14.6 UART(通用异步收发器)设备驱动
一个特定的 UART 设备驱动完全可以定义tty_driver 并实现 tty_operations 其中的成员函数,但Linux 已经在serial_core.c 中实现UART 设备的通用 tty 驱动层(串口核心层),UART 驱动的主要任务演变成实现
serial-core.c 中定义的一组 uart_xxx 接口。如图 14.4 所示。
14.4 串口核心层
serial_core.c 串口核心层完全可以被当作 tty 设备驱动的实例,实现了 UART设备的 tty 驱动。这体现了软件工程中设备驱动的分层思想。
串口核心层为串口设备驱动提供了如下 3 个结构体。
1.uart_driver
uart_driver 包含串口设备的驱动名、设备名、设备号等信息,uart_driver封装了 tty_driver,使得底层的 UART 驱动无需关心 tty_driver,其定义如代码清单 14.13 所示。
代码清单 14.13 uart_driver 结构体
include/linux/serial_core.h
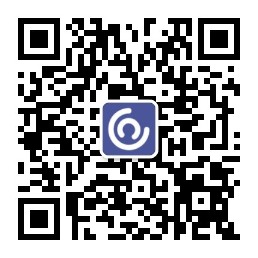
struct uart_driver {
struct module *owner;
const char *driver_name;/* 驱动名 */
const char *dev_name;/* 设备名 */
int major;/* 主设备号 */
int minor;/* 次设备号 */
int nr;
struct console *cons;
/** these are private; the low level driver should not
* touch these; they should be initialised to NULL
*/
struct uart_state *state;
struct tty_driver *tty_driver;
};
注册/注销 uart_driver,使用如下接口:
int uart_register_driver(struct uart_driver *drv);
void uart_unregister_driver(struct uart_driver *drv);
uart_register_driver()和 uart_unregister_driver()中分别包含了 tty_register_driver()和tty_unregister_driver()的操作,如代码清单 14.14 所示。
代码清单 14.14 uart_register_driver()和 uart_unregister_driver()函数
drivers/serial/serial_core.c
int uart_register_driver(struct uart_driver *drv)
{
struct tty_driver *normal = NULL;
int i, retval;
BUG_ON(drv->state);
/*
* Maybe we should be using a slab cache for this, especially if
* we have a large number of ports to handle.
*/
drv->state = kzalloc(sizeof(struct uart_state) * drv->nr, GFP_KERNEL);
retval = -ENOMEM;
if (!drv->state)
goto out;
normal = alloc_tty_driver(drv->nr);
if (!normal)
goto out;
drv->tty_driver = normal;
normal->owner = drv->owner;
normal->driver_name = drv->driver_name;
normal->name = drv->dev_name;
normal->major = drv->major;
normal->minor_start = drv->minor;
normal->type = TTY_DRIVER_TYPE_SERIAL;
normal->subtype = SERIAL_TYPE_NORMAL;
normal->init_termios = tty_std_termios;
normal->init_termios.c_cflag = B9600 | CS8 | CREAD | HUPCL | CLOCAL;
normal->init_termios.c_ispeed = normal->init_termios.c_ospeed = 9600;
normal->flags = TTY_DRIVER_REAL_RAW | TTY_DRIVER_DYNAMIC_DEV;
normal->driver_state = drv;
tty_set_operations(normal, &uart_ops);
/*
* Initialise the UART state(s).
