import numpy as np
import matplotlib.pyplot as plt
import matplotlib
from sklearn.model_selection import train_test_split
from sklearn import datasets
digits = datasets.load_digits()
digits
{'data': array([[ 0., 0., 5., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 10., 0., 0.],
[ 0., 0., 0., ..., 16., 9., 0.],
...,
[ 0., 0., 1., ..., 6., 0., 0.],
[ 0., 0., 2., ..., 12., 0., 0.],
[ 0., 0., 10., ..., 12., 1., 0.]]),
'target': array([0, 1, 2, ..., 8, 9, 8]),
'target_names': array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]),
'images': array([[[ 0., 0., 5., ..., 1., 0., 0.],
[ 0., 0., 13., ..., 15., 5., 0.],
[ 0., 3., 15., ..., 11., 8., 0.],
...,
[ 0., 4., 11., ..., 12., 7., 0.],
[ 0., 2., 14., ..., 12., 0., 0.],
[ 0., 0., 6., ..., 0., 0., 0.]],
[[ 0., 0., 0., ..., 5., 0., 0.],
[ 0., 0., 0., ..., 9., 0., 0.],
[ 0., 0., 3., ..., 6., 0., 0.],
...,
[ 0., 0., 1., ..., 6., 0., 0.],
[ 0., 0., 1., ..., 6., 0., 0.],
[ 0., 0., 0., ..., 10., 0., 0.]],
[[ 0., 0., 0., ..., 12., 0., 0.],
[ 0., 0., 3., ..., 14., 0., 0.],
[ 0., 0., 8., ..., 16., 0., 0.],
...,
[ 0., 9., 16., ..., 0., 0., 0.],
[ 0., 3., 13., ..., 11., 5., 0.],
[ 0., 0., 0., ..., 16., 9., 0.]],
...,
[[ 0., 0., 1., ..., 1., 0., 0.],
[ 0., 0., 13., ..., 2., 1., 0.],
[ 0., 0., 16., ..., 16., 5., 0.],
...,
[ 0., 0., 16., ..., 15., 0., 0.],
[ 0., 0., 15., ..., 16., 0., 0.],
[ 0., 0., 2., ..., 6., 0., 0.]],
[[ 0., 0., 2., ..., 0., 0., 0.],
[ 0., 0., 14., ..., 15., 1., 0.],
[ 0., 4., 16., ..., 16., 7., 0.],
...,
[ 0., 0., 0., ..., 16., 2., 0.],
[ 0., 0., 4., ..., 16., 2., 0.],
[ 0., 0., 5., ..., 12., 0., 0.]],
[[ 0., 0., 10., ..., 1., 0., 0.],
[ 0., 2., 16., ..., 1., 0., 0.],
[ 0., 0., 15., ..., 15., 0., 0.],
...,
[ 0., 4., 16., ..., 16., 6., 0.],
[ 0., 8., 16., ..., 16., 8., 0.],
[ 0., 1., 8., ..., 12., 1., 0.]]]),
'DESCR': "Optical Recognition of Handwritten Digits Data Set\n===================================================\n\nNotes\n-----\nData Set Characteristics:\n :Number of Instances: 5620\n :Number of Attributes: 64\n :Attribute Information: 8x8 image of integer pixels in the range 0..16.\n :Missing Attribute Values: None\n :Creator: E. Alpaydin (alpaydin '@' boun.edu.tr)\n :Date: July; 1998\n\nThis is a copy of the test set of the UCI ML hand-written digits datasets\nhttp://archive.ics.uci.edu/ml/datasets/Optical+Recognition+of+Handwritten+Digits\n\nThe data set contains images of hand-written digits: 10 classes where\neach class refers to a digit.\n\nPreprocessing programs made available by NIST were used to extract\nnormalized bitmaps of handwritten digits from a preprinted form. From a\ntotal of 43 people, 30 contributed to the training set and different 13\nto the test set. 32x32 bitmaps are divided into nonoverlapping blocks of\n4x4 and the number of on pixels are counted in each block. This generates\nan input matrix of 8x8 where each element is an integer in the range\n0..16. This reduces dimensionality and gives invariance to small\ndistortions.\n\nFor info on NIST preprocessing routines, see M. D. Garris, J. L. Blue, G.\nT. Candela, D. L. Dimmick, J. Geist, P. J. Grother, S. A. Janet, and C.\nL. Wilson, NIST Form-Based Handprint Recognition System, NISTIR 5469,\n1994.