版权声明:本文原创如果喜欢,欢迎转载。^_^ https://blog.csdn.net/ccutyear/article/details/77206192
Transformation
Time Limit: 15000/8000 MS (Java/Others) Memory Limit: 65535/65536 K (Java/Others)Total Submission(s): 5865 Accepted Submission(s): 1459
Problem Description
Yuanfang is puzzled with the question below:
There are n integers, a 1, a 2, …, a n. The initial values of them are 0. There are four kinds of operations.
Operation 1: Add c to each number between a x and a y inclusive. In other words, do transformation a k<---a k+c, k = x,x+1,…,y.
Operation 2: Multiply c to each number between a x and a y inclusive. In other words, do transformation a k<---a k×c, k = x,x+1,…,y.
Operation 3: Change the numbers between a x and a y to c, inclusive. In other words, do transformation a k<---c, k = x,x+1,…,y.
Operation 4: Get the sum of p power among the numbers between a x and a y inclusive. In other words, get the result of a x p+a x+1 p+…+a y p.
Yuanfang has no idea of how to do it. So he wants to ask you to help him.
There are n integers, a 1, a 2, …, a n. The initial values of them are 0. There are four kinds of operations.
Operation 1: Add c to each number between a x and a y inclusive. In other words, do transformation a k<---a k+c, k = x,x+1,…,y.
Operation 2: Multiply c to each number between a x and a y inclusive. In other words, do transformation a k<---a k×c, k = x,x+1,…,y.
Operation 3: Change the numbers between a x and a y to c, inclusive. In other words, do transformation a k<---c, k = x,x+1,…,y.
Operation 4: Get the sum of p power among the numbers between a x and a y inclusive. In other words, get the result of a x p+a x+1 p+…+a y p.
Yuanfang has no idea of how to do it. So he wants to ask you to help him.
Input
There are no more than 10 test cases.
For each case, the first line contains two numbers n and m, meaning that there are n integers and m operations. 1 <= n, m <= 100,000.
Each the following m lines contains an operation. Operation 1 to 3 is in this format: "1 x y c" or "2 x y c" or "3 x y c". Operation 4 is in this format: "4 x y p". (1 <= x <= y <= n, 1 <= c <= 10,000, 1 <= p <= 3)
The input ends with 0 0.
For each case, the first line contains two numbers n and m, meaning that there are n integers and m operations. 1 <= n, m <= 100,000.
Each the following m lines contains an operation. Operation 1 to 3 is in this format: "1 x y c" or "2 x y c" or "3 x y c". Operation 4 is in this format: "4 x y p". (1 <= x <= y <= n, 1 <= c <= 10,000, 1 <= p <= 3)
The input ends with 0 0.
Output
For each operation 4, output a single integer in one line representing the result. The answer may be quite large. You just need to calculate the remainder of the answer when divided by 10007.
Sample Input
5 5 3 3 5 7 1 2 4 4 4 1 5 2 2 2 5 8 4 3 5 3 0 0
Sample Output
307 7489
思路:线段树经典题,需要想到的是对于相加时:sum^3 = sum^3 + 3*sum^2*add_num + 3*sum*add_num^2 + (right - left + 1)*add_num^3,sum^2 = sum^2 + 2*sum*add_num + (right - left + 1)*add_num^2,sum[0] = sum[0] + (right - left + 1)*add_num。对于相乘时:sum = sum[0]*mul_num,sum^2 = sum^2*mul_num^2,sum^3 = sum^3*mul_num^3。同时还需要清楚:变化会影响相乘和相加,相乘会影响相加不会影响变化,相加不会影响相乘和变化。
AC代码:
#include<iostream>
#include<cstring>
#include<cstdio>
#include<cmath>
#include<algorithm>
#define MAX 100005
#define MOD 10007
#define ll long long
using namespace std;
struct Node{
ll left,right;
ll add,mul,constant;
ll sum[3];
}node[MAX*3];
ll n,m;
void UpdateNodeChange(ll pos,ll change_num){
node[pos].add = 0,node[pos].mul = 1,node[pos].constant = change_num;
node[pos].sum[0] = ((node[pos].right - node[pos].left + 1)*change_num)%MOD;
node[pos].sum[1] = ((node[pos].right - node[pos].left + 1)*change_num*change_num)%MOD;
node[pos].sum[2] = ((node[pos].right - node[pos].left + 1)*change_num*change_num*change_num)%MOD;
return ;
}
void UpdateNodeMul(ll pos,ll mul_num){
node[pos].add = (node[pos].add*mul_num)%MOD;
node[pos].mul = (node[pos].mul*mul_num)%MOD;
node[pos].sum[0] = (node[pos].sum[0]*mul_num)%MOD;
node[pos].sum[1] = (node[pos].sum[1]*mul_num*mul_num)%MOD;
node[pos].sum[2] = (node[pos].sum[2]*mul_num*mul_num*mul_num)%MOD;
return ;
}
void UpdateNodeAdd(ll pos,ll add_num){
node[pos].add = (node[pos].add + add_num)%MOD;
node[pos].sum[2] = (node[pos].sum[2] + 3*node[pos].sum[1]*add_num + 3*node[pos].sum[0]*add_num*add_num + (node[pos].right - node[pos].left + 1)*add_num*add_num*add_num)%MOD;
node[pos].sum[1] = (node[pos].sum[1] + 2*node[pos].sum[0]*add_num + (node[pos].right - node[pos].left + 1)*add_num*add_num)%MOD;
node[pos].sum[0] = (node[pos].sum[0] + (node[pos].right - node[pos].left + 1)*add_num)%MOD;
return ;
}
void UpdateNode(ll pos,ll add_num,ll mul_num,ll change_num)
{//在此处更新时因为change会影响mul和add,mul不会影响change会影响add,add不会影响change和mul
//所以更新的顺序必须是change -> mul -> add.
