一、什么是 Robot Framework?
1. Robot Framework 的历史由来
Robot Framework是一种通用的自动化测试框架,最早由Pekka Klärck在2005年开发,并由Nokia Networks作为内部工具使用。后来,该项目以开源形式发布,并得到了广泛的社区支持和贡献。
2.官方介绍:
Robot Framework is a generic open source automation framework. It can be used for test automation and robotic process automation (RPA).
Robot Framework 是一个通用的开源自动化框架。它可用于测试自动化和机器人流程自动化 (RPA)。
Robot Framework is supported by Robot Framework Foundation. Many industry-leading companies use the tool in their software development.
Robot Framework 由 Robot Framework Foundation 提供支持。许多行业领先的公司在其软件开发中使用该工具。
Robot Framework is open and extensible. Robot Framework can be integrated with virtually any other tool to create powerful and flexible automation solutions. Robot Framework is free to use without licensing costs.
机器人框架是开放且可扩展的。Robot Framework几乎可以与任何其他工具集成,以创建强大而灵活的自动化解决方案。Robot Framework 可免费使用,无需许可费用。
Robot Framework has an easy syntax, utilizing human-readable keywords. Its capabilities can be extended by libraries implemented with Python, Java or many other programming languages. Robot Framework has a rich ecosystem around it, consisting of libraries and tools that are developed as separate projects.
Robot Framework 具有简单的语法,使用人类可读的关键字。它的功能可以通过使用 Python、Java 或许多其他编程语言实现的库来扩展。Robot Framework 拥有丰富的生态系统,由作为独立项目开发的库和工具组成。
3. 基本语法
- 测试用例以
*** Test Cases ***
开头,后跟一个或多个测试用例。 - 关键字定义以
*** Keywords ***
开头,后跟一个或多个关键字定义。 - 测试用例和关键字定义使用缩进来表示层次结构。
- 关键字和关键字参数之间使用制表符或多个空格分隔。
4. Code is worth a thousand words代码胜过千言万语
简单示例
此示例包含用户登录的单个测试用例。它使用模拟的后端 API 进行用户管理。该测试套件TestSuite.robot包含两个测试用例“使用密码登录用户”和“使用错误密码拒绝登录”。此测试用例从资源文件 keywords.resource 调用关键字。
TestSuite.robot:
*** Settings ***
Documentation A test suite for valid login.
...
... Keywords are imported from the resource file
Resource keywords.resource
Default Tags positive
*** Test Cases ***
Login User with Password
Connect to Server
Login User ironman 1234567890
Verify Valid Login Tony Stark
[Teardown] Close Server Connection
Denied Login with Wrong Password
[Tags] negative
Connect to Server
Run Keyword And Expect Error *Invalid Password Login User ironman 123
Verify Unauthorised Access
[Teardown] Close Server Connection
Resource File 资源文件
keywords.resource 包含关键字定义。资源文件还使用库 CustomLibrary.py 中基于 python 的关键字来实现更专业的功能。存在无数社区制作的库,它们以各种方式扩展了机器人框架!
keywords.resource:
*** Settings ***
Documentation This is a resource file, that can contain variables and keywords.
... Keywords defined here can be used where this Keywords.resource in loaded.
Library CustomLibrary.py
*** Keywords ***
Connect to Server
Connect fe80::aede:48ff:fe00:1122
Close Server Connection
Disconnect
Login User
[Arguments] ${
login} ${
password}
Set Login Name ${
login}
Set Password ${
password}
Execute Login
Verify Valid Login
[Arguments] ${
exp_full_name}
${
version}= Get Server Version
Should Not Be Empty ${
version}
${
name}= Get User Name
Should Be Equal ${
name} ${
exp_full_name}
Verify Unauthorised Access
Run Keyword And Expect Error PermissionError* Get Server Version
Login Admin
[Documentation] 'Login Admin' is a Keyword.
... It calls 'Login User' from 'CustomLibrary.py'
Login User admin @RBTFRMWRK@
Verify Valid Login Administrator
CustomLibrary.py:
from TestObject import TestObject
from robot.api.logger import info, debug, trace, console
class CustomLibrary:
'''This is a user written keyword library.
