相关文章链接:
1.5 ProgressBar
常用属性 |
描述 |
android:max |
进度条的最大值 |
android:progress |
进度条已完成进度值 |
android:indeterminate |
如果设置为true,则进度条不精确显示进度 |
style=“?android:attr/progressBarStyleHorizontal" |
水平进度条 |
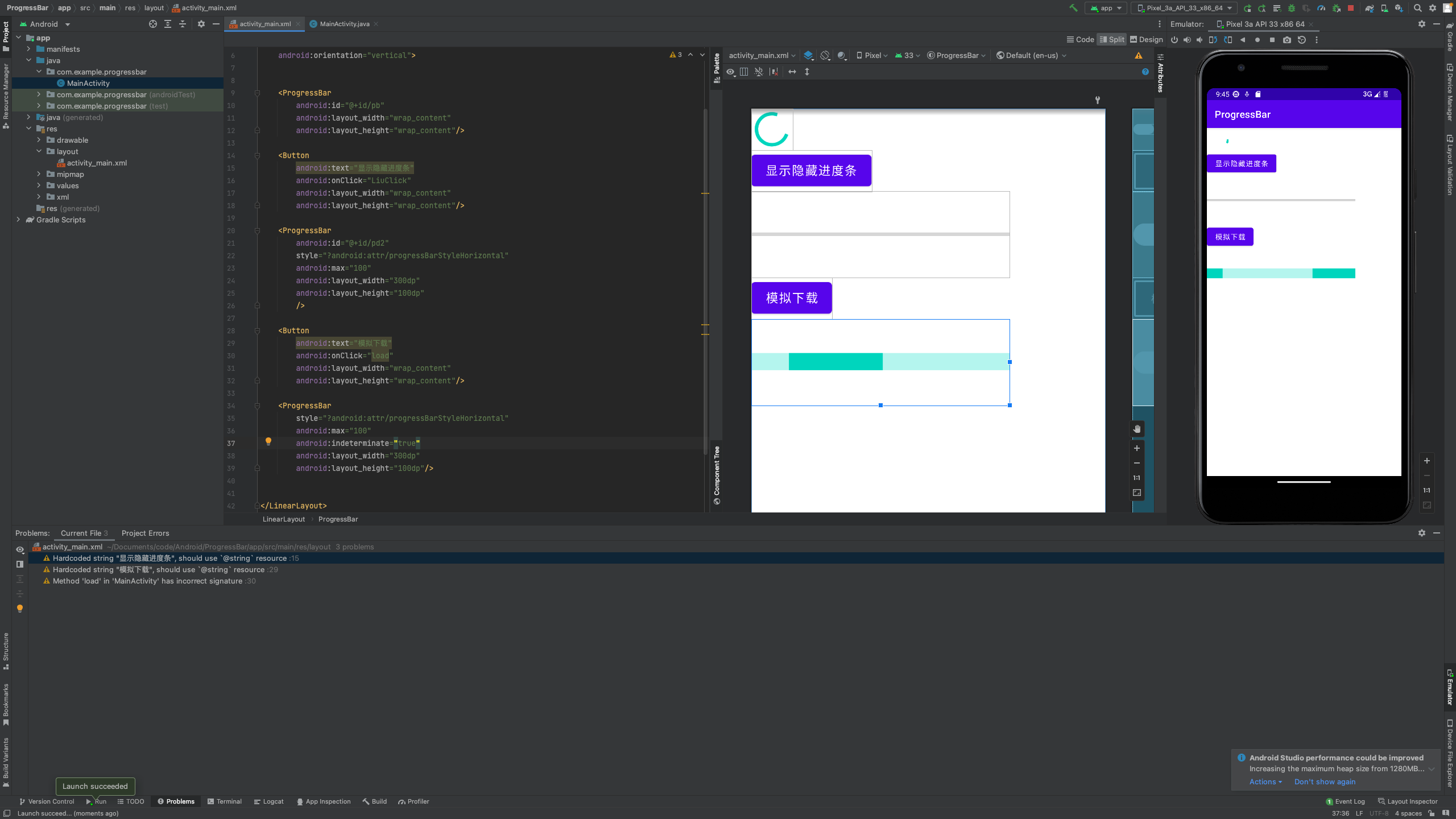
package com.example.progressbar;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ProgressBar;
public class MainActivity extends AppCompatActivity {
private ProgressBar progressBar;
private ProgressBar progressBar2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
progressBar = findViewById(R.id.pb);
progressBar2 = findViewById(R.id.pd2);
}
public void LiuClick(View view){
if (progressBar.getVisibility() == View.GONE){
progressBar.setVisibility(View.VISIBLE);
}
else {
progressBar.setVisibility(View.GONE);
}
}
public void load(){
int progress = progressBar2.getProgress();
progress += 10;
