前面讲过 选择排序 是基于元素比较的,现在这个是基于相邻元素比较的算法
算法描述:
使用从小到大的排序策略.
从最后一个元素开始,按照从后向前的方向,相邻元素之间进行比较,如果发现后一个元素比前一个元素小,则交换,直至数组结束,这样就可以找出最小的元素,放在第一个位置.
然后再次重复上面的策略,在剩下的元素中找到最小的元素放在第二个位置.
直至整个循环结束
算法实现为:
public class BubbleSort { public static void main(String[] args) { int[] nums = new int[]{1, 15, 3, 78, 34, 23, 46, 2, 8, 34, 57}; System.out.println("before insert sort "+ Arrays.toString(nums)); sort(nums); System.out.println("after insert sort "+Arrays.toString(nums)); } public static void sort(int[] arrays) { int length = arrays.length; for(int i=0; i< length; i++) { for(int j = length -1; j>0; j--) { if (arrays[j] < arrays[j - 1]) { int temp = arrays[j]; arrays[j] = arrays[j-1]; arrays[j-1] = temp; } } } } }
这个过程就像水里的气泡上浮一样,每次外循环结束都上浮一个最小的元素(剩余元素中的最小元素),这样直至整个数组结束,所有的元素都变得有序了
这里有个小的优化点,就是内循环的循环次数,并不需要n次,因为每次上浮都会使数组的前 i 个元素变得有序
优化
扫描二维码关注公众号,回复:
169830 查看本文章
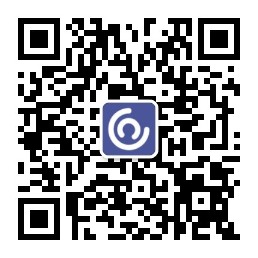
public static void sort(int[] arrays) { int length = arrays.length; for(int i=0; i< length; i++) { for(int j = length -1; j>i; j--) { if (arrays[j] < arrays[j - 1]) { int temp = arrays[j]; arrays[j] = arrays[j-1]; arrays[j-1] = temp; } } } }
优化点为内循环的循环次数,由n次,减小到了 n-i 次,条件由 j>0 改为 j>i
这里面有个点需要说明下,我们再插入排序中由这样的写法:
for(int j=i ; j> 0 && arrays[j] < arrays[j-1] ; j--) { int temp = arrays[j]; arrays[j] = arrays[j - 1]; arrays[j-1] = temp; }
我们将比较条件放在了 for()循环的循环体中,在冒泡排序中,我们可以这样写么?比如:
for (int j = length - 1; j > i && arrays[j] < arrays[j - 1]; j--) { int temp = arrays[j]; arrays[j] = arrays[j - 1]; arrays[j - 1] = temp; }
答案是肯定不行,如果你这样写了,你一定不能得到正确的排序结果,
对于插入排序来说,他的条件是在已经排好顺序的数组中找个一个合适的位置插入,比较的过程是发生在相邻元素之间的,只要待插入的元素比有序数组中的元素大,则立即结束循环,然后插入制定位置.
但是对于冒泡排序和选择排序来说,它必须不断的比较直至数组结束,因为他是在未排序的数组中比较的,必须遍历所有的未排序数组元素才行
下一篇: 插入排序