参考文档:https://blog.csdn.net/h295928126/article/details/53587142?locationNum=2&fps=1
1、数据库创建表(测试数据可以自己随机插入,我使用的数据库为mysql)
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for topic
-- ----------------------------
DROP TABLE IF EXISTS `topic`;
CREATE TABLE `topic` (
`id` varchar(255) NOT NULL,
`name` varchar(255) DEFAULT NULL,
`teacher` varchar(255) DEFAULT NULL,
`number` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
-- ----------------------------
-- Records of topic
-- ----------------------------
INSERT INTO `topic` VALUES ('1', '1111', '1111', '2-16');
2、创建一个springboot项目
详细可参考我的文章:idea+springboot+mybatis+jsp
改动的地方是这一次我选择的数据库是mysql,还加了一个搜索引擎模板thymeleaf
生成pom.xml中的配置如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com</groupId>
<artifactId>boot</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>boot</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
<!--后来加的-->
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
</resources>
</build>
</project>
application.properties中配置如下:
#填自己的数据库信息
spring.datasource.url=jdbc:mysql://localhost:3306/test
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
#mybatis的路径
mybatis.mapperLocation=classpath*:com/**/*Mapper.xml
接下来创建项目结构:如下如所示
详细类的代码如下所示:
扫描二维码关注公众号,回复:
1769000 查看本文章
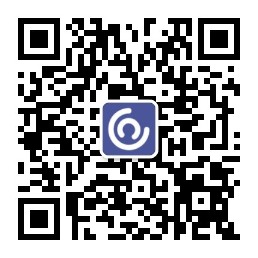
model 中Topic类
@Data
public class Topic {
private String id ;
private String name;
private String teacher;
private String number;
}
由于data的加入,pom.xml中增加如下依赖。
写data注解,就可以不用手动添加get set方法
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.12</version>
</dependency>
mapper中的TopicMapper
@Mapper
public interface TopicMapper {
List<Topic> findAll();
Topic findById(String id);
void update(Topic topic);
}
mapper中的TopicMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.mapper.TopicMapper" >
<resultMap id="TopicResultMap" type="com.model.Topic">
<id column="id" property="id" jdbcType="VARCHAR"/>
<result column="name" property="name" jdbcType="VARCHAR"/>
<result column="teacher" property="teacher" jdbcType="INTEGER"/>
<result column="number" property="number" jdbcType="INTEGER"/>
</resultMap>
<select id="findAll" parameterType="String" resultMap="TopicResultMap">
select * from topic
</select>
</mapper>
service中TopicService 类
public interface TopicService {
List<Topic> findAll();
String check(Topic topic);
}
service中TopicServiceImpl 类
@SuppressWarnings("SpringJavaAutowiringInspection")
@Service("topicService")
public class TopicServiceImpl implements TopicService {
@Autowired
private TopicMapper topicMapper;
@Override
public List<Topic> findAll() {
return topicMapper.findAll();
}
}
controller中的UserController类
@Controller
@RequestMapping("")
public class UserController {
@Autowired
private TopicService topicService;
//选题系统首页
@RequestMapping(value = "/topic",method = RequestMethod.GET)
public ModelAndView getTopicAll(Model model){
List<Topic> topics=topicService.findAll();
model.addAttribute("topics",topics);
return new ModelAndView("act/model");
}
}
new ModelAndView(“act/model”)调转后的路径templates下创建的html页面
界面model.html中的代码:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="http://www.thymeleaf.org"
xmlns:text-align="http://www.w3.org/1999/xhtml">
<head>
<title>简单粗暴选题系统!</title>
<link href="css/main.css" rel="stylesheet" type="text/css" />
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<meta http-equiv="X-UA-COMPATIBLE" content="IE=edge,chrome=1" />
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no" />
</head>
<body>
<h1>简单粗暴选题系统!</h1>
<h2>题目列表</h2>
<table width="400" border="1" cellspacing="0" cellpadding="0">
<thead>
<tr>
<th width="105">序号</th>
<th width="105">题目</th>
<th width="105">老师</th>
<th width="105">已选学号(0为未有人选)</th>
</tr>
</thead>
<tbody>
<tr th:each="topic:${topics}">
<td width="105" th:text="${topic.id}">序号</td>
<td width="105" th:text="${topic.name}">题目</td>
<td width="105" th:text="${topic.teacher}">老师</td>
<td width="105" th:text="${topic.number}">已选学号</td>
</tr>
</tbody>
</table>
<form action="/check" method="get">
<p>选择你要选的题目的序号: <input type="text" name="id" /></p>
<p>你的学号(比如二班1号:“2-1”): <input type="text" name="number" /></p>
<input type="submit" value="确认" />
</form>
</body>
</html>
特别注意:
<tr th:each="topic:${topics}">
<td width="105" th:text="${topic.id}">序号</td>
<td width="105" th:text="${topic.name}">题目</td>
<td width="105" th:text="${topic.teacher}">老师</td>
<td width="105" th:text="${topic.number}">已选学号</td>
</tr>
和jsp的区别 类型前加上th:
至此集合thymeleaf的搭建完成,界面显示如下: