改变节点内部的值,而是需要实际的进行节点交换。
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode* pre;
ListNode* p = head;
ListNode* q = head;
if(!p || k == 1) return p;
for(int i = 1; i < k; i ++){
if(!q->next) return head;
q = q->next;
}
pre = p;
head = q;
while(p->next != head){
q = p->next;
p->next = p->next->next;
q->next = pre;
pre = q;
}
p->next = reverseKGroup(head->next, k);
head->next = pre;
return head;
}
};
(以上题目均摘自leetcode)