有意见请随时指教,本人基础还比较浅薄。谢谢
今天第一次使用springboot2.0版本集成redis,在参考http://412887952-qq-com.iteye.com/blog/2294942 进行集成的时候遇见了如下问题:
RedisCacheManager类初始化不再能以RedisTemplate为参数进行初始化,取而代之,官方(https://docs.spring.io/spring-data/redis/docs/2.0.5.RELEASE/reference/html/#new-in-2.0.0)给出了另一种初始化RedisCacheManager的方法:
RedisCacheManager在新版本中移除了该方法,所以不能再使用,若要使用则需要将spring-data-redis版本降低,1.6.0.RELEASE此方法是可用的,但是不推荐此方法,除非整体降低springboot的版本。
然后去查看spring2.0的官方文档,发下了如下描述:
1、添加spring-boot-starter-data-redis依赖(该依赖版本继承spring-boot版本)
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、按照文档描述创建了Bean:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.stereotype.Component;
@Component
public class MyRedisConfigBean {
@Autowired
private StringRedisTemplate template;
//使用StringRedisTemplate进行所需操作
}
3、应该注意到,获得连接池,则应该添加common-pool2.jar的依赖
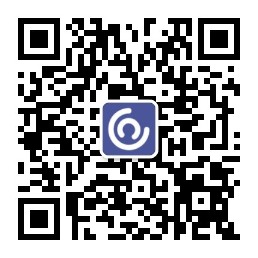
<!--spring2.0集成redis所需common-pool2-->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.4.2</version>
</dependency>
经测,2.5.0版本的该jar包缺少方法,2.4.2可用。
以上就是spring2.0简单集成redis的步骤。
测试方法见:
http://412887952-qq-com.iteye.com/blog/2294942
第6-10步
补充:
在编写测试类,使用@Resource注解注入RedisTemplate时
@Service
public class DemoInfoServiceImpl implements DemoInfoService{
@Autowired
private RedisTemplate redisTemplate;
@Override
public void test() {
ValueOperations<String,String> valueOperations = redisTemplate.opsForValue();
valueOperations.set("mykey4","random1="+Math.random());
System.out.println(valueOperations.get("mykey4"));
}
}
使用test方法向redis存储值的时候会在key值前出现\xAC\xED\x00\x05t\x00\x06等乱码。
经查阅,有两种解决方法:
1、
private RedisTemplate redisTemplate; @Autowired(required = false) public void setRedisTemplate(RedisTemplate redisTemplate) { RedisSerializer stringSerializer = new StringRedisSerializer(); redisTemplate.setKeySerializer(stringSerializer); redisTemplate.setValueSerializer(stringSerializer); redisTemplate.setHashKeySerializer(stringSerializer); redisTemplate.setHashValueSerializer(stringSerializer); this.redisTemplate = redisTemplate; }
为redistemplates手动设置StringSerializer,即可解决以上问题。
2、查看org.springframework.boot.autoconfigure.data.redis.RedisAutoConfiguration代码,有如下配置:
@Configuration @ConditionalOnClass({ RedisOperations.class }) @EnableConfigurationProperties(RedisProperties.class) @Import({ LettuceConnectionConfiguration.class, JedisConnectionConfiguration.class }) public class RedisAutoConfiguration { @Bean @ConditionalOnMissingBean(name = "redisTemplate") public RedisTemplate<Object, Object> redisTemplate( RedisConnectionFactory redisConnectionFactory) throws UnknownHostException { RedisTemplate<Object, Object> template = new RedisTemplate<>(); template.setConnectionFactory(redisConnectionFactory); return template; } @Bean @ConditionalOnMissingBean(StringRedisTemplate.class) public StringRedisTemplate stringRedisTemplate( RedisConnectionFactory redisConnectionFactory) throws UnknownHostException { StringRedisTemplate template = new StringRedisTemplate(); template.setConnectionFactory(redisConnectionFactory); return template; } }
可以看出,在自动配置的时候配置有StringRedisTemplate和RedisTemplate两种,则直接注入StringRedisTemplate 即可优雅的解决问题。
代码如下:
package com.imooc.firstappdemo.redis.service; import com.imooc.firstappdemo.redis.domain.DemoInfo; import com.imooc.firstappdemo.redis.repository.DemoInfoRepository; import com.imooc.firstappdemo.service.DemoService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.cache.annotation.CacheEvict; import org.springframework.cache.annotation.Cacheable; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.core.StringRedisTemplate; import org.springframework.data.redis.core.ValueOperations; import org.springframework.data.redis.serializer.RedisSerializer; import org.springframework.data.redis.serializer.StringRedisSerializer; import org.springframework.stereotype.Service; import javax.annotation.Resource; import javax.sound.midi.Soundbank; import java.util.Optional; @Service public class DemoInfoServiceImpl implements DemoInfoService{ @Autowired private StringRedisTemplate stringRedisTemplate; @Override public void test() { stringRedisTemplate.setStringSerializer(); ValueOperations<String,String> valueOperations = stringRedisTemplate.opsForValue(); valueOperations.set("mykey4","random1="+Math.random()); System.out.println(valueOperations.get("mykey4")); } }
ps:实际上StringRedisTemplate是RedisTemplate的子类:
public class StringRedisTemplate extends RedisTemplate<String, String> { public StringRedisTemplate() { RedisSerializer<String> stringSerializer = new StringRedisSerializer(); this.setKeySerializer(stringSerializer); this.setValueSerializer(stringSerializer); this.setHashKeySerializer(stringSerializer); this.setHashValueSerializer(stringSerializer); } public StringRedisTemplate(RedisConnectionFactory connectionFactory) { this(); this.setConnectionFactory(connectionFactory); this.afterPropertiesSet(); } protected RedisConnection preProcessConnection(RedisConnection connection, boolean existingConnection) { return new DefaultStringRedisConnection(connection); } }
主要就是再RedisTemplate基础上设置了Serializer。