Problem Description
Fill the following 8 circles with digits 1~8,with each number exactly once . Conntcted circles cannot be filled with two consecutive numbers.
There are 17 pairs of connected cicles:
A-B , A-C, A-D
B-C, B-E, B-F
C-D, C-E, C-F, C-G
D-F, D-G
E-F, E-H
F-G, F-H
G-H
Filling G with 1 and D with 2 (or G with 2 and D with 1) is illegal since G and D are connected and 1 and 2 are consecutive .However ,filling A with 8 and B with 1 is legal since 8 and 1 are not consecutive .
In this problems,some circles are already filled,your tast is to fill the remaining circles to obtain a solution (if possivle).
There are 17 pairs of connected cicles:
A-B , A-C, A-D
B-C, B-E, B-F
C-D, C-E, C-F, C-G
D-F, D-G
E-F, E-H
F-G, F-H
G-H
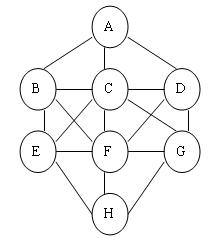
Filling G with 1 and D with 2 (or G with 2 and D with 1) is illegal since G and D are connected and 1 and 2 are consecutive .However ,filling A with 8 and B with 1 is legal since 8 and 1 are not consecutive .
In this problems,some circles are already filled,your tast is to fill the remaining circles to obtain a solution (if possivle).
Input
The first line contains a single integer T(1≤T≤10),the number of test cases. Each test case is a single line containing 8 integers 0~8,the numbers in circle A~H.0 indicates an empty circle.
Output
For each test case ,print the case number and the solution in the same format as the input . if there is no solution ,print “No answer”.If there more than one solution,print “Not unique”.
Sample Input
3 7 3 1 4 5 8 0 0 7 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0
Sample Output
Case 1: 7 3 1 4 5 8 6 2 Case 2: Not unique Case 3: No answer
这道题就是一般的dfs,不过有17条边,需要定义一个check函数
代码:
//
// main.cpp
// 2514
//
// Created by showlo on 2018/5/17.
// Copyright © 2018年 showlo. All rights reserved.
//
#include <iostream>
#include <stdio.h>
using namespace std;
int vis[10];
int a[10],num,ans[10];
int abs(int q) //定义求绝对值的函数
{
if(q<0) return -q;
return q;
}
int check(){ //检查此时的数组是否符合要求
if (abs(a[2]-a[1])!=1&&
abs(a[3]-a[1])!=1&&
abs(a[4]-a[1])!=1&&
abs(a[2]-a[3])!=1&&
abs(a[2]-a[5])!=1&&
abs(a[2]-a[6])!=1&&
abs(a[3]-a[4])!=1&&
abs(a[3]-a[5])!=1&&
abs(a[3]-a[6])!=1&&
abs(a[3]-a[7])!=1&&
abs(a[4]-a[6])!=1&&
abs(a[4]-a[7])!=1&&
abs(a[5]-a[6])!=1&&
abs(a[5]-a[8])!=1&&
abs(a[6]-a[7])!=1&&
abs(a[6]-a[8])!=1&&
abs(a[7]-a[8])!=1
)
return 1;
else
return 0;
}
void dfs(int n){
if(n==9&&check()){ //如果已经到第9个了,就可以进行检查了
num++;
if(num==1){
for(int i=1;i<=8;i++)
ans[i]=a[i];
}
//for(int i=1;i<=8;i++)
// cout<<" "<<ans[i];
//cout<<endl;
return;
}
if(num>=2)
return;
if(a[n]!=0)
dfs(n+1);
else
for (int i=1; i<=8; i++) {
if (vis[i]==0) {
a[n]=i;
vis[i]=1;
dfs(n+1);
vis[i]=0;
a[n]=0;
}
}
}
int main() {
int n,cas=0;
cin>>n;
while (n--) {
num=0;
memset(vis, 0, sizeof(vis));
memset(ans, 0, sizeof(ans));
for(int i=1;i<=8;i++)
cin>>a[i];
for (int i=1; i<=8; i++)
vis[a[i]]=1; //记录已经用过的数
dfs(1);
cout<<"Case "<<++cas<<":";
if(num>=2)
cout<<" Not unique"<<endl;
else if(num==1)
{
for(int i=1;i<=8;i++)
cout<<" "<<ans[i];
cout<<endl;
}
else if(num==0)
cout<<" No answer"<<endl;
}
return 0;
}