1. vuex,是为了搞定组件间通信问题(关键在于集中式存储管理)
2. 创建一个store.js文件
import Vue from "vue"
import Vuex from "vuex"
Vue.use(Vuex)
const store = new Vuex.Store({
state: { //这里的state必须是JSON,是一个对象
count: 1 //这是初始值
},
mutations: {//突变,罗列所有可能改变state的方法
add(state) {
state.count++; //直接改变了state中的值,而并不是返回了一个新的state
},
reduce(state){
state.count--;
}
}
});
export default store;//用export default 封装代码,让外部可以引用
在main.js文件中引入store.js文件
import store from "./vuex/store"
new Vue({
router,
store,
el: '#app',
render: h => h(App)
})
新建一个模板文件Count.vue
<template>
<div>
<h2>{{msg}}</h2><hr/>
<h2>{{$store.state.count}}-{{count}}</h2>//这两种写法都可以
<button @click="addNumber">+</button>
<button @click="reduceNumber">-</button>
</div>
</template>
<script>
import {mapState} from 'vuex'
export default {
data() {
return {
msg: "Hello Vuex"
};
},
methods: {
addNumber() {
return this.$store.commit("add");
},
reduceNumber() {
return this.$store.commit("reduce");
}
},
computed: mapState(['count'])// 映射 this.count 到 this.$store.state.count
mapState 函数可以接受一个对象,也可以接收一个数组
};
</script>
3. 改变store 中的状态的唯一途径就是显式地提交 (commit) mutations。
4. 如何在Mutations里传递参数
先store.js文件里给add方法加上一个参数n
mutations: {
add(state,n) {
state.count+=n;
},
reduce(state){
state.count--;
}
}
然后在Count.vue里修改按钮的commit( )方法传递的参数
addNumber() {
return this.$store.commit("add",2);
},
reduceNumber() {
return this.$store.commit("reduce");
}
5. getters如何实现计算过滤操作
getters从表面是获得的意思,可以把他看作在获取数据之前进行的一种再编辑,相当于对数据的一个过滤和加工。你可以把它看作store.js的计算属性。
例如:要对store.js文件中的count进行操作,在它输出前,给它加上100。
扫描二维码关注公众号,回复:
1898740 查看本文章
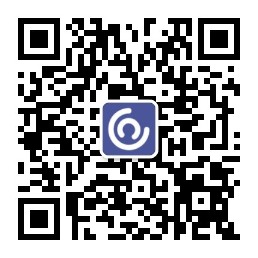
首先要在store.js里Vuex.Store()里引入getters
getters:{
count:state=>state.count+=100
}
然后在Count.vue中对computed进行配置,在vue 的构造器里边只能有一个computed属性,如果你写多个,只有最后一个computed属性可用,所以要用展开运算符”…”对上节写的computed属性进行一个改造。
computed: {
...mapState(["count"]),
count() {
return this.$store.getters.count;
}
}
6. 最后的结果是mutations和getters共同作用的