1、网络权限
<uses-permission android:name="android.permission.INTERNET" />
2、动画
Main XML
<LinearLayout android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <!--FrameLayout包裹按钮--> <FrameLayout android:id="@+id/frament" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"> </FrameLayout><!--FrameLayout包裹按钮--> <RadioGroup android:id="@+id/redo" android:layout_width="match_parent" android:layout_height="54dp" android:layout_gravity="bottom" android:orientation="horizontal"> <RadioButton android:id="@+id/lie_biao" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:button="@null" android:gravity="center" android:text="列表" /> <RadioButton android:id="@+id/xiang_qing" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:button="@null" android:gravity="center" android:text="详情" /> </RadioGroup> </LinearLayout>
3、Main点击切换页面
public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private FragmentManager supportFragmentManager; //执行者调全局 private FragmentTransaction fragmentTransaction; //调全局FrameLayout控件 public FrameLayout frament; private AFragment aFragment; private BFragment bFragment; private RadioButton lie_biao; private RadioButton xiang_qing; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);//注意改变Main入口 initView(); initData(); } private void initData() { aFragment = new AFragment(); bFragment = new BFragment(); supportFragmentManager = getSupportFragmentManager(); fragmentTransaction = supportFragmentManager.beginTransaction(); fragmentTransaction.add(R.id.frament,aFragment); fragmentTransaction.commit(); } private void initView() { lie_biao = (RadioButton) findViewById(R.id.lie_biao); xiang_qing = (RadioButton) findViewById(R.id.xiang_qing); lie_biao.setOnClickListener(this); xiang_qing.setOnClickListener(this); frament = (FrameLayout) findViewById(R.id.frament); frament.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.lie_biao: //管理者 执行者 //替换 //提交 getSupportFragmentManager().beginTransaction().replace(R.id.frament,aFragment).commit(); break; case R.id.xiang_qing: //管理者 执行者 //替换 //提交 getSupportFragmentManager().beginTransaction().replace(R.id.frament,bFragment).commit(); break; } }
}
4、AFragment页面
public class AFragment extends Fragment { private Handler handler = new Handler() { @Override public void handleMessage(Message msg) { super.handleMessage(msg); String json = (String) msg.obj; MyBean myBean = new Gson().fromJson(json, MyBean.class); student = myBean.getStudents().getStudent(); recy.setLayoutManager(new LinearLayoutManager(getContext())); myAdapter = new MyAdapter(AFragment.this.student, getContext()); recy.setAdapter(myAdapter); myAdapter.setOnCliK(new MyAdapter.OnClik() { @Override public void OnCliklistener(int position) { //在AFragment中适配器点击事件中,调用BFragment的集合进行添加我们电机的图片的url地址 BFragment.list.add(student.get(position).getImg()); } }); } }; private RecyclerView recy; public static MyAdapter myAdapter; public static List<MyBean.StudentsBean.StudentBean> student; public AFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment View inflate = inflater.inflate(R.layout.fragment_a, container, false); initView(inflate); initData(); return inflate; } private void initData() { OkHttpClient build = new OkHttpClient.Builder().build(); Request request = new Request.Builder().url("http://192.168.43.191:8080/data.json").build(); build.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { } @Override public void onResponse(Call call, Response response) throws IOException { String string = response.body().string(); handler.obtainMessage(1, string).sendToTarget(); } }); } private void initView(View inflate) { recy = (RecyclerView) inflate.findViewById(R.id.recy); } }
5、Bfragment页面
public class BFragment extends Fragment { private MyBean.StudentsBean.StudentBean bean; private ImageView img; private String imgV; public static ArrayList<String> list = new ArrayList<>(); private RecyclerView recy; private MyBAdapter myBAdapter; // private // private ImageView img; public BFragment() { // Required empty public constructor } //在我们的Activity获取到焦点的时候我们进行适配器刷新 @Override public void onResume() { super.onResume(); myBAdapter.notifyDataSetChanged(); } public static int tag; @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment View view = inflater.inflate(R.layout.fragment_b, container, false); initView(view); myBAdapter = new MyBAdapter(list, getActivity()); recy.setLayoutManager(new GridLayoutManager(getContext(),2)); recy.setAdapter(myBAdapter); myBAdapter.setOnCilckListener(new MyBAdapter.onCilckListener() { @Override public void onClick(int position) { Intent intent = new Intent(getContext(), Main2Activity.class); tag = position; startActivity(intent); } }); return view; } private void initView(View view) { img = (ImageView) view.findViewById(R.id.img); recy = (RecyclerView) view.findViewById(R.id.recy); } }
6、A适配器