\n\nReferences\n----------\n - C. Kaynak (1995) Methods of Combining Multiple Classifiers and Their\n Applications to Handwritten Digit Recognition, MSc Thesis, Institute of\n Graduate Studies in Science and Engineering, Bogazici University.\n - E. Alpaydin, C. Kaynak (1998) Cascading Classifiers, Kybernetika.\n - Ken Tang and Ponnuthurai N. Suganthan and Xi Yao and A. Kai Qin.\n Linear dimensionalityreduction using relevance weighted LDA. School of\n Electrical and Electronic Engineering Nanyang Technological University.\n 2005.\n - Claudio Gentile. A New Approximate Maximal Margin Classification\n Algorithm. NIPS. 2000.\n"}
X = digits.data
y = digits.target
some_digit = X[666]
some_digit.shape
(64,)
y[666]
0
some_digit_image = some_digit.reshape(8, 8)
plt.imshow(some_digit_image, cmap=matplotlib.cm.binary)
<matplotlib.image.AxesImage at 0x7fe5bb710588>
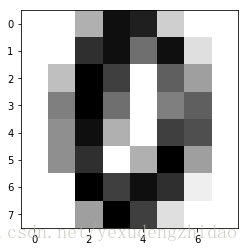
对原始数据进行分类train_test_split
X_train, X_test,y_train, y_test = train_test_split(X, y, test_size=0.2)
y_train
array([7, 5, 5, ..., 0, 0, 7])
创建一个kNN分类器对象
from sklearn.neighbors import KNeighborsClassifier
kNN_classifier = KNeighborsClassifier(n_neighbors=3)
kNN_classifier.fit(X_train, y_train)
KNeighborsClassifier(algorithm='auto', leaf_size=30, metric='minkowski',
metric_params=None, n_jobs=1, n_neighbors=3, p=2,
weights='uniform')
y_predict = kNN_classifier.predict(X_test)
y_test
array([9, 8, 5, 2, 3, 0, 2, 5, 3, 5, 7, 7, 9, 4, 8, 6, 7, 4, 3, 9, 6, 3,
9, 1, 2, 5, 4, 5, 3, 1, 3, 7, 2, 0, 2, 3, 3, 3, 6, 6, 6, 5, 9, 3,
0, 9, 1, 3, 9, 2, 4, 0, 8, 2, 4, 1, 2, 3, 5, 4, 8, 5, 4, 3, 3, 9,
3, 9, 7, 3, 9, 6, 9, 6, 6, 2, 2, 4, 1, 6, 2, 3, 3, 6, 3, 1, 4, 5,
4, 5, 0, 0, 8, 2, 4, 1, 1, 0, 6, 7, 9, 1, 3, 9, 5, 9, 5, 7, 8, 7,
3, 7, 0, 6, 2, 4, 4, 2, 8, 7, 0, 0, 3, 8, 9, 4, 9, 1, 8, 2, 1, 2,
0, 0, 1, 8, 4, 3, 2, 5, 9, 1, 8, 0, 0, 5, 0, 1, 8, 9, 6, 0, 7, 6,
1, 2, 6, 9, 7, 4, 4, 6, 4, 4, 9, 4, 4, 7, 4, 5, 1, 3, 2, 4, 3, 7,
2, 7, 2, 6, 5, 7, 5, 9, 1, 6, 5, 1, 3, 1, 3, 3, 7, 7, 2, 0, 5, 5,
1, 3, 4, 9, 9, 1, 6, 3, 4, 5, 2, 7, 8, 1, 3, 9, 4, 0, 8, 3, 3, 9,
2, 8, 5, 1, 1, 8, 0, 8, 7, 4, 3, 4, 4, 8, 5, 5, 2, 7, 3, 2, 6, 5,
8, 3, 2, 5, 0, 5, 7, 6, 4, 7, 9, 6, 4, 7, 5, 3, 7, 5, 2, 1, 4, 8,
4, 4, 1, 4, 2, 4, 7, 8, 2, 7, 5, 1, 2, 6, 2, 5, 0, 9, 6, 2, 4, 3,
9, 7, 7, 7, 0, 9, 8, 6, 3, 6, 1, 5, 1, 8, 0, 6, 8, 6, 5, 6, 6, 3,
1, 6, 1, 4, 6, 0, 2, 1, 4, 7, 9, 8, 2, 5, 3, 3, 3, 4, 7, 3, 7, 8,
7, 2, 9, 4, 6, 2, 5, 5, 9, 8, 2, 6, 9, 4, 6, 9, 9, 0, 6, 6, 8, 1,
8, 3, 9, 0, 4, 8, 0, 2])
sum(y_predict == y_test)/len(y_test)
0.9916666666666667
使用scikit-learn中的accuracy_score
from sklearn.metrics import accuracy_score
accuracy_score(y_predict, y_test)
0.9916666666666667
kNN_classifier.score(X_test, y_test)
0.9916666666666667
在手写识别中寻找最好的k
best_method = ''
best_score = 0.0
best_k = -1
for method in ['uniform', 'distance']:
for k in range(1, 11):
kNN_classifier = KNeighborsClassifier(n_neighbors=k, weights=method)
kNN_classifier.fit(X_train, y_train)
score = kNN_classifier.score(X_test, y_test)
if score > best_score:
best_score = score
best_k = k
best_method = method
print('best_k is %s'% best_k)
print('best_method is %s'% best_method)
best_k is 3
best_method is uniform