progressBar2.setProgress(progress);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ProgressBar
android:id="@+id/pb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="显示隐藏进度条"
android:onClick="LiuClick"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<ProgressBar
android:id="@+id/pd2"
style="?android:attr/progressBarStyleHorizontal"
android:max="100"
android:layout_width="300dp"
android:layout_height="100dp"
/>
<Button
android:text="模拟下载"
android:onClick="load"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<ProgressBar
style="?android:attr/progressBarStyleHorizontal"
android:max="100"
android:indeterminate="true"
android:layout_width="300dp"
android:layout_height="100dp"/>
</LinearLayout>
1.6 Notification
创建一个NotificationManager
NotificationManager类是一个通知管理器类,这个对象是由系统维护的服务,是以单例模式的方法获得,所以一般并不直接实力化这个对象。在Activity中,可以使用Activity.getSystemService(String)方法获取NotificationManager对象,Activity.getSystemService(String)方法可以通过Android系统服务的句柄,返回对应的对象。在这里需要返回NotificationManager,所以直接传递Context.NOTIFICATION_SERVICE即可。
2、使用Builder构造器来创建Notification对象
使用NotificationCompat类的Builder构造器来创建Notification对象,可以保证程序在所有的版本上都能正常工作。Android8.0新增了通知渠道这个概念,如果没有设置,则通知无法在Android8.0的机器上显示。
通知重要程度设置 |
描述 |
IMPORTANCE_NONE |
关闭通知 |
IMPORTANCE_MIN |
开启通知,不会弹出,没有提示音,状态栏中无显示 |
IMPORTANCE_LOW |
开启通知,不会弹出,没有提示音,状态栏中显示 |
IMPORTANCE_DEFAULT |
开启通知,不会弹出,发出提示音,状态栏中显示 |
IMPORTANCE_HIGH |
开启通知,会弹出,发出提示音,状态栏中显示 |
常见属性 |
说明 |
setContentTitle(String string) |
设置标题 |
setContentTile(String sring) |
设置文本内容 |
setSmallIcon(int icon) |
设置小图标 |
setLargeIcon(Bitmap icon) |
设置通知的大图标 |
setColor(int argb) |
设置小图标的颜色 |
setAutoCancel(boolean boolean) |
设置点击通知后自动清除通知 |
setContentIntent(pendingIntent intent) |
设置点击通知后的跳转意图 |
setWhen(long when) |
设置通知被创建的时间 |
注意:图片不能带颜色
1.7 Toolbar
常见属性:
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="#ffff00"
