1、多种遍历
代码:
//多种遍历3
#include<cstdlib>//动态分配内存
#include<iostream>
#include<stdlib.h>
#include<algorithm>
using namespace std;
//建立节点
struct NODE
{
int value;
NODE*left;
NODE*right;
NODE(int v,NODE *l,NODE *r):value(v),left(NULL),right(NULL)
{
left=l;
right=r;
}
} ;
//生成随机函数
int randint(int lower,int upper)
{
return rand()%(upper-lower+1)+lower;
}
//rand函数 生成随机树
NODE*generateRandomBST(int lower,int upper)
{
NODE*t=nullptr;
int randomValue;
if(lower<=upper)
{
randomValue=randint(lower,upper);
t=new NODE(randomValue,generateRandomBST(lower,randomValue-1),generateRandomBST(randomValue+1,upper));
}
return t;
}
//1为下限,n为上限
NODE *generateRandomBST(int n)
{
return generateRandomBST(1, n);
}
//前序遍历
void preOrder(NODE*root)
{
if(root!=NULL)
{
cout<<root->value<<" ";
preOrder(root->left);
preOrder(root->right);
}
}
//中序遍历
void inOrder(NODE*root)
{
if(root!=NULL)
{
inOrder(root->left);
cout<<root->value<<" ";
inOrder(root->right);
}
}
//后序遍历
void postOrder(NODE*root)
{
if(root!=NULL)
{
postOrder(root->left);
postOrder(root->right);
cout<<root->value<<" ";
}
}
//层次遍历
void levelOrder(NODE*t)
{
int front=0,rear=1;
NODE *q[1000];
q[0]=t;
while(front<rear)
{
if(q[front])
{
cout<<q[front]->value<<" ";
q[rear++]=q[front]->left;
q[rear++]=q[front]->right;
front++;
}
else
{
front++;
}
}
}
int main()
{
NODE*root;
root=generateRandomBST(5,300);
cout<<"the pre Order result is:"<<endl;
preOrder(root);
cout<<endl;
cout<<"the in Order result is:"<<endl;
inOrder(root);
cout<<endl;
cout<<"the post Order result is:"<<endl;
postOrder(root);
cout<<endl;
cout<<"the level Order result is:"<<endl;
levelOrder(root);
cout<<endl;
return 0;
}
运行:
2、访问伸展树
题目:
答案:
原理:
当我们沿着树向下搜索某个节点X的时候,我们将搜索路径上的节点及其子树移走。我们构建两棵临时的树──左树和右树。
扫描二维码关注公众号,回复:
2748775 查看本文章
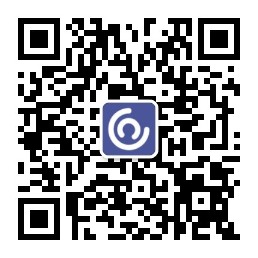
没有被移走的节点构成的树称作中树。在伸展操作的过程中:
1、当前节点X是中树的根。
2、左树L保存小于X的节点。
3、右树R保存大于X的节点。
开始时候,X是树T的根,左右树L和R都是空的。
例子图解(查找2结点的实例,来图解SplayTree的伸展过程):