*/
for (i = 0; i < drv->nr; i++) {
struct uart_state *state = drv->state + i;
state->close_delay = 500; /* .5 seconds */
state->closing_wait = 30000; /* 30 seconds */
mutex_init(&state->mutex);
}
retval = tty_register_driver(normal);
out:
if (retval < 0) {
put_tty_driver(normal);
kfree(drv->state);
}
return retval;
}
void uart_unregister_driver(struct uart_driver *drv)
{
struct tty_driver *p = drv->tty_driver;
tty_unregister_driver(p);
put_tty_driver(p);
kfree(drv->state);
drv->tty_driver = NULL;
}
2.uart_port
uart_port 用于描述一个 UART 端口(直接对应于一个串口)的 I/O 端口或 I/O 内存地址、FIFO大小、端口类型等信息,其定义如代码清单 14.15。
代码清单 14.15 uart_port 结构体
include/linux/serial_core.h
struct uart_port {
spinlock_t lock; /* port lock */
unsigned int iobase; /* in/out[bwl] */
unsigned char __iomem *membase; /* read/write[bwl] */
unsigned int irq; /* irq number */
unsigned int uartclk; /* base uart clock */
unsigned int fifosize; /* tx fifo size */
unsigned char x_char; /* xon/xoff char */
unsigned char regshift; /* reg offset shift */
unsigned char iotype; /* io access style */
unsigned char unused1;
#define UPIO_PORT (0)
#define UPIO_HUB6 (1)
#define UPIO_MEM (2)
#define UPIO_MEM32 (3)
#define UPIO_AU (4) /* Au1x00 type IO */
#define UPIO_TSI (5) /* Tsi108/109 type IO */
#define UPIO_DWAPB (6) /* DesignWare APB UART */
#define UPIO_RM9000 (7) /* RM9000 type IO */
unsigned int read_status_mask; /* driver specific */
unsigned int ignore_status_mask; /* driver specific */
struct uart_info *info; /* pointer to parent info */
struct uart_icount icount; /* statistics */
struct console *cons; /* struct console, if any */
#ifdef CONFIG_SERIAL_CORE_CONSOLE
unsigned long sysrq; /* sysrq timeout */
#endif
upf_t flags;
#define UPF_FOURPORT ((__force upf_t) (1 << 1))
#define UPF_SAK ((__force upf_t) (1 << 2))
#define UPF_SPD_MASK ((__force upf_t) (0x1030))
#define UPF_SPD_HI ((__force upf_t) (0x0010))
#define UPF_SPD_VHI ((__force upf_t) (0x0020))
#define UPF_SPD_CUST ((__force upf_t) (0x0030))
#define UPF_SPD_SHI ((__force upf_t) (0x1000))
#define UPF_SPD_WARP ((__force upf_t) (0x1010))
#define UPF_SKIP_TEST ((__force upf_t) (1 << 6))
#define UPF_AUTO_IRQ ((__force upf_t) (1 << 7))
#define UPF_HARDPPS_CD ((__force upf_t) (1 << 11))
#define UPF_LOW_LATENCY ((__force upf_t) (1 << 13))
#define UPF_BUGGY_UART ((__force upf_t) (1 << 14))
#define UPF_MAGIC_MULTIPLIER ((__force upf_t) (1 << 16))
#define UPF_CONS_FLOW ((__force upf_t) (1 << 23))
#define UPF_SHARE_IRQ ((__force upf_t) (1 << 24))
#define UPF_BOOT_AUTOCONF ((__force upf_t) (1 << 28))
#define UPF_FIXED_PORT ((__force upf_t) (1 << 29))
#define UPF_DEAD ((__force upf_t) (1 << 30))
#define UPF_IOREMAP ((__force upf_t) (1 << 31))
#define UPF_CHANGE_MASK ((__force upf_t) (0x17fff))
#define UPF_USR_MASK ((__force upf_t) (UPF_SPD_MASK|UPF_LOW_LATENCY))
unsigned int mctrl; /* current modem ctrl settings */
unsigned int timeout; /* character-based timeout */
unsigned int type; /* port type */
const struct uart_ops *ops;
unsigned int custom_divisor;
unsigned int line; /* port index */
unsigned long mapbase; /* for ioremap */
struct device *dev; /* parent device */
unsigned char hub6; /* this should be in the 8250 driver */
unsigned char unused[3];
void *private_data; /* generic platform data pointer */
};
串口核心层提供如下函数来添加一个端口:
int uart_add_one_port(struct uart_driver *drv, struct uart_port *port);
这个函数的调用应该发生在 uart_register_driver()之后,uart_add_one_port()的一个最重要作用是封装了 tty_register_device()。
int uart_add_one_port(struct uart_driver *drv, struct uart_port *port)
{
struct uart_state *state;
int ret = 0;
BUG_ON(in_interrupt());
if (port->line >= drv->nr)
return -EINVAL;
state = drv->state + port->line;
mutex_lock(&port_mutex);
mutex_lock(&state->mutex);
if (state->port) {
ret = -EINVAL;
goto out;
}
state->port = port;
port->cons = drv->cons;
port->info = state->info;
/*
* If this port is a console, then the spinlock is already
* initialised.