app:navigation="@drawable/ic_baseline_arrow_back_24"
app:title="主标题"
app:titleTextColor="#ff0000"
app:titleMarginStart="90dp"
app:subtitle="子标题"
app:subtitleTextColor="#00ffff"
app:logo="@mipmap/ic_launcher"
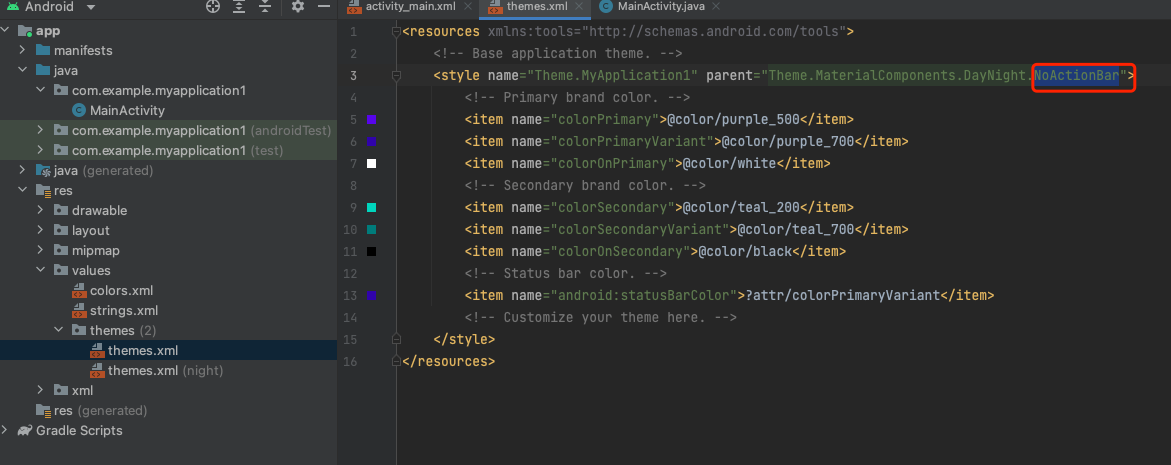
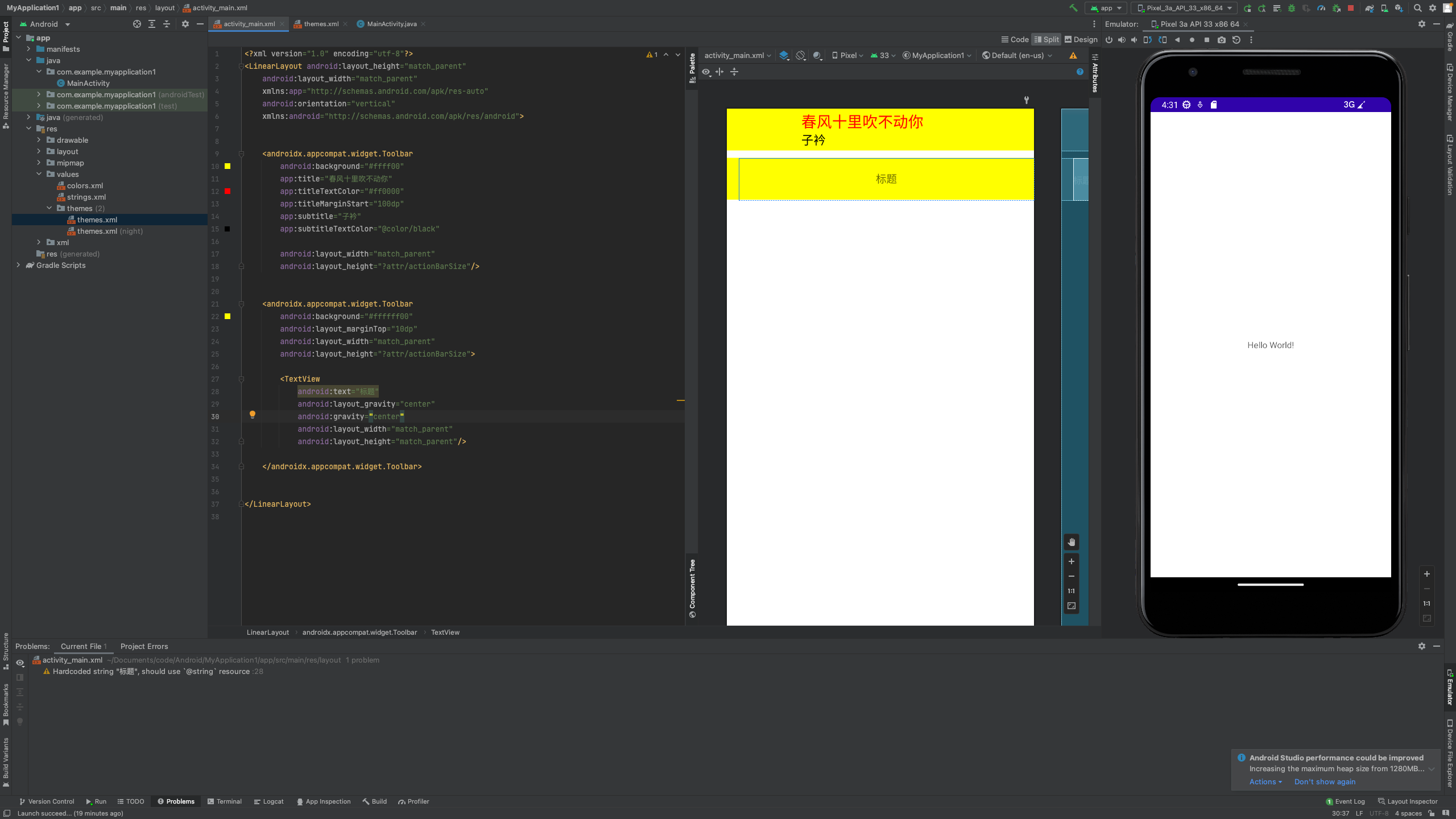
1.8 AlertDialog
实现方式
属性 |
说明 |
AlertDialog.Builder builder = new AlertDialog.Builder(context) |
构建Dialog的各种参数 |
Builder.setIcon(int iconId) |
添加ICON |
Builder.setTitle(CharSequence title) |
添加标题 |
Builder.setMessage(CharSequence message) |
添加消息 |
Builder.setView(View view) |
设置自定义布局 |
Builder.create() |
创建Dialog |
Builder.show() |
显示对话框 |
setPositiveButton |
确定按钮 |
setNefativeButton |
取消按钮 |
setNeutralButton |
中间按钮 |
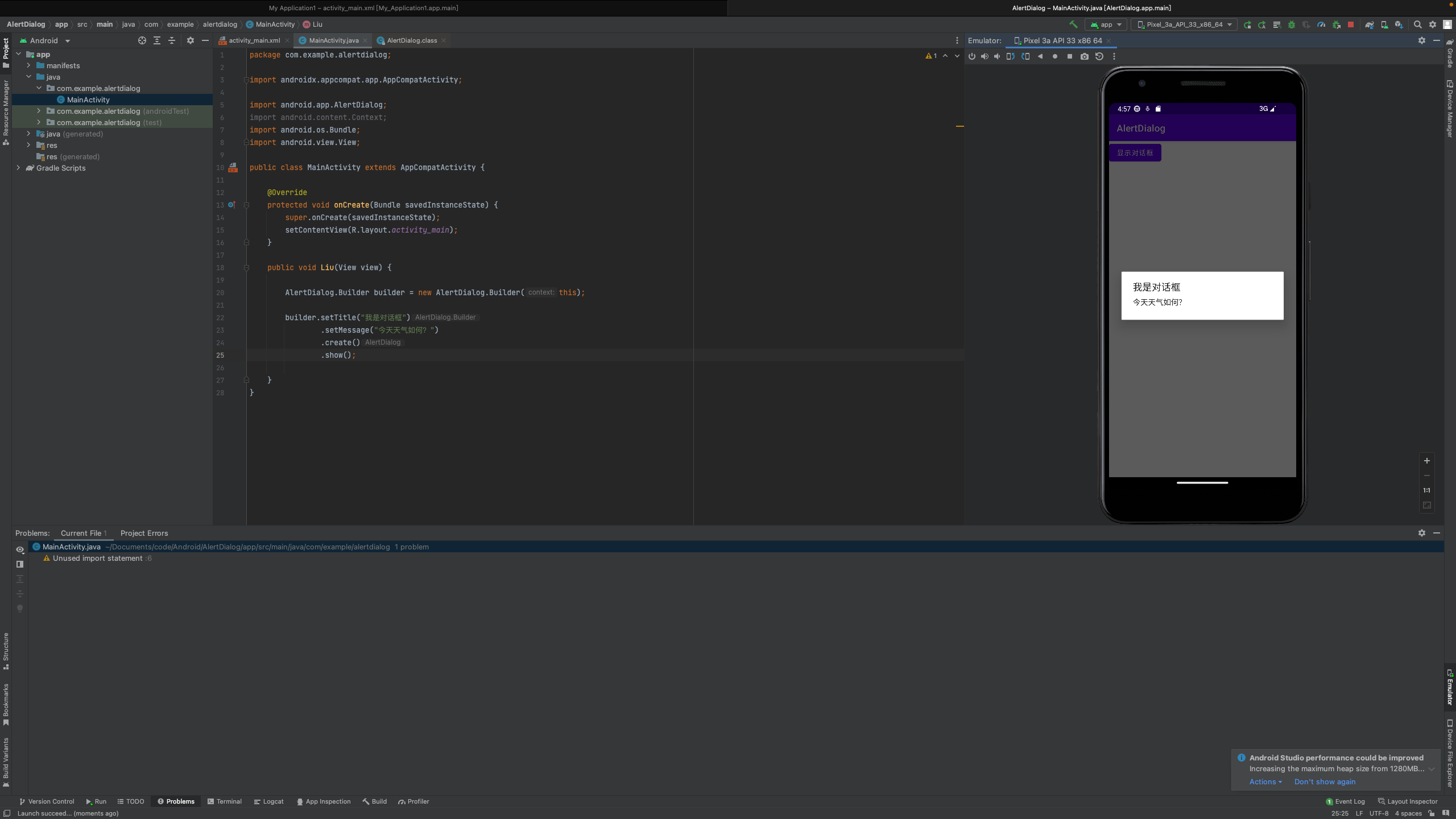
package com.example.alertdialog;
import androidx.appcompat.app.AppCompatActivity;
