原生js日历
demo效果:
实现日历的思路:
1、利用new Date()获取今天日期
2、判断今年是平年还是闰年,确定今年每个月有多少天
3、确定今天日期所在月的第一天是星期几
4、计算出日历的行数
5、利用今天日期所在月的天数与该月第一天星期几来渲染日历表格
6、左右切换月份
源码:
- html
<div class="calendar-container">
<div class="calendar-header">
<div class="left btn"><</div>
<div class="year"></div>
<div class="right btn">></div>
</div>
<div class="calendar-body">
<div class="week-row">
<div class="week box">日</div>
<div class="week box">一</div>
<div class="week box">二</div>
<div class="week box">三</div>
<div class="week box">四</div>
<div class="week box">五</div>
<div class="week box">六</div>
</div>
<div class="day-rows">
<!--日期的渲染的地方-->
</div>
</div>
</div>
- css
.calendar-container{
width: calc(31px*7 + 1px);
}
.calendar-header{
display: flex;
justify-content: space-between;
}
.year{
text-align: center;
line-height: 30px;
}
.btn{
width: 30px;
height: 30px;
text-align: center;
line-height: 30px;
cursor: pointer;
}
.calendar-body{
border-right: 1px solid #9e9e9e;
border-bottom: 1px solid #9e9e9e;
}
.week-row, .day-rows, .day-row{
overflow: hidden;
}
.box{
float: left;
width: 30px;
height: 30px;
border-top: 1px solid #9e9e9e;
border-left: 1px solid #9e9e9e;
text-align: center;
line-height: 30px;
}
.week{
background: #00bcd4;
}
.day{
background: #ffeb3b;
}
.curday{
background: #ff5722;
}
- js
// 获取今天日期
let curTime = new Date(),
curYear = curTime.getFullYear(),
curMonth = curTime.getMonth(),
curDate = curTime.getDate();
console.log(curTime, curYear, curMonth, curDate)
// 判断平年还是闰年
function isLeapYear(year){
return (year%400 === 0) || ((year%4 === 0) && (year%100 !== 0))
}
function render(curYear, curMonth){
document.querySelector('.year').innerHTML = `${curYear}年${curMonth + 1}月`;
// 判断今年是平年还是闰年,并确定今年的每个月有多少天
let daysInMonth = [31, isLeapYear(curYear) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31];
// 确定今天日期所在月的第一天是星期几
let firstDayInMonth = new Date(curYear, curMonth, 1),
firstDayWeek = firstDayInMonth.getDay();
// 根据当前月的天数和当前月第一天星期几来确定当前月的行数
let calendarRows = Math.ceil((firstDayWeek + daysInMonth[curMonth])/7);
// 将每一行的日期放入到rows数组中
let rows = [];
// 外循环渲染日历的每一行
for(let i = 0; i < calendarRows; i++){
rows[i] = `<div class="day-row">`;
// 内循环渲染日历的每一天
for(let j = 0; j < 7; j++){
// 内外循环构成了一个calendarRows*7的表格,为当前月的每个表格设置idx索引;
// 利用idx索引与当前月第一天星期几来确定当前月的日期
let idx = i*7 + j,
date = idx - firstDayWeek + 1;
// 过滤掉无效日期、渲染有效日期
if(date <= 0 || date > daysInMonth[curMonth]){
rows[i] += `<div class="day box"></div>`
}else if(date === curDate){
rows[i] += `<div class="day box curday">${date}</div>`
}else{
rows[i] += `<div class="day box">${date}</div>`
}
}
rows[i] += `</div>`
}
let dateStr = rows.join('');
document.querySelector('.day-rows').innerHTML = dateStr;
}
// 首次调用render函数
render(curYear, curMonth);
let leftBtn = document.querySelector('.left'),
rightBtn = document.querySelector('.right');
// 向左切换月份
leftBtn.addEventListener('click', function(){
curMonth--;
if(curMonth < 0){
curYear -= 1;
curMonth = 11;
}
render(curYear, curMonth);
})
// 向右切换月份
rightBtn.addEventListener('click', function(){
curMonth++;
if(curMonth > 11){
curYear += 1;
curMonth = 0;
}
render(curYear, curMonth);
})
小结:
扫描二维码关注公众号,回复:
3237156 查看本文章
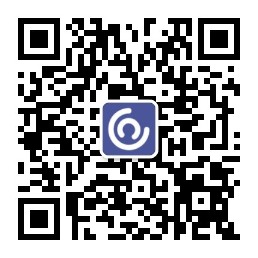
1、为了实现左右切换月份,将日历日期渲染代码放入到了render
函数,方便月份切换后重新渲染;
2、确定当前月的行数时,要结合当前月的天数与当前月第一天星期几来共同确定;
3、原生js日历中比较核心的就是如何确定每一天的日期,在这儿利用了内外循环,内外循环构成了一个calendarRows*7的表格,为当前月的每个表格设置idx索引;利用idx索引与当前月第一天星期几来确定当前月的日期;记得要过滤掉无效日期!!!
参考文献:
[1] js实现日历的简单算法
[2] 创建一个js日历(原生JS实现日历)