8、商品类别-类
package com.imooc.o2o.entity;
import java.util.Date;
public class ProductCategory {
private Long productCategoryId;
private Long shopId;
private String productCategoryName;
private Integer priority;
private Date createTime;
public Long getProductCategoryId() {
return productCategoryId;
}
public void setProductCategoryId(Long productCategoryId) {
this.productCategoryId = productCategoryId;
}
public Long getShopId() {
return shopId;
}
public void setShopId(Long shopId) {
this.shopId = shopId;
}
public String getProductCategoryName() {
return productCategoryName;
}
public void setProductCategoryName(String productCategoryName) {
this.productCategoryName = productCategoryName;
}
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
}
CREATE TABLE `tb_product_category` (
`product_category_id` int(11) NOT NULL AUTO_INCREMENT,
`product_category_name` varchar(100) NOT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY (`product_category_id`),
CONSTRAINT `fk_procate_shop` FOREIGN KEY (`shop_id`) REFERENCES `tb_shop` (`shop_id`)
) ENGINE=InnoDB AUTO_INCREMENT=16 DEFAULT CHARSET=utf8;
9、商品-类
package com.imooc.o2o.entity;
import java.util.Date;
import java.util.List;
public class Product {
private Long productId;
private String productName;
private String productDesc;
private String imgAddr;
private String normalPrice;
private String promotionPrice;
private Integer priority;
private Date createTime;
private Date lastEditTime;
private Integer enableStatus;
private List<ProductImg> productImgList;
private ProductCategory productCategory;
private Shop shop;
public Long getProductId() {
return productId;
}
public void setProductId(Long productId) {
this.productId = productId;
}
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public String getProductDesc() {
return productDesc;
}
public void setProductDesc(String productDesc) {
this.productDesc = productDesc;
}
public String getImgAddr() {
return imgAddr;
}
public void setImgAddr(String imgAddr) {
this.imgAddr = imgAddr;
}
public String getNormalPrice() {
return normalPrice;
}
public void setNormalPrice(String normalPrice) {
this.normalPrice = normalPrice;
}
public String getPromotionPrice() {
return promotionPrice;
}
public void setPromotionPrice(String promotionPrice) {
this.promotionPrice = promotionPrice;
}
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
public Date getLastEditTime() {
return lastEditTime;
}
public void setLastEditTime(Date lastEditTime) {
this.lastEditTime = lastEditTime;
}
public Integer getEnableStatus() {
return enableStatus;
}
public void setEnableStatus(Integer enableStatus) {
this.enableStatus = enableStatus;
}
public List<ProductImg> getProductImgList() {
return productImgList;
}
public void setProductImgList(List<ProductImg> productImgList) {
this.productImgList = productImgList;
}
public ProductCategory getProductCategory() {
return productCategory;
}
public void setProductCategory(ProductCategory productCategory) {
this.productCategory = productCategory;
}
public Shop getShop() {
return shop;
}
public void setShop(Shop shop) {
this.shop = shop;
}
}
CREATE TABLE `tb_product` (
`product_id` int(100) NOT NULL AUTO_INCREMENT,
`product_name` varchar(100) NOT NULL,
`product_desc` varchar(2000) DEFAULT NULL,
`img_addr` varchar(2000) DEFAULT '',
`normal_price` varchar(100) DEFAULT NULL,
`promotion_price` varchar(100) DEFAULT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`last_edit_time` datetime DEFAULT NULL,
`enable_status` int(2) NOT NULL DEFAULT '0',
`point` int(10) DEFAULT NULL,
`product_category_id` int(11) DEFAULT NULL,
`shop_id` int(20) NOT NULL DEFAULT '0',
PRIMARY KEY (`product_id`),
KEY `fk_product_shop` (`shop_id`),
KEY `fk_product_procate` (`product_category_id`),
CONSTRAINT `fk_product_procate` FOREIGN KEY (`product_category_id`) REFERENCES `tb_product_category` (`product_category_id`),
CONSTRAINT `fk_product_shop` FOREIGN KEY (`shop_id`) REFERENCES `tb_shop` (`shop_id`)
) ENGINE=InnoDB AUTO_INCREMENT=16 DEFAULT CHARSET=utf8;
10、详情图片-类
package com.imooc.o2o.entity;
import java.util.Date;
public class ProductImg {
private Long productImgId;
private String imgAddr;
private String imgDesc;
private Integer priority;
private Date createTime;
private Long productId;
public Long getProductImgId() {
return productImgId;
}
public void setProductImgId(Long productImgId) {
this.productImgId = productImgId;
}
public String getImgAddr() {
return imgAddr;
}
public void setImgAddr(String imgAddr) {
this.imgAddr = imgAddr;
}
public String getImgDesc() {
return imgDesc;
}
public void setImgDesc(String imgDesc) {
this.imgDesc = imgDesc;
}
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
public Long getProductId() {
return productId;
}
public void setProductId(Long productId) {
this.productId = productId;
}
}
CREATE TABLE `tb_product_img` (
`product_img_id` int(20) NOT NULL AUTO_INCREMENT,
`img_addr` varchar(2000) NOT NULL,
`img_desc` varchar(2000) DEFAULT NULL,
`priority` int(2) DEFAULT '0',
`create_time` datetime DEFAULT NULL,
`product_id` int(20) DEFAULT NULL,
PRIMARY KEY (`product_img_id`),
KEY `fk_proimg_product` (`product_id`),
CONSTRAINT `fk_proimg_product` FOREIGN KEY (`product_id`) REFERENCES `tb_product` (`product_id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=38 DEFAULT CHARSET=utf8;
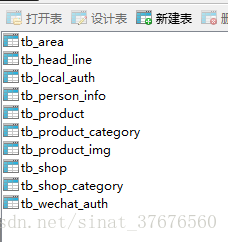