import android.app.AlertDialog;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void Liu(View view) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("我是对话框")
.setMessage("今天天气如何?")
.create()
.show();
}
}
注意:builder中其他顺序无所谓,create()必须放到数第二,show()必须放最后。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:orientation="vertical">
<Button
android:text="显示对话框"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="Liu"
/>
</LinearLayout>
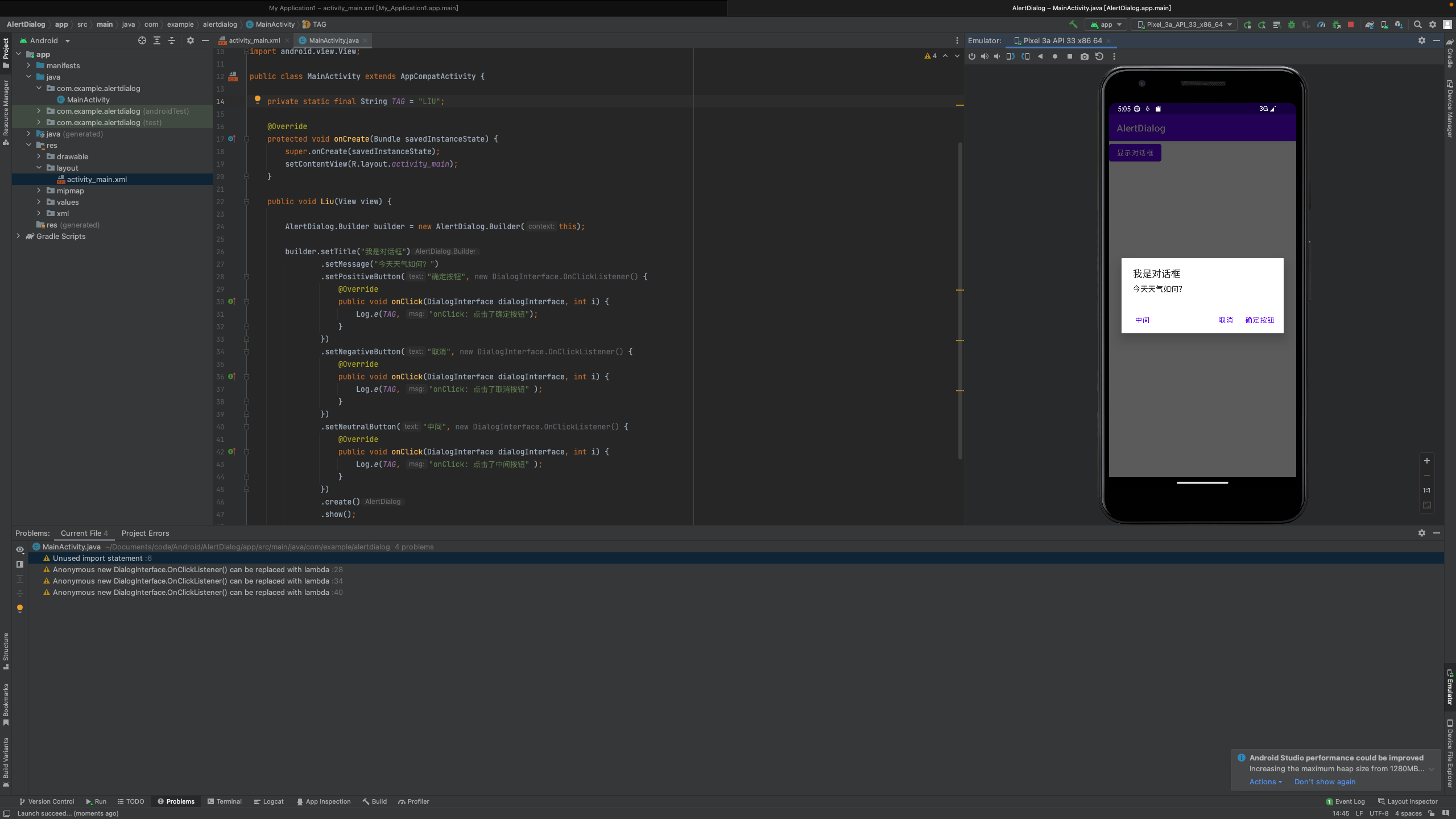
package com.example.alertdialog;
import androidx.appcompat.app.AppCompatActivity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "LIU";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void Liu(View view) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("我是对话框")
.setMessage("今天天气如何?")
.setPositiveButton("确定按钮", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Log.e(TAG, "onClick: 点击了确定按钮");
}
})
.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Log.e(TAG, "onClick: 点击了取消按钮" );
}
})
.setNeutralButton("中间", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Log.e(TAG, "onClick: 点击了中间按钮" );
}
})
.create()
.show();
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:orientation="vertical">
<Button
android:text="显示对话框"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="Liu"
/>
</LinearLayout>
1.9 PopupWindow
常用方法 |
说明 |
setContentView(View contentView) |
设置PopupWindow显示的View |
showAsDropDown(View anchor) |
相对某个控件的位置(正左下方),无偏移 |
showAsDropDown(View anchor,int xoff, int yoff) |
相对某个控件的位置,有偏移 |
setFocusable(boolean focusable) |
设置是否获取焦点 |
setBackgroundDrawable(Drawable background) |
设置背景 |
dismiss() |
关闭弹窗 |
setAnimationStyle(int animationStyle) |
设置加载动画 |
setTouchable(boolean touchable) |
设置触摸功能 |
setOutsideTouchable(boolean touchable) |
设置PoupWindow外面的触摸功能 |