内容来自spring实战第四篇,根据自己理解整理
在上一篇,我们已经了解过spring是如何使用模版去简化业务与数据库链接的逻辑,但是只针对于关系型数据库
spring Data提供了多种对NoSQL数据库的支持,包括MongoDB,Neo4j和Redis。
这里简略说一下,个人理解-MongoDB主要用于文本储存
Neo4j主要用于图片储存
Redis常用数据key-value储存(本编讲解)
第一:链接Redis
Redis链接工厂会生成Redsi数据库服务器的链接。Spring Data Redsi为四种Redis客户端实现提供了链接工厂:
JedisConnectionFactory
JredisConnectionFactory
LettuceConnectionFactory
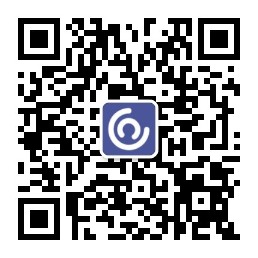
SrpConnectionFactory
简单讲解JedisConnectionFactory,构造器:
内容来自spring官网
Constructor Summary
Constructors Constructor and Description JedisConnectionFactory()
Constructs a newJedisConnectionFactory
instance with default settings (default connection pooling, no shard information).JedisConnectionFactory(redis.clients.jedis.JedisPoolConfig poolConfig)
Constructs a newJedisConnectionFactory
instance using the given pool configuration.JedisConnectionFactory(redis.clients.jedis.JedisShardInfo shardInfo)
Constructs a newJedisConnectionFactory
instance.构造器摘要
构造函数 构造函数和说明 JedisConnectionFactory()
JedisConnectionFactory
使用默认设置构造一个新实例(默认连接池,无分片信息)。JedisConnectionFactory(redis.clients.jedis.JedisPoolConfig poolConfig)
JedisConnectionFactory
使用给定的池配置构造一个新实例。JedisConnectionFactory(redis.clients.jedis.JedisShardInfo shardInfo)
构造一个新的JedisConnectionFactory
实例。
第二:如何最简单实战(提供两种方式:1.注解(推荐),2.xml配置,工具类编写(old function))
1.spring boot配置
#Redis Config spring.redis.database=0 spring.redis.host= spring.redis.port= spring.redis.password= spring.redis.pool.max-active=8 spring.redis.pool.max-wait=-1 spring.redis.pool.max-idle=8 spring.redis.pool.min-idle=0 spring.redis.timeout=0
2.使用标签把数据放入Cache
首先在Class上加入标签:@CacheConfig
@CacheConfig public class ComAppService {
比如说我想把数据库查处的集合放入缓存:
方法讲解,主要标签@Cacheable
@Cacheable(value = "getComApp", key = "'app_'.concat(#comApp.appId)")
public ComApp getComApp(ComApp comApp) {
return mapper.selectOne(comApp);
}
如果使用方法取值和存值使用redisTemplat,这就是spring对redis链接模版,提供set与get方法,粘贴直接用
@Resource private RedisTemplate redisTemplate;
public boolean set(String key, Object value){
boolean result = false;
try {
ValueOperations<Serializable, Object> operations = redisTemplate.opsForValue();
operations.set(key, value);
result = true;
} catch (Exception e) {
LOGGER.error(e.getMessage(), e);
}
return result;
}
public Object get(String key) {
Object result;
ValueOperations<Serializable, Object> operations = redisTemplate.opsForValue();
result = operations.get(key);
return result;
}
2.第二种方案xml配置
这种方式直接贴上配置文件极其代码了
spring-redis.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context"
xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:cache="http://www.springframework.org/schema/cache"
xsi:schemaLocation="
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-4.0.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd ">
<!-- jedis 配置 -->
<bean id="poolConfig" class="redis.clients.jedis.JedisPoolConfig">
<property name="maxIdle" value="${redis.maxIdle}" />
<property name="minIdle" value="${redis.minIdle}" />
<property name="maxWaitMillis" value="${redis.maxWait}" />
<property name="testOnBorrow" value="${redis.testOnBorrow}" />
</bean>
<!-- redis服务器中心 -->
<bean id="connectionFactory"
class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
<property name="poolConfig" ref="poolConfig" />
<property name="port" value="${redis.port}" />
<property name="hostName" value="${redis.host}" />
<property name="password" value="${redis.pass}" />
<property name="timeout" value="${redis.timeout}" />
</bean>
<!-- redis操作模板,面向对象的模板 -->
<bean id="redisTemplate" class="org.springframework.data.redis.core.StringRedisTemplate">
<property name="connectionFactory" ref="connectionFactory" />
<!-- 如果不配置Serializer,那么存储的时候只能使用String,如果用对象类型存储,那么会提示错误 -->
<property name="keySerializer">
<bean
class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<property name="valueSerializer">
<bean
class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" />
</property>
</bean>
</beans>
redis.properties
# Redis Setting
# Redis默认有16个库,序号是0-15,默认是选中的是0号数据库
spring.redis.database=0
# 连接池最大连接数(使用负值表示没有限制),根据实际情况修改
spring.redis.pool.maxActive=8
# Redis服务器地址
redis.host=localhost
# Redis服务器连接端口,默认是6379
redis.port=6379
# Redis服务器连接密码(默认为空)
redis.pass=
# 连接超时时间(毫秒),根据实际情况修改
redis.timeout=-1
# 连接池中的最大空闲连接,根据实际情况修改
redis.maxIdle=100
# 连接池中的最小空闲连接,根据实际情况修改
redis.minIdle=8
# 连接池最大阻塞等待时间(使用负值表示没有限制),根据实际情况修改
redis.maxWait=-1
redis.testOnBorrow=true
redisUtils
package com.shen.utils;
import java.util.concurrent.TimeUnit;
import javax.annotation.Resource;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.stereotype.Component;
/**
* 操作 hash 的基本操作
*
* @author sy
* 有额外需求 可以在这个网站 http://blog.csdn.net/u011911084/article/details/53435172
*/
@Component("redisCache")
public class RedisCacheUtil {
@Resource
private StringRedisTemplate redisTemplate;
/**
* 获取缓存的地址
*
* @param cacheKey
* @return
*/
public String getCacheValue(String cacheKey) {
String cacheValue = (String) redisTemplate.opsForValue().get(cacheKey);
return cacheValue;
}
/**
* 设置缓存值
*
* @param key
* @param value
*/
public void setCacheValue(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
/**
* 设置缓存值并设置有效期
*
* @param key
* @param value
*/
public void setCacheValueForTime(String key, String value, long time) {
redisTemplate.opsForValue().set(key, value, time, TimeUnit.SECONDS);
}
/**
* 删除key值
*
* @param key
*/
public void delCacheByKey(String key) {
redisTemplate.opsForValue().getOperations().delete(key);
redisTemplate.opsForHash().delete("");
}
/**
* 获取token的有效期
*
* @param key
*/
public long getExpireTime(String key) {
long time = redisTemplate.getExpire(key);
return time;
}
/**
* 指定时间类型---秒
*
* @param key
* @return
*/
public long getExpireTimeType(String key) {
long time = redisTemplate.getExpire(key, TimeUnit.SECONDS);
return time;
}
/**
*
* @param key---分
* @return
*/
public long getExpireTimeTypeForMin(String key) {
long time = redisTemplate.getExpire(key, TimeUnit.MINUTES);
return time;
}
/**
* 设置一个自增的数据
*
* @param key
* @param growthLength
*/
public void testInc(String key, Long growthLength) {
redisTemplate.opsForValue().increment(key, growthLength);
}
}