Consider the string s
to be the infinite wraparound string of "abcdefghijklmnopqrstuvwxyz", so s
will look like this: "...zabcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcd....".
Now we have another string p
. Your job is to find out how many unique non-empty substrings of p
are present in s
. In particular, your input is the string p
and you need to output the number of different non-empty substrings of p
in the string s
.
Note: p
consists of only lowercase English letters and the size of p might be over 10000.
Example 1:
Input: "a" Output: 1 Explanation: Only the substring "a" of string "a" is in the string s.
Example 2:
Input: "cac" Output: 2 Explanation: There are two substrings "a", "c" of string "cac" in the string s.
Example 3:
Input: "zab" Output: 6 Explanation: There are six substrings "z", "a", "b", "za", "ab", "zab" of string "zab" in the string s.
思路一:暴力求解,将各个子串列出来,判断是否是连续循环的,中间做一些剪枝,但仍会TLE,程序如下所示:
class Solution {
public int findSubstringInWraproundString(String p) {
int len = p.length();
int cnt = 0;
Set<String> set = new HashSet<>();
for (int i = 0; i < len; ++ i){
for (int j = i; j < len; ++ j){
String s = p.substring(i, j+1);
if (set.contains(s)){
continue;
}
set.add(s);
if (checkLegality(s, j + 1 - i)){
cnt ++;
}
else {
break;
}
}
}
return cnt;
}
public boolean checkLegality(String p, int len){
if (len == 1){
return true;
}
for (int i = 1; i < len; ++ i){
if (p.charAt(i) - p.charAt(i-1) == 1 || p.charAt(i-1) - p.charAt(i) == 25){
continue;
}
return false;
}
return true;
}
}
思路二:DP求解,程序如下所示:
class Solution {
public int findSubstringInWraproundString(String p) {
int len = p.length();
if (len == 0){
return 0;
}
int size = 1;
int[] dp = new int[26];
dp[p.charAt(0) - 'a'] = 1;
for (int i = 1; i < len; ++ i){
if (p.charAt(i) - p.charAt(i-1) == 1 || p.charAt(i-1) - p.charAt(i) == 25){
size ++;
}
else {
size = 1;
}
dp[p.charAt(i) - 'a'] = Math.max(dp[p.charAt(i) - 'a'], size);
}
int result = 0;
for (int val : dp){
result += val;
}
return result;
}
}
扫描二维码关注公众号,回复:
2343228 查看本文章
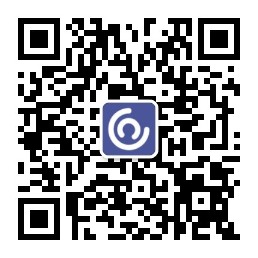