【LeetCode】205. Isomorphic Strings 同构字符串(Easy)(JAVA)
题目地址: https://leetcode.com/problems/isomorphic-strings/
题目描述:
Given two strings s and t, determine if they are isomorphic.
Two strings are isomorphic if the characters in s can be replaced to get t.
All occurrences of a character must be replaced with another character while preserving the order of characters. No two characters may map to the same character but a character may map to itself.
Example 1:
Input: s = "egg", t = "add"
Output: true
Example 2:
Input: s = "foo", t = "bar"
Output: false
Example 3:
Input: s = "paper", t = "title"
Output: true
Note:
You may assume both s and t have the same length.
题目大意
给定两个字符串 s 和 t,判断它们是否是同构的。
如果 s 中的字符可以被替换得到 t ,那么这两个字符串是同构的。
所有出现的字符都必须用另一个字符替换,同时保留字符的顺序。两个字符不能映射到同一个字符上,但字符可以映射自己本身。
说明:
你可以假设 s 和 t 具有相同的长度。
解题方法
- 用一个 map 把已经有的映射记录下来
- 下一次要先判断 s.charAt(i) 是否和记录的映射相同, t.charAt(i) 是否已经在映射里了(因为不能多对一,也就是 s 中的 a,b 不能映射到 t 中的 a)
class Solution {
public boolean isIsomorphic(String s, String t) {
if (s.length() != t.length()) return false;
Map<Character, Character> map = new HashMap<>();
for (int i = 0; i < s.length(); i++) {
char tempS = s.charAt(i);
char tempT = t.charAt(i);
Character pre = map.get(tempS);
if (pre == null && map.containsValue(tempT)) return false;
if (pre != null && pre != tempT) return false;
map.put(tempS, tempT);
}
return true;
}
}
执行耗时:14 ms,击败了38.16% 的Java用户
内存消耗:38.7 MB,击败了62.40% 的Java用户
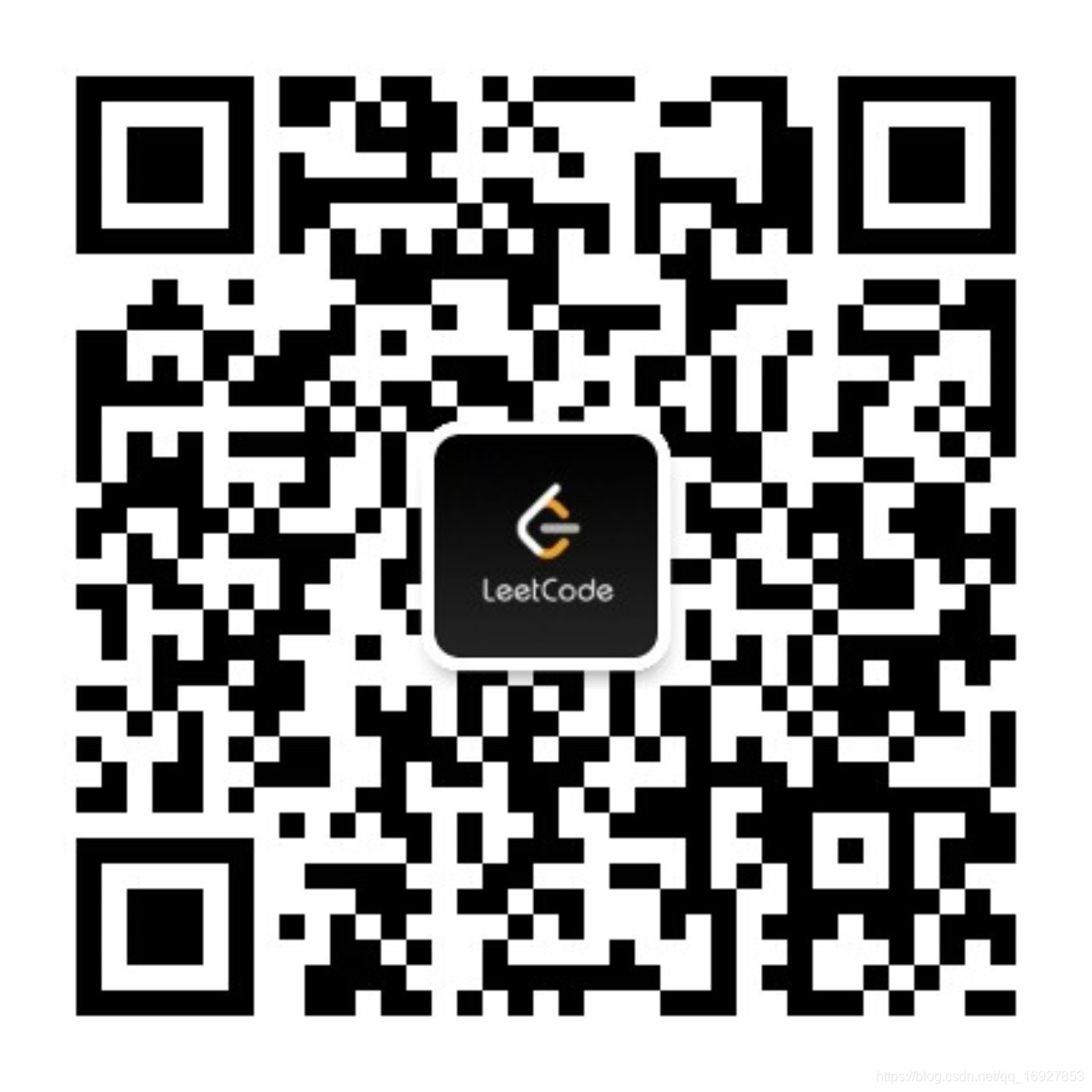