获取示例
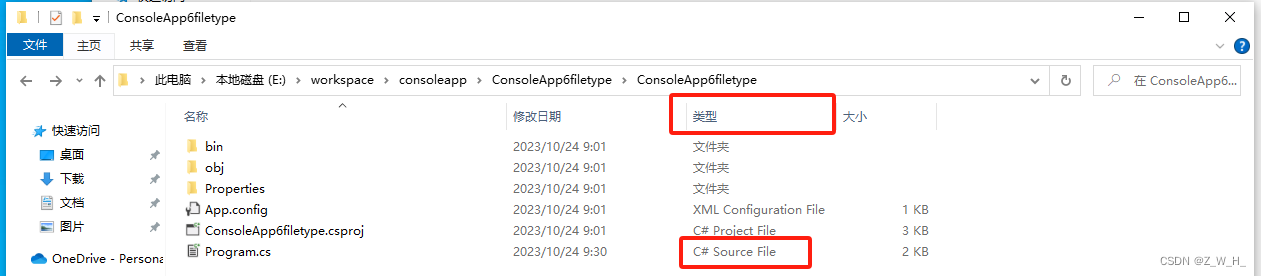
代码
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp6filetype
{
internal class Program
{
static void Main(string[] args)
{
string filePath = Path.GetFullPath("..//..");
//Console.WriteLine(filePath);
string fileFullPath = Path.Combine(filePath, "Program.cs");
string typeName = GetFileTypeName(fileFullPath);
Console.WriteLine(typeName);
Console.ReadKey();
}
//pszPath指向包含路径和文件名的最大长度MAX_PATH 以 null 结尾的字符串的指针。 绝对路径和相对路径均有效。
//dwFileAttributes 一个或多个 文件属性标志 的组合, (FILE_ATTRIBUTE_ Winnt.h) 中定义的值。 如果 uFlags 不包含 SHGFI_USEFILEATTRIBUTES 标志,则忽略此参数。
//psfi指向 SHFILEINFO 结构的指针,用于接收文件信息。
//cbFileInfo psfi 参数指向的 SHFILEINFO 结构的大小(以字节为单位)。
//uFlags指定要检索的文件信息的标志。 此参数可以是以下值的组合。
//https://learn.microsoft.com/zh-cn/windows/win32/api/shellapi/nf-shellapi-shgetfileinfoa?redirectedfrom=MSDN
[DllImport("shell32.dll", CharSet = CharSet.Auto)]
public static extern int SHGetFileInfo(string pszPath, uint dwFileAttributes, out SHFILEINFO psfi, uint cbFileInfo, uint uFlags);
//https://learn.microsoft.com/zh-cn/windows/win32/api/shellapi/ns-shellapi-shfileinfoa
[StructLayout(LayoutKind.Sequential, CharSet = CharSet.Auto)]
public struct SHFILEINFO
{
public IntPtr hIcon;//表示文件的图标的句柄。
public int iIcon;//系统映像列表中的图标图像的索引。
public uint dwAttributes;//一个值数组,指示文件对象的属性。
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 260)]
public string szDisplayName;//一个字符串,其中包含文件在 Windows Shell 中显示的名称,或包含表示该文件的图标的文件的路径和文件名。
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 80)]
public string szTypeName;//描述文件类型的字符串。
}
//检索描述文件类型的字符串。 字符串将复制到 psfi 中指定的结构的 szTypeName 成员。
public const uint SHGFI_TYPENAME = 0x000000400;
//未设置其他属性的文件。 此属性仅在单独使用时才有效。
//https://learn.microsoft.com/zh-cn/windows/win32/fileio/file-attribute-constants
public const uint FILE_ATTRIBUTE_NORMAL = 0x00000080;
static string GetFileTypeName(string filePath)
{
SHFILEINFO shfi = new SHFILEINFO();
int ret = SHGetFileInfo(filePath, FILE_ATTRIBUTE_NORMAL, out shfi, (uint)Marshal.SizeOf(shfi),
SHGFI_TYPENAME);
if (ret != 0)
return shfi.szTypeName;
else
return string.Empty;
}
}
}
结果

参考文献
https://www.cnblogs.com/log9527blog/p/17723109.html