Find the result of the following code:
long long pairsFormLCM( int n ) {
long long res = 0;
for( int i = 1; i <= n; i++ )
for( int j = i; j <= n; j++ )
if( lcm(i, j) == n ) res++; // lcm means least common multiple
return res;
}
A straight forward implementation of the code may time out. If you analyze the code, you will find that the code actually counts the number of pairs (i, j) for which lcm(i, j) = n and (i ≤ j).
Input
Input starts with an integer T (≤ 200), denoting the number of test cases.
Each case starts with a line containing an integer n (1 ≤ n ≤ 1e14).
Output
For each case, print the case number and the value returned by the function ‘pairsFormLCM(n)’.
Sample Input
15
2
3
4
6
8
10
12
15
18
20
21
24
25
27
29
Sample Output
Case 1: 2
Case 2: 2
Case 3: 3
Case 4: 5
Case 5: 4
Case 6: 5
Case 7: 8
Case 8: 5
Case 9: 8
Case 10: 8
Case 11: 5
Case 12: 11
Case 13: 3
Case 14: 4
Case 15: 2
题意: 给你一个正整数n,让你求满足LCM(i,j) = n 的数对(i,j)有多少个
新知: 暴力不可解,然后做这个题时想了各种思路,都感觉时间复杂度爆炸。肝了一个多小时后选择放弃QAQ
然后一查题解发现了自己数论知识的盲区……
就是质因数分解和LCM和GCD之间有联系,自己发现一篇讲解不错,可以查看
https://www.cnblogs.com/linliu/p/5549544.html
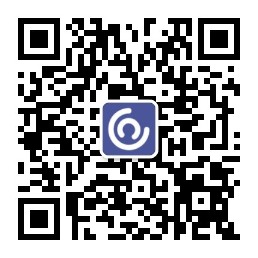
AC代码:
#include<bits/stdc++.h>
using namespace std;
typedef long long LL;
const int maxn = 1e7+1000;
const int MAXN = 1e3+5;
bool p[maxn];
int prime[maxn/10];
int a[MAXN];
int b[MAXN];
int tot;
int num;
void Init()
{
tot = 0;
memset(p,true,sizeof(p));
p[0] = p[1] = false;
for(LL i=2;i<maxn;i++)
{
if(p[i]){
prime[tot++] = i;
for(LL j=i*i;j<maxn;j+=i) p[j] = false;
}
}
}
void dec(LL x)
{
num = 0;
memset(a,0,sizeof(a));
memset(b,0,sizeof(b));
for(int i=0;1LL*prime[i]*prime[i]<=x;i++)
{
if(x%prime[i] == 0){
a[num] = prime[i];
while(x%prime[i] == 0)
{
b[num]++;
x/=prime[i];
}
num++;
}
}
if(x!=1){
a[num] = x;
b[num] = 1;
num++;
}
}
int main()
{
#ifdef LOCAL_FILE
freopen("in.txt","r",stdin);
#endif
// ios_base::sync_with_stdio(false);
// cin.tie(NULL),cout.tie(NULL);
int t;
int id = 1;
scanf("%d",&t);
Init();
while(t--)
{
LL n;
scanf("%lld",&n);
dec(n);
LL ans = 1;
for(int i=0;i<num;i++)
ans*=(2*b[i]+1);
ans = ans/2+1;
printf("Case %d: %lld\n",id++,ans);
}
return 0;
}