Android开发学习【Day01】
Android onCreate 详解
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
此处进行简要介绍,详细请点击Android onCreate 详解
一、 super.onCreate(savedInstanceState)
其中super.onCreate(savedInstanceState)的作用是调用其父类Activity的onCreate方法来实现对界面的图画绘制工作。
在实现自己定义的Activity子类的onCreate方法时一定要记得调用该方法,以确保能够绘制界面。
二、setContentView(R.layout.caculator_layout)
作用:加载一个界面。
该方法中传入的参数是”R.layout.caculator_layout“,其含义为R.java类中静态内部类layout的静态常量caculator_layout的值,
而该值是一个指向res目录下的layout子目录下的caculator_layout.xml文件的标识符。因此代表着显示caculator_layout.xml所定义的画面。
简单控件
文本显示
设置文本内容方式
设置文本内容有两种方式:
·在 XML 文件中通过属性 android:text 设置文本
<resources>
<string name="app_name">Study01</string>
<string name="hello">你好,世界!</string>
</resources>
android:text="Hello World!"//直接设置
or
android:text="@string/hello" //引用string里面设置的常量
·在 Java 代码中调用文本视图对象的 setText
<TextView
android:id="@+id/tv_hello"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello"/>
通过java代码设置文本内容
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_text_view);
TextView tv_hello = findViewById(R.id.tv_hello);
tv_hello.setText("你好世界");
}
设置文本的大小
在 Java 代码中调用 setTextSize 方法,即可指定文本大小。
在 XML 文件中则通过属性 android:textSize 指定文本大小,此时需要指定字号单位。
px:它是手机屏幕的最小显示单位,与设备的显示屏有关。
dp:它是与设备无关的显示单位,只与屏幕的尺寸有关。
sp:它专门用来设置字体大小,在系统设置中可以调整字体大小/(追随系统)。
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello"
android:textSize="30px"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello"
android:textSize="30dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello"
android:textSize="30sp"/>
设置文本的颜色
在 Java 代码中调用 setTextColor 方法即可设置文本颜色,具体色值可从 Color 类
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_color_view);
TextView tv_hello_color = findViewById(R.id.tv_hello_color);
tv_hello_color.setTextColor(Color.GREEN);
}
也次从color.xml中获取 例如:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="purple_200">#FFBB86FC</color>
<color name="purple_500">#FF6200EE</color>
<color name="purple_700">#FF3700B3</color>
<color name="teal_200">#FF03DAC5</color>
<color name="teal_700">#FF018786</color>
<color name="black">#FF000000</color>
<color name="white">#FFFFFFFF</color>
</resources>
<TextView
android:id="@+id/tv_hello_color"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello"
android:textColor="@color/black"/>
设置视图的宽高
直接设置
视图宽度通过属性android:layout_width表达,视图高度通过属性android:layout_height表达,宽高的取值主要有下列三种:
match_parent:表示与上级视图保持一致。
wrap_content:表示与内容自适应。
以dp为单位的具体尺寸。
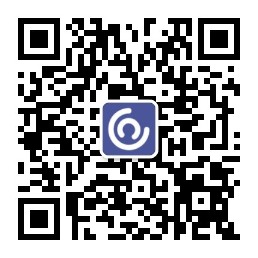
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="设置视图的宽高"
android:textSize="10dp"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="设置视图的宽高"
android:textSize="10dp"/>
在代码中设置视图宽高
首先确保XML中的宽高属性值为wrap_content,接着打开该页面对应的Java代码,依序执行以下三个步骤:
调用控件对象的getLayoutParams方法,获取该控件的布局参数。
布局参数的width属性表示宽度,height属性表示高度,修改这两个属性值。
调用控件对象的setLayoutParams方法,填入修改后的布局参数使之生效。
public class ViewBorderActivity extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_view_border);
TextView tv_code = findViewById(R.id.tv_code);
//获取tv_code的布局参数(含宽度和高度)
ViewGroup.LayoutParams params = tv_code.getLayoutParams();
//修改布局参数,注意默认px单位
params.height=300;
params.width=300;
tv_code.setLayoutParams(params);
}
}
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="设置视图的宽高"
android:textSize="10dp"/>
<TextView
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="设置视图的宽高"
android:textSize="10dp"/>
<TextView
android:id="@+id/tv_code"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:background="#00ffff"
android:text="通过代码设置视图的宽高"