if(change_num != 0)
UpdateNodeChange(pos,change_num);
UpdateNodeMul(pos,mul_num);
UpdateNodeAdd(pos,add_num);
return ;
}
void PushDown(ll pos)
{
UpdateNode(pos<<1,node[pos].add,node[pos].mul,node[pos].constant);
UpdateNode(pos<<1|1,node[pos].add,node[pos].mul,node[pos].constant);
node[pos].add = 0,node[pos].mul = 1,node[pos].constant = 0;
return ;
}
void PushUp(ll pos)
{
for(ll j = 0; j < 3; j++)
node[pos].sum[j] = (node[pos<<1].sum[j] + node[pos<<1|1].sum[j])%MOD;
}
void BuildTree(ll now_left,ll now_right,ll pos)
{
node[pos]= (Node){now_left,now_right,0,1,0,{0,0,0}};
if(now_left == now_right) return ;
BuildTree(now_left,(now_right + now_left)>>1,pos<<1);
BuildTree(((now_left + now_right)>>1) + 1,now_right,pos<<1|1);
}
void AddTree(ll target_left,ll target_right,ll add_num,ll pos)
{
if(node[pos].left > target_right || node[pos].right < target_left) return ;
if(node[pos].left >= target_left&& node[pos].right <= target_right){
UpdateNodeAdd(pos,add_num);
return ;
}
PushDown(pos);
AddTree(target_left,target_right,add_num,pos<<1);
AddTree(target_left,target_right,add_num,pos<<1|1);
PushUp(pos);
}
void MulTree(ll target_left,ll target_right,ll mul_num,ll pos)
{
if(node[pos].left > target_right || node[pos].right < target_left) return ;
if(node[pos].left >= target_left && node[pos].right <= target_right){
UpdateNodeMul(pos,mul_num);
return;
}
PushDown(pos);
MulTree(target_left,target_right,mul_num,pos<<1);
MulTree(target_left,target_right,mul_num,pos<<1|1);
PushUp(pos);
}
void ChangeTree(ll target_left,ll target_right,ll change_num,ll pos)
{
if(node[pos].left > target_right || node[pos].right < target_left) return ;
if(node[pos].left >= target_left && node[pos].right <= target_right){
UpdateNodeChange(pos,change_num);
return ;
}
PushDown(pos);
ChangeTree(target_left,target_right,change_num,pos<<1);
ChangeTree(target_left,target_right,change_num,pos<<1|1);
PushUp(pos);
}
ll QueryTree(ll target_left,ll target_right,ll query_num,ll pos)
{
if(node[pos].left > target_right || node[pos].right < target_left) return 0;
if(node[pos].left >= target_left && node[pos].right <= target_right) return node[pos].sum[query_num];
PushDown(pos);
return (QueryTree(target_left,target_right,query_num,pos<<1) + QueryTree(target_left,target_right,query_num,pos<<1|1))%MOD;
}
int main( )
{
while(scanf("%lld%lld",&n,&m) != EOF){
if(n == 0 && m == 0) break;
BuildTree(1,n,1);
while(m--){
ll o,l,r,c;
scanf("%lld%lld%lld%lld",&o,&l,&r,&c);
switch(o){
case 1: AddTree(l,r,c,1);break;
case 2: MulTree(l,r,c,1);break;
case 3: ChangeTree(l,r,c,1);break;
case 4: c--;printf("%lld\n",QueryTree(l,r,c,1));
}
}
}
return 0;
}
本来应该继续补题的,但是,因为补起来有些吃力。所以,就把以前的题又拉出来深夜鞭尸了。^-^11
扫描二维码关注公众号,回复:
3049407 查看本文章
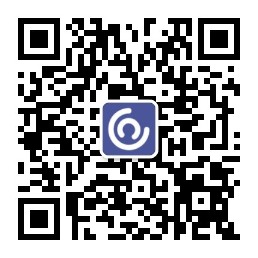