问题:
George took sticks of the same length and cut them randomly until all parts became at most 50 units long. Now he wants to return sticks to the original state, but he forgot how many sticks he had originally and how long they were originally. Please help him and design a program which computes the smallest possible original length of those sticks. All lengths expressed in units are integers greater than zero.
Input
The input contains blocks of 2 lines. The first line contains the number of sticks parts after cutting, there are at most 64 sticks. The second line contains the lengths of those parts separated by the space. The last line of the file contains zero.
Output
The output should contains the smallest possible length of original sticks, one per line.
Sample Input
9 5 2 1 5 2 1 5 2 1 4 1 2 3 4 0
Sample Output
6 5
大意:
给你若干小木棒,问他们是有长度最小为多少的等长大木棒分割而成。
思路:
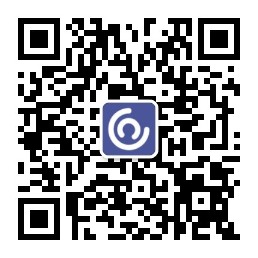
0:参考了各种博客,也询问了很多大佬,总结如下。这个题真mmp.
1:小木棒总长度一定是大木棒的倍数,而大木棒不能比最长的小木棒短。
2:在搜索过程中,如果用a木棒失败,泽和a等长的就可以直接跳过。
3:由大到小排序,便于 2 中等长的判断。
4:关于 3 中为什么要由大到小排序,而不是由小到大。可以想一想:多个较短的小木棒 要比和他们 总和 等长的较长的小木棒拼接起来要灵活,所以我们先拼接长的,留下小的(为了使后面更拼接更方便)。
5:每次遍历小木棒,只需要从上一个拼接位置向后遍历,前面的不用考虑,因为如果前面的能用的话,在之前就已经拼接上了。
6:还有一些细节,我留在了代码中。
代码:
#define NN 110
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
int n,Mlen,a[NN],book[NN];
int cmp(int a,int b)
{
return a>b;
}
int dfs(int N,int now,int lon)
{
int i,j;
if(N==0&&now==0)//当所有的小木棒都拼接为大木棒
return 1;
if(now==0)//成功拼接一根大木棒,去拼接下一个
{
now=Mlen;
lon=0;
}
for(i=lon; i<n; i++)
{
if(!book[i]&&a[i]<=now)
{
if(i>0)
{
if(!book[i-1]&&a[i-1]==a[i]) continue;
//如果当前的没用过的棒子不可用,那么和他长度相同的未使用的棒子也不可用,直接跳过
}
book[i]=1;
if(dfs(N-1,now-a[i],i+1))return 1;
book[i]=0;
//细节
if(a[i]==now||now==Mlen)return 0;
//拼接到这个地方时,一根大木棒已经拼接成功,
//但是后面的却拼接失败,说明之前的拼接有误,要把之前的拼接的拆掉
}
}
return 0;
}
int main()
{
int i,j,sum;
while(scanf("%d",&n)&&n)
{
sum=0;
for(i=0; i<n; i++)
{
scanf("%d",&a[i]);
sum+=a[i];
}
sort(a,a+n,cmp);//从大到小排序,便于深搜
for(i=a[0]; i<=sum/2; i++)
{//从最长的小木棒开始搜
if(sum%i==0)//长度必须是总和的倍数
{
memset(book,0,sizeof(book));
Mlen=i;
if(dfs(n,Mlen,0))
{
printf("%d\n",Mlen);
break;
}
}
}
if(i>sum/2)printf("%d\n",sum);
}
return 0;
}