*/
if (!(uart_console(port) && (port->cons->flags & CON_ENABLED))) {
spin_lock_init(&port->lock);
lockdep_set_class(&port->lock, &port_lock_key);
}
uart_configure_port(drv, state, port);
/*
* Register the port whether it's detected or not. This allows
* setserial to be used to alter this ports parameters.
*/
tty_register_device(drv->tty_driver, port->line, port->dev);
/*
* If this driver supports console, and it hasn't been
* successfully registered yet, try to re-register it.
* It may be that the port was not available.
*/
if (port->type != PORT_UNKNOWN &&
port->cons && !(port->cons->flags & CON_ENABLED))
register_console(port->cons);
/*
* Ensure UPF_DEAD is not set.
*/
port->flags &= ~UPF_DEAD;
out:
mutex_unlock(&state->mutex);
mutex_unlock(&port_mutex);
return ret;
}
串口核心层提供如下函数来移除一个端口:
int uart_remove_one_port(struct uart_driver *drv, struct uart_port *port);
其中会调用 tty_unregister_device()。
int uart_remove_one_port(struct uart_driver *drv, struct uart_port *port)
{
struct uart_state *state = drv->state + port->line;
struct uart_info *info;
BUG_ON(in_interrupt());
if (state->port != port)
printk(KERN_ALERT "Removing wrong port: %p != %p\n",
state->port, port);
mutex_lock(&port_mutex);
/*
* Mark the port "dead" - this prevents any opens from
* succeeding while we shut down the port.
*/
mutex_lock(&state->mutex);
port->flags |= UPF_DEAD;
mutex_unlock(&state->mutex);
/*
* Remove the devices from the tty layer
*/
tty_unregister_device(drv->tty_driver, port->line);
info = state->info;
if (info && info->tty)
tty_vhangup(info->tty);
/*
* All users of this port should now be disconnected from
* this driver, and the port shut down. We should be the
* only thread fiddling with this port from now on.
*/
state->info = NULL;
/*
* Free the port IO and memory resources, if any.
*/
if (port->type != PORT_UNKNOWN)
port->ops->release_port(port);
/*
* Indicate that there isn't a port here anymore.
*/
port->type = PORT_UNKNOWN;
/*
* Kill the tasklet, and free resources.
*/
if (info) {
tasklet_kill(&info->tlet);
kfree(info);
}
state->port = NULL;
mutex_unlock(&port_mutex);
return 0;
}
3.uart_ops
uart_ops 定义针对 UART 的一系列操作,包括发送、接收及线路设置等,其定义如代码清单 14.16。Linux 驱动中面向对象编程中基类、派生类的关系,派生类(子类)针对特定的事物会更加具体,而基类(父类)则站在更高的抽象层次上。
代码清单 14.16 uart_ops 结构体
include/linux/serial_core.h
/*
* This structure describes all the operations that can be
* done on the physical hardware.