These libraries can be pretty handy for more complex tasks an typically
more efficiant to implement compare to Resource files.
However, they are less simple in syntax and less transparent in test protocols.
The TestObject object (t) has the following public functions:
class TestObject:
def authenticate(self, login: str, password: str) -> str: ...
def logout(self, token): ...
def get_version(self, token) -> str: ...
def get_user_id(self, token, login) -> str: ...
def get_user_name(self, token, user_id) -> str: ...
def get_user(self, token, user_id=None) -> Dict[str, str]: ...
def get_user_all(self, token) -> List[Dict[str, str]]: ...
def delete_user(self, token, userid): ...
def get_logout(self, token): ...
def put_user_password(self, token, new_password, user_id=None): ...
def put_user_name(self, token, name, user_id=None): ...
def put_user_right(self, token, right, user_id): ...
def post_new_user(self, token, name, login) -> str: ...
'''
ROBOT_LIBRARY_SCOPE = 'SUITE'
def __init__(self) -> None:
self._session = None
self.login = ''
self.password = ''
self._connection: TestObject = None
def connect(self, ip):
self._connection = TestObject(ip)
def disconnect(self):
self._connection = None
@property
def connection(self):
if not self._connection:
raise SystemError('No Connection established! Connect to server first!')
return self._connection
@property
def session(self):
if self._session is None:
raise PermissionError('No valid user session. Authenticate first!')
return self._session
def set_login_name(self, login):
'''Sets the users login name and stores it for authentication.'''
self.login = login
info(f'User login set to: {
login}')
def set_password(self, password):
'''Sets the users login name and stores it for authentication.'''
self.password = password
info(f'Password set.')
def execute_login(self):
'''Triggers the authentication process at the backend and stores the session token.'''
self._session = self.connection.authenticate(self.login, self.password)
if self.session:
info(f'User session successfully set.')
debug(f'Session token is: {
self.session}')
self.login = self.password = ''
def login_user(self, login, password) -> None:
'''`Login User` authenticates a user to the backend.
The session will be stored during this test suite.'''
self._session = self.connection.authenticate(login, password)
def logout_user(self):
'''Logs out the current user.'''
self.connection.logout(self.session)
def create_new_user(self, name, login, password, right):
'''Creates a new user with the give data.'''
user_id = self.connection.post_new_user(self.session, name, login)
self.connection.put_user_password(self.session, password, user_id=user_id)
self.connection.put_user_right(self.session, right, user_id)
def change_own_password(self, new_password, old_password):
'''Changes the own password given the new and current one.'''
self.connection.put_user_password(self.session, new_password, old_password)
def change_users_password(self, login, new_password):
'''Changes the password of a user by its name.
Requires Admin priviliges!'''
user_id = self.get_user_id(login)
self.connection.put_user_password(self.session, new_password, user_id=user_id)
def get_all_users(self):
'''`Get All Users` does return a list of user-dictionaries.
A user dictionary has the keys `name`, `login`, `right` and `active`.
This keyword need Admin privileges.
Example:
`{'name': 'Peter Parker', 'login': 'spider', 'right': 'user', 'active': True}`
'''
return self.connection.get_user_all(self.session)
def get_user_details(self, user_id=None):
'''Returs the user details of the given user_id or if None the own user data.'''
return self.connection.get_user(self.session, user_id)
def get_user_id(self, login):
'''Returns the user_id based on login.'''
return self.connection.get_user_id(self.session, login)
def get_username(self, user_id=None):
'''Returns the users full name of the given user_id or if None the own user data.'''
