//用到的权限
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.INTERNET" />//用到的依赖
useLibrary 'org.apache.http.legacy'
implementation 'com.google.guava:guava:16.0.1' implementation 'com.google.code.gson:gson:2.2.4'
//mainactivity
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
private RadioGroup group;
private List<Fragment> fList;
private ListView lv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.viewP);
group = findViewById(R.id.group);
fList = new ArrayList<>();
fList.add(new FragmentOne());
fList.add(new FragmentTwo());
viewPager.setAdapter(new FragmentPagerAdapter(getSupportFragmentManager()) {
@Override
public Fragment getItem(int position) {
return fList.get(position);
}
@Override
public int getCount() {
return fList.size();
}
});
// 小圆点
viewPager.setOnPageChangeListener(new ViewPager.OnPageChangeListener() {
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
}
@Override
public void onPageSelected(int position) {
switch (position) {
case 0:
group.check(R.id.but1);
break;
case 1:
group.check(R.id.but2);
break;
default:
break;
}
}
@Override
public void onPageScrollStateChanged(int state) {
}
});
group.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
switch (checkedId){
case R.id.but1:
viewPager.setCurrentItem(0);
break;
case R.id.but2:
viewPager.setCurrentItem(1);
break;
default:
break;
}
}
});
}
}
//fragmentOne
public class FragmentOne extends Fragment {
public static final String TAG = FragmentOne.class.getSimpleName();
private View view;
private ListView lv;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
//解决与系统view的冲突
if(view == null){
view = inflater.inflate(R.layout.one,container,false);
}
ViewGroup parent = (ViewGroup)view.getParent();
if(parent != null){
parent.removeView(view);
}
//资源ID的获取
lv = view.findViewById(R.id.lv);
//当点击子条目时,跳转到webActivity
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
NewsBean.DataBean.ToutiaoBean newsBean = (NewsBean.DataBean.ToutiaoBean) parent.getAdapter().getItem(position);
//进行跳转
Intent intent = new Intent(getActivity(), WebActivity.class);
//将数据传入web并且展示
intent.putExtra("url",newsBean.getLink());
//启动跳转
startActivity(intent);
}
});
new HttpURLConnectionGetDataTask().execute("https://www.apiopen.top/journalismApi");//地址
return view;
}
//使用 HttpURLConnectionGetDataTask 的 AsyncTask 做
class HttpURLConnectionGetDataTask extends AsyncTask<String, Void, String>{
@Override
protected String doInBackground(String... strings) {
try {
URL url = new URL(strings[0]);//地址的调用
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setRequestMethod("GET");//读取的方式
urlConnection.setConnectTimeout(3000);//响应时间
urlConnection.setReadTimeout(3000);//读取时间
//判断状态码
if (urlConnection.getResponseCode() == 200){
return CharStreams.toString(new InputStreamReader(urlConnection.getInputStream(), "UTF-8"));
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(String s) {
Log.i(TAG,"ssssssss" + s);
Gson gson = new Gson();//解析
NewsBean newsBean = gson.fromJson(s, NewsBean.class);
lv.setAdapter(new UrlAdapter(getActivity(), newsBean.getData().getToutiao()));
}
}
}
//fragmetTwo使用的是httpclient
public class FragmentTwo extends Fragment {
public static final String TAG = FragmentOne.class.getSimpleName();
private View view;
private ListView listIew;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
if(view == null){
view = inflater.inflate(R.layout.two,container,false);
}
ViewGroup parent = (ViewGroup)view.getParent();
if(parent != null){
parent.removeView(view);
}
listIew = view.findViewById(R.id.lvs);
listIew.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
NewsBean.DataBean.ToutiaoBean newsBean = (NewsBean.DataBean.ToutiaoBean) parent.getAdapter().getItem(position);
Intent intent = new Intent(getActivity(), WebActivity.class);
intent.putExtra("url",newsBean.getLink());
Toast.makeText(getActivity(),"ssssssss",Toast.LENGTH_SHORT).show();
startActivity(intent);
}
});
new HttpClientGetDataTask().execute("https://www.apiopen.top/journalismApi");
return view;