*/
struct uart_ops {
unsigned int (*tx_empty)(struct uart_port *);
void (*set_mctrl)(struct uart_port *, unsigned int mctrl);
unsigned int (*get_mctrl)(struct uart_port *);
void (*stop_tx)(struct uart_port *);
void (*start_tx)(struct uart_port *);
void (*send_xchar)(struct uart_port *, char ch);
void (*stop_rx)(struct uart_port *);
void (*enable_ms)(struct uart_port *);
void (*break_ctl)(struct uart_port *, int ctl);
int (*startup)(struct uart_port *);
void (*shutdown)(struct uart_port *);
void (*set_termios)(struct uart_port *, struct ktermios *new, struct ktermios *old);
void (*pm)(struct uart_port *, unsigned int state, unsigned int oldstate);
int (*set_wake)(struct uart_port *, unsigned int state);
/*一个描述端口类型的字符串 */
const char *(*type)(struct uart_port *);
/* 释放该端口使用的 I/O 和 memory 资源 */
void (*release_port)(struct uart_port *);
/* 申请该端口使用的 I/O 和 memory 资源 */
int (*request_port)(struct uart_port *);
void (*config_port)(struct uart_port *, int);
int (*verify_port)(struct uart_port *, struct serial_struct *);
int (*ioctl)(struct uart_port *, unsigned int, unsigned long);
};
分析:
serial_core.c 中定义 tty_operations 的实例,包含 uart_open()、uart_close()、uart_write()、uart_send_xchar()等成员函数(如代码清单 14.17)。
static const struct tty_operations uart_ops = {
.open = uart_open,
.close = uart_close,
.write = uart_write,
.put_char = uart_put_char,
.flush_chars = uart_flush_chars,
.write_room = uart_write_room,
.chars_in_buffer= uart_chars_in_buffer,
.flush_buffer = uart_flush_buffer,
.ioctl = uart_ioctl,
.throttle = uart_throttle,
.unthrottle = uart_unthrottle,
.send_xchar = uart_send_xchar,
.set_termios = uart_set_termios,
.stop = uart_stop,
.start = uart_start,
.hangup = uart_hangup,
.break_ctl = uart_break_ctl,
.wait_until_sent= uart_wait_until_sent,
#ifdef CONFIG_PROC_FS
.read_proc = uart_read_proc,
#endif
.tiocmget = uart_tiocmget,
.tiocmset = uart_tiocmset,
};
这些函数借助 uart_ops 结构体中的成员函数来完成具体的操作,代码清单14.18所示为tty_operations的uart_send_xchar()成员函数利用uart_ops中 start_tx()、send_xchar()成员函数的例子。
代码清单 14.18 串口核心层的 tty_operations 与 uart_ops 关系
static void uart_send_xchar(struct tty_struct *tty, char ch)
{
struct uart_state *state = tty->driver_data;
struct uart_port *port = state->port;
unsigned long flags;
/* 如果 uart_ops 中实现send_xchar 成员函数 */
port->ops->send_xchar(port, ch);
else { /* uart_ops 中未实现 send_xchar 成员函数 */
port->x_char = ch;
if (ch) {
spin_lock_irqsave(&port->lock, flags);
port->ops->start_tx(port);/* 发送 xchar */
spin_unlock_irqrestore(&port->lock, flags);
}
}
}
总结:
在使用串口核心层这个通用串口 tty 驱动层的接口后,一个串口驱动主要完成的工作如下。
1、定义 uart_driver、uart_ops、uart_port 等结构体的实例,在适当的地方根据具体硬件和驱动的情况初始化,具体设备 xxx 的驱动可以将这些结构套在新定义的 xxx_uart_driver、xxx_uart_ops、xxx_uart_port 之内。
2、在模块初始化时调用 uart_register_driver()和 uart_add_one_port()以注册 UART 驱动并添加端口,在模块卸载时调用 uart_unregister_driver()和 uart_remove_one_port()以注销 UART 驱动并移除端口。
3、根据具体硬件的 datasheet 实现 uart_ops 中的成员函数,这些函数的实现成为 UART 驱动的主体工作。