1、web.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
3 <display-name>springmvc1</display-name>
4
5 <filter>
6 <filter-name>characterEncoding</filter-name>
7 <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
8 <init-param>
9 <param-name>encoding</param-name>
10 <param-value>UTF-8</param-value>
11 </init-param>
12 </filter>
13 <filter-mapping>
14 <filter-name>characterEncoding</filter-name>
15 <url-pattern>/*</url-pattern>
16 </filter-mapping>
17
18 <servlet>
19 <servlet-name>springmvc</servlet-name>
20 <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
21 <init-param>
22 <param-name>contextConfigLocation</param-name>
23 <param-value>classpath:springmvc.xml</param-value>
24 </init-param>
25 </servlet>
26 <servlet-mapping>
27 <servlet-name>springmvc</servlet-name>
28 <url-pattern>*.do</url-pattern>
29 </servlet-mapping>
30
31 <welcome-file-list>
32 <welcome-file>index.html</welcome-file>
33 <welcome-file>index.htm</welcome-file>
34 <welcome-file>index.jsp</welcome-file>
35 <welcome-file>default.html</welcome-file>
36 <welcome-file>default.htm</welcome-file>
37 <welcome-file>default.jsp</welcome-file>
38 </welcome-file-list>
39
40 </web-app>
2、springmvc.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <beans xmlns="http://www.springframework.org/schema/beans"
3 xmlns:mvc="http://www.springframework.org/schema/mvc"
4 xmlns:aop="http://www.springframework.org/schema/aop"
5 xmlns:tx="http://www.springframework.org/schema/tx"
6 xmlns:context="http://www.springframework.org/schema/context"
7 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
8 xsi:schemaLocation="http://www.springframework.org/schema/beans
9 http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
10 http://www.springframework.org/schema/mvc
11 http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
12 http://www.springframework.org/schema/context
13 http://www.springframework.org/schema/context/spring-context-3.2.xsd
14 http://www.springframework.org/schema/aop
15 http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
16 http://www.springframework.org/schema/tx
17 http://www.springframework.org/schema/tx/spring-tx-3.2.xsd">
18
19 <!-- 把Controller交给spring管理 -->
20 <context:component-scan base-package="com.xiaostudy"/>
21
22 <!-- 配置注解处理器映射器 功能:寻找执行类Controller -->
23 <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
24
25 <!-- 配置注解处理器适配器 功能:调用controller方法,执行controller -->
26 <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter"/>
27
28 <!-- 配置sprigmvc视图解析器:解析逻辑试图
29 后台返回逻辑试图:index
30 视图解析器解析出真正物理视图:前缀+逻辑试图+后缀====/WEB-INF/index.jsp -->
31 <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
32 <property name="prefix" value="/WEB-INF/"/>
33 <property name="suffix" value=".jsp"/>
34 </bean>
35 </beans>
3、用注解@Controller类
1 package com.xiaostudy.controller;
2
3 import org.springframework.stereotype.Controller;
4 import org.springframework.web.bind.annotation.RequestMapping;
5 import org.springframework.web.bind.annotation.RequestMethod;
6
7 @Controller//<bean class="com.xiaostudy.controller.MyController"/>
8 @RequestMapping(value="/myController")//访问该类的方法时,前面多这样一个路径
9 public class MyController {
10
11 // @RequestMapping("hello")//http://localhost:8080/demo2/hello.do
12 // @RequestMapping("/hello")//http://localhost:8080/demo2/hello.do
13 // @RequestMapping(value="/hello.do")//http://localhost:8080/demo2/hello.do
14 // @RequestMapping(value="/hello.do",method=RequestMethod.GET)//http://localhost:8080/demo2/hello.do
15 // @RequestMapping(value="/hello.do",method= {RequestMethod.GET,RequestMethod.POST})//http://localhost:8080/demo2/hello.do
16 public String print() {
17 return "index";
18 }
19
20 @RequestMapping("hi")//http://localhost:8080/demo2/myController/hi.do
21 public String hello() {
22 return "index";
23 }
24
25 }
4、index.jsp
1 <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
2 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
3 "http://www.w3.org/TR/html4/loose.dtd">
4 <html>
5 <head>
6 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
7 <title>springMVC_demo</title>
8 </head>
9 <body>
10 xiaostudy
11 </body>
12 </html>
项目文件结构
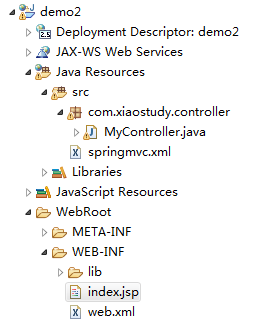