}
//使用AsyncTask
private class HttpClientGetDataTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... strings) {
HttpClient client = new DefaultHttpClient();
HttpGet get = new HttpGet(strings[0]);
try {
HttpResponse response = client.execute(get);
//判断状态码
if (response.getStatusLine().getStatusCode() == 200){
return EntityUtils.toString(response.getEntity());
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(String s) {
Gson gson = new Gson();//解析
NewsBean newsBean = gson.fromJson(s,NewsBean.class);
listIew.setAdapter(new UrlAdapter(getActivity(),newsBean.getData().getToutiao()));
}
}
}
//WebActivity
public class WebActivity extends AppCompatActivity {
private WebView webView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web);
webView = findViewById(R.id.webview);//获取资源ID
webView.setWebViewClient(new WebViewClient(){
@Override
public void onPageStarted(WebView view, String url, Bitmap favicon) {
super.onPageStarted(view, url, favicon);
}
@Override
public void onPageFinished(WebView view, String url) {
super.onPageFinished(view, url);
}
@Override
public void onPageCommitVisible(WebView view, String url) {
super.onPageCommitVisible(view, url);
}
});
webView.loadUrl(getIntent().getStringExtra("url"));//得到地址里面的链接
}
}
//Adapter
public class UrlAdapter extends BaseAdapter {
private Context context;
private List<NewsBean.DataBean.ToutiaoBean> list;
public UrlAdapter(Context context, List<NewsBean.DataBean.ToutiaoBean> list) {
this.context = context;
this.list = list;
}
@Override
public int getCount() {
return list.size();
}
@Override
public Object getItem(int position) {
return list.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null){
holder = new ViewHolder();
convertView = View.inflate(context, R.layout.url_item,null);
holder.textView = convertView.findViewById(R.id.text_1);
holder.textView2 = convertView.findViewById(R.id.text_2);
convertView.setTag(holder);
}else {
holder = (ViewHolder) convertView.getTag();
}
holder.textView.setText(list.get(position).getTitle());
holder.textView2.setText(list.get(position).getType());
return convertView;
}
class ViewHolder{
TextView textView,textView2;
}
}
//main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.bwie.wangbingjun.MainActivity">
<android.support.v4.view.ViewPager
android:id="@+id/viewP"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="9"
>
</android.support.v4.view.ViewPager>
<RadioGroup
android:id="@+id/group"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
>
<RadioButton
android:id="@+id/but1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:button="@null"
android:checked="true"
android:padding="8dp"
android:text="HttpURLConnection"
/>
<RadioButton
android:id="@+id/but2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:button="@null"
android:padding="8dp"
android:text="HttpClient"
/>
</RadioGroup>
</LinearLayout>
//web.xml
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.bwie.wangbingjun.WebActivity">
<WebView
android:id="@+id/webview"
android:layout_width="match_parent"
android:layout_height="match_parent">
</WebView>
</android.support.constraint.ConstraintLayout>
//one
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#cee"
>
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="wrap_content"></ListView>
</LinearLayout>
扫描二维码关注公众号,回复:
2694004 查看本文章
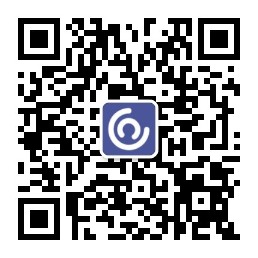
//two
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorPrimaryDark"
>
<ListView
android:id="@+id/lvs"
android:layout_width="match_parent"
android:layout_height="wrap_content"></ListView>
</LinearLayout>
//item
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="24dp"
android:layout_marginStart="24dp"
android:layout_marginTop="33dp"
android:text="TextView" />
<TextView
android:id="@+id/text_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignEnd="@id/text_1"
android:layout_alignRight="@id/text_1"
android:layout_below="@id/text_1"
android:layout_marginTop="40dp"
android:text="TextView" />
</RelativeLayout>