return self.connection.get_user_name(self.session, user_id)
def get_server_version(self):
return self.connection.get_version(self.session)
二、为什么选择 Robot Framework
Robot Framework支持插件化的架构,可以使用许多内置库和第三方库来扩展功能,例如SeleniumLibrary用于Web自动化测试,RequestsLibrary用于API测试等。
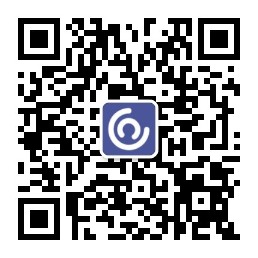
1. Robot Framework 的常见用法
Robot Framework可用于各种自动化测试任务,包括:
- Web应用测试
- API测试
- 数据库测试
- 移动应用测试
- 自动化任务和流程
- 持续集成和部署
2. 常见关键字库
Robot Framework有广泛的关键字库可供使用,以下是一些常见的关键字库:
- SeleniumLibrary:用于Web应用测试,包括页面操作、表单填写、页面验证等。
- RequestsLibrary:用于发送HTTP请求,进行API测试。
- DatabaseLibrary:用于数据库测试,支持各种数据库操作。
- SSHLibrary:用于通过SSH执行命令和操作远程服务器。
- ExcelLibrary:用于读写Excel文件。
- DateTimeLibrary:用于日期和时间相关操作。
3. 技术架构和原理
Robot Framework的技术架构基于关键字驱动的测试方法。它使用Python语言编写,通过解析测试用例文件和执行测试步骤来实现自动化测试。
Robot Framework的核心原理如下:
- 测试用例文件(通常使用
.robot
扩展名)包含测试用例、关键字定义和设置。 - 测试执行器(test runner)读取并解析测试用例文件。
- 测试执行器调用相应的关键字库(如SeleniumLibrary、RequestsLibrary等)执行测试步骤。
- 关键字库提供了一组关键字(如打开浏览器、点击按钮等)来操作被测试的系统。
- 执行结果被记录下来,并生成报告和日志文件。
三、怎么使用 Robot Framework
1. Robot Framework的安装方法
1. 安装Python
Robot Framework是基于Python的,所以首先需要安装Python。你可以从Python官方网站(https://www.python.org)下载适合你操作系统的Python安装程序,并按照官方指南进行安装。
2. 安装Robot Framework
一旦Python安装完成,你可以使用Python的包管理工具pip来安装Robot Framework。打开终端或命令提示符窗口,并执行以下命令:
pip install robotframework
这将下载并安装最新版本的Robot Framework及其依赖项。
3. 安装额外的关键字库
根据你的测试需求,你可能需要安装额外的关键字库。例如,如果你需要进行Web应用测试,可以安装SeleniumLibrary。以安装SeleniumLibrary为例,执行以下命令:
pip install robotframework-seleniumlibrary
类似地,你可以使用pip命令安装其他关键字库,具体取决于你的需求。
4. 验证安装
安装完成后,你可以验证Robot Framework是否成功安装。在终端或命令提示符窗口中执行以下命令:
robot --version
如果成功安装,它将显示安装的Robot Framework的版本号。
2. 自动化测试用例常见实例代码
示例1:Web应用测试
*** Settings ***
Library SeleniumLibrary
*** Test Cases ***
Open Browser and Verify Title
Open Browser https://www.example.com chrome
Title Should Be Example Domain
Close Browser
示例2:API测试
*** Settings ***
Library RequestsLibrary
*** Test Cases ***
Get User Details
Create Session Example API https://api.example.com
${response} Get Request Example API /users/123
Should Be Equal As Strings ${response.status_code} 200
${user_details} Set Variable ${response.json()}
Log ${user_details}
Delete All Sessions
示例3:数据库测试
*** Settings ***
Library DatabaseLibrary
Library Collections
*** Test Cases ***
Verify User in Database
Connect To Database pymysql mydatabase myusername mypassword
@{query_result} Query SELECT * FROM users WHERE id = '123'
Should Contain ${query_result} username=John
Disconnect From Database
示例4:SSH操作
*** Settings ***
Library SSHLibrary
*** Test Cases ***
Execute Command on Remote Server
Open Connection 192.168.1.100 username=myusername password=mypassword
${output} Execute Command ls /path/to/directory
Should Contain ${output} myfile.txt
Close Connection
示例5:Excel操作
*** Settings ***
Library ExcelLibrary
*** Test Cases ***
Read Data From Excel
Open Excel path/to/excel/file.xlsx
${cell_value} Read Cell Data By Name Sheet1 A1
Log ${cell_value}
Close Excel
四、相关链接
RobotFramework基金会成员