Given two strings s and t, determine if they are isomorphic.
Two strings are isomorphic if the characters in s can be replaced to get t.
All occurrences of a character must be replaced with another character while preserving the order of characters. No two characters may map to the same character but a character may map to itself.
Example 1:
Input: s ="egg",
t ="add"
Output: true
Example 2:
Input: s ="foo",
t ="bar"
Output: false
Example 3:
Input: s ="paper",
t ="title"
Output: true
Note:
You may assume both s and t have the same length.
给定两个字符串s和t,判断它们是否是同构的。如果字符串s可以通过字符替换的方式得到字符串t,则称s和t是同构的。字符的每一次出现都必须被其对应字符所替换,同时还需要保证原始顺序不发生改变。两个字符不能映射到同一个字符,但是字符可以映射到其本身。
解法1: 将字符串全部输出成数字序列, Given "paper", return "01023". Given "isomorphic", return "0123245607". 最后在比较两个数字是否相等。超时不能Accept。
解法2: 哈希表,用哈希表记录每一个字符出现的位置,如果对应的字符出现的位置不一样,就返回False,到最后都一样就返回True。也可以只记录最后一个字符出现的位置,因为之前出现的位置已经比较过了。
Java:
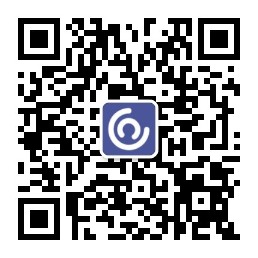
public class Solution { public boolean isIsomorphic(String s1, String s2) { int[] m = new int[512]; for (int i = 0; i < s1.length(); i++) { if (m[s1.charAt(i)] != m[s2.charAt(i)+256]) return false; m[s1.charAt(i)] = m[s2.charAt(i)+256] = i+1; } return true; } }
Python:
def isIsomorphic1(self, s, t): d1, d2 = {}, {} for i, val in enumerate(s): d1[val] = d1.get(val, []) + [i] for i, val in enumerate(t): d2[val] = d2.get(val, []) + [i] return sorted(d1.values()) == sorted(d2.values()) def isIsomorphic2(self, s, t): d1, d2 = [[] for _ in xrange(256)], [[] for _ in xrange(256)] for i, val in enumerate(s): d1[ord(val)].append(i) for i, val in enumerate(t): d2[ord(val)].append(i) return sorted(d1) == sorted(d2) def isIsomorphic3(self, s, t): return len(set(zip(s, t))) == len(set(s)) == len(set(t)) def isIsomorphic4(self, s, t): return [s.find(i) for i in s] == [t.find(j) for j in t] def isIsomorphic5(self, s, t): return map(s.find, s) == map(t.find, t) def isIsomorphic(self, s, t): d1, d2 = [0 for _ in xrange(256)], [0 for _ in xrange(256)] for i in xrange(len(s)): if d1[ord(s[i])] != d2[ord(t[i])]: return False d1[ord(s[i])] = i+1 d2[ord(t[i])] = i+1 return True
C++:
class Solution { public: bool isIsomorphic(string s, string t) { int m1[256] = {0}, m2[256] = {0}, n = s.size(); for (int i = 0; i < n; ++i) { if (m1[s[i]] != m2[t[i]]) return false; m1[s[i]] = i + 1; m2[t[i]] = i + 1; } return true; } };
ll LeetCode Questions List 题目汇总