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.Holder> { private List<MyBean.StudentsBean.StudentBean> student; private Context mc; private OnClik onClik; public MyAdapter(List<MyBean.StudentsBean.StudentBean> student, Context mc) { this.student = student; this.mc = mc; } @NonNull @Override public MyAdapter.Holder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View inflate = LayoutInflater.from(mc).inflate(R.layout.itema, parent, false); return new Holder(inflate); } @Override public void onBindViewHolder(@NonNull MyAdapter.Holder holder, final int position) { holder.name.setText(student.get(position).getName()); holder.conten.setText(student.get(position).getContent()); Picasso.with(mc).load(student.get(position).getImg()).into(holder.img); holder.itemView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { onClik.OnCliklistener(position); } }); } @Override public int getItemCount() { return student.size(); } public class Holder extends RecyclerView.ViewHolder { private final ImageView img; private final TextView name; private final TextView conten; public Holder(View itemView) { super(itemView); img = itemView.findViewById(R.id.img); name = itemView.findViewById(R.id.name); conten = itemView.findViewById(R.id.conten); } } public interface OnClik{ void OnCliklistener (int position); } public void setOnCliK(OnClik onClik){ this.onClik=onClik; } }
7、B适配器
扫描二维码关注公众号,回复:
2485815 查看本文章
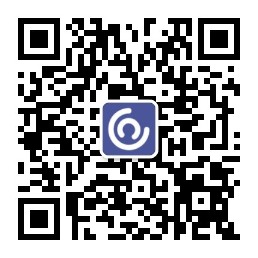
public class MyBAdapter extends RecyclerView.Adapter<MyBAdapter.Holder> { private ArrayList<String> list; private Context mc; onCilckListener onCilckListener; public void setOnCilckListener(MyBAdapter.onCilckListener onCilckListener) { this.onCilckListener = onCilckListener; } public interface onCilckListener{ void onClick(int position); } public MyBAdapter(ArrayList<String> list, Context mc) { this.list = list; this.mc = mc; } @NonNull @Override public MyBAdapter.Holder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View inflate = LayoutInflater.from(mc).inflate(R.layout.itemb, parent, false); return new Holder(inflate); } @Override public void onBindViewHolder(@NonNull MyBAdapter.Holder holder, final int position) { Picasso.with(mc).load(list.get(position)).into(holder.img); holder.img.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { onCilckListener.onClick(position); } }); } @Override public int getItemCount() { return list==null?0:list.size(); } public class Holder extends RecyclerView.ViewHolder { private final ImageView img; public Holder(View itemView) { super(itemView); img = itemView.findViewById(R.id.img); } } }
8、b——item XML
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <ImageView android:id="@+id/img" android:layout_width="match_parent" android:layout_height="100dp" android:src="@mipmap/ic_launcher" /> </LinearLayout>
9、a_item XML;
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <ImageView android:id="@+id/img" android:layout_width="100dp" android:layout_height="100dp" android:src="@mipmap/ic_launcher" /> <LinearLayout android:orientation="vertical" android:layout_width="match_parent" android:layout_height="100dp"> <TextView android:id="@+id/name" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="hhh" /> <TextView android:layout_marginTop="20dp" android:id="@+id/conten" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="hhh" /> </LinearLayout> </LinearLayout>
A Fragment XML
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".AFragment"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="首页" android:gravity="center" android:textSize="25sp"/> <android.support.v7.widget.RecyclerView android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/recy"/> </LinearLayout>
BFragment XML
<LinearLayout android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".BFragment"> <android.support.v7.widget.RecyclerView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/recy" ></android.support.v7.widget.RecyclerView> </LinearLayout>
轮播图XML
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".Main2Activity"> <android.support.v4.view.ViewPager android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/vp" ></android.support.v4.view.ViewPager> </android.support.constraint.ConstraintLayout>
轮播图Java代码
public class Main2Activity extends AppCompatActivity { public static ViewPager vp; private ArrayList<ImageView> imageViews; private PagerAdapter pagerAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main2); initView(); ArrayList<String> list = BFragment.list; imageViews = new ArrayList<>(); for (int i = 0; i < list.size(); i++) { ImageView imageView = new ImageView(this); Picasso.with(this).load(list.get(i)).into(imageView); imageViews.add(imageView); } pagerAdapter = new PagerAdapter() { @Override public int getCount() { return imageViews.size(); } @Override public boolean isViewFromObject(@NonNull View view, @NonNull Object object) { return view == object; } @NonNull @Override public Object instantiateItem(@NonNull ViewGroup container, int position) { container.addView(imageViews.get(position)); return imageViews.get(position); } @Override public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) { container.removeView(imageViews.get(position)); } }; vp.setAdapter(pagerAdapter); } @Override protected void onResume() { super.onResume(); vp.setCurrentItem(BFragment.tag); pagerAdapter.notifyDataSetChanged(); } private void initView() { vp = (ViewPager) findViewById(R.id.vp); } }