android:textSize="10dp"/>
设置视图间距
采用layout_margin属性,它指定了当前视图与周围平级视图之间的距离/(外边距)。包括layout_margin、layout_marginLeft、layout_marginTop、layout_marginRight、layout_marginBottom
采用padding属性,它指定了当前视图与内部下级视图之间的距离/(内边距)。包括padding、paddingLeft、paddingTop、paddingRight、paddingBottom
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".ViewMarginActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="60dp"
android:background="@color/teal_200"
android:orientation="vertical">
<View
android:layout_width="match_parent"
android:layout_height="300dp"
android:layout_margin="20dp"
android:background="@color/teal_700"/>
<View
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white"/>
</LinearLayout>
</LinearLayout>
设置视图的对齐方式
设置视图的对齐方式有两种途径:
采用layout_gravity属性,它指定了当前视图相对于上级视图的对齐方式。
采用gravity属性,它指定了下级视图相对于当前视图的对齐方式。
layout_gravity与gravity的取值包括:left、top、right、bottom,还可以用竖线连接各
取值,例如“left|top”表示即靠左又靠上,也就是朝左上角对齐。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="400dp"
android:orientation="horizontal">
<LinearLayout
android:layout_width="0dp"
android:layout_height="200dp"
android:layout_weight="1"
android:background="@color/red"
android:layout_margin="10dp"
android:padding="10dp"
android:layout_gravity="bottom"
android:gravity="right|top">
<View
android:layout_width="100dp"
android:layout_height="100dp"
android:background="@color/teal_200"/>
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="200dp"
android:layout_weight="1"
android:background="@color/red"
android:layout_margin="10dp"
android:padding="10dp"
android:layout_gravity="top"
android:gravity="left|bottom">
<View
android:layout_width="100dp"
android:layout_height="100dp"
android:background="@color/teal_200" />
</LinearLayout>
</LinearLayout>
线性布局LinearLayout
线性布局内部的各视图的两种排列方式
orientation属性值为horizontal时,内部视图在水平方向从左往右排列。
orientation属性值为vertical时,内部视图在垂直方向从上往下排列。
如果不指定orientation属性,则LinearLayout默认水平方向排列。
线性布局的权重
线性布局的权重概念,指的是线性布局的下级视图各自拥有多大比例的宽高。
权重属性名叫layout_weight,但该属性不在LinearLayout节点设置,而在线性布局的直
接下级视图设置,表示该下级视图占据的宽高比例。
layout_width填0dp时,layout_weight表示水平方向的宽度比例。
layout_height填0dp时,layout_weight表示垂直方向的高度比例。
layout_weight所代表的值->相当于占总和的百分比
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:textSize="17sp"
android:background="@color/teal_200"
android:text="水平方向第一个单元"/>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:textSize="17sp"
android:background="@color/teal_200"
android:text="水平方向第二个单元"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:textSize="17sp"
android:background="@color/teal_700"
android:text="竖直方向第一个单元"/>
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:textSize="17sp"
android:background="@color/teal_700"
android:text="竖直方向第二个单元"/>
</LinearLayout>
</LinearLayout>
相对布局RelativeLayout
相对布局的下级视图位置由其他视图决定。用于确定下级视图位置的参照物分两种:
与该视图自身平级的视图;
该视图的上级视图(也就是它归属的RelativeLayout)
如果不设定下级视图的参照物,那么下级视图默认显示在RelativeLayout内部的左上角。
相对位置的取值
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="400dp">
<TextView
android:id="@+id/pos_mid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="中间"
android:layout_centerInParent="true"
android:textColor="@color/black"
android:textSize="17sp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="水平居中"
android:layout_centerHorizontal="true"
android:textColor="@color/black"
android:textSize="17sp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="垂直居中"
android:textSize="17sp"
android:textColor="@color/black"
android:layout_centerVertical="true"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="左侧对齐"
android:textSize="17sp"
android:textColor="@color/black"
android:layout_alignParentLeft="true"/>
<TextView
android:id="@+id/pos_right"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="右侧对齐"
android:textSize="17sp"
android:textColor="@color/black"
android:layout_alignParentRight="true"/>
<TextView
android:id="@+id/bottom"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="底部"
android:textSize="17sp"
android:textColor="@color/black"
android:layout_alignParentBottom="true"/>
<TextView
android:id="@+id/pos_mid_right"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="右侧中间"
android:layout_centerVertical="true"
android:layout_alignRight="@id/pos_right"
android:textSize="17sp"
android:textColor="@color/black" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="底部中间"
android:layout_alignLeft="@id/pos_mid"
android:layout_alignParentBottom="true"
android:textSize="17sp"
android:textColor="@color/black" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="底部右侧"
android:layout_alignLeft="@id/pos_mid_right"
android:layout_alignParentBottom="true"
android:textSize="17sp"
android:textColor="@color/black" />
</RelativeLayout>
网格布局GridLayout
网格布局支持多行多列的表格排列。
网格布局默认从左往右、从上到下排列,它新增了两个属性:
columnCount属性,它指定了网格的列数,即每行能放多少个视图;
rowCount属性,它指定了网格的行数,即每列能放多少个视图;
网格布局的权重
使用app:layout_columnWeight 和app:layout_rowWeight 设置权重
例如:
<?xml version="1.0" encoding="utf-8"?>
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="2"
android:rowCount="2">
<TextView
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_columnWeight="1"
android:text="浅红色"
android:gravity="center"
android:textColor="@color/black"
android:background="#ffcccc"
android:textSize="17sp"/>
<TextView
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_columnWeight="1"
android:gravity="center"
android:text="橙色"
android:textColor="@color/black"
android:background="#ffaa00"
android:textSize="17sp"/>
<TextView
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_columnWeight="1"
android:gravity="center"
android:text="绿色"
android:textColor="@color/black"
android:background="#00ff00"
android:textSize="17sp"/>
<TextView
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_columnWeight="1"
android:layout_colWeight="1"
android:gravity="center"
android:text="深紫色"
android:textColor="@color/black"
android:background="#660066"
android:textSize="17sp"/>
</GridLayout>
滚动视图ScrollView
两种滚动视图
ScrollView,它是垂直方向的滚动视图;垂直方向滚动时,layout_width属性值设置为match_p
arent,layout_height属性值设置为wrap_content。
HorizontalScrollView,它是水平方向的滚动视图;水平方向滚动时,layout_width属性值设置
为wrap_content,layout_height属性值设置为match_parent。
此处建议在虚拟机or实体机运行体验(个人表示体验感很爽)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<HorizontalScrollView
android:layout_width="wrap_content"
android:layout_height="200dp">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:orientation="horizontal">
<View
android:layout_width="300dp"
android:layout_height="match_parent"
android:background="@color/teal_200"/>
<View
android:layout_width="300dp"
android:layout_height="match_parent"
android:background="@color/teal_700"/>
</LinearLayout>
</HorizontalScrollView>
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="400dp"
android:text="1024节日快乐!"
android:textColor="@color/black"
android:gravity="center"
android:textSize="17sp"
android:background="@color/red"/>
<View
android:layout_width="match_parent"
android:layout_height="400dp"
android:background="@color/purple_200"/>
</LinearLayout>
</ScrollView>
</LinearLayout>