1 winform 读取excel文档
1)点击button按钮,弹出上传excel窗口
private void button_headcompany_Click(object sender, EventArgs e) { string HeadCompany_rowValue = HeadCompany_row.Text; string HeadCompany_columnValue = HeadCompany_column.Text; if (string.IsNullOrEmpty(HeadCompany_rowValue) || string.IsNullOrEmpty(HeadCompany_columnValue)) { MessageBox.Show("请填写行和列的值,例如 行A5 列BB", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information); return; } ButtonClick("总公司汇总", HeadCompany_rowValue, HeadCompany_columnValue); }
2)判断是否上传的文档格式是否正常 xls和xlsx类型的文档
public static void ButtonClick(string SheetType,string rowValue,string ColumnValue) { string worksheetname = string.Empty; string filepath = ""; //导入本地文件 OpenFileDialog file = new OpenFileDialog(); file.Filter = "文档(*.xls)|*.xls|文档(*.xlsx)|*.xlsx"; if (file.ShowDialog() == DialogResult.OK) filepath = file.FileName; string fileNameWithoutExtension = System.IO.Path.GetDirectoryName(filepath);// 没有扩展名的文件名 “Default” //判断有没有文件导入 if (file.FileName.Length == 0) { MessageBox.Show("请选择要导入的文件", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information); return; } DataSet ds = new DataSet(); DataTable dt = new DataTable(); ////改变了原来的逻辑,直接从中间表导出数据Excel,ADDBYxingkai // dt = Liveflow.HaiKang.ExcelHelper.ExcelToDataTable(filePath, 1, 27, -1, 0, 1); //把excel表数据读取到DataTable中 string strConn = string.Empty; string excelName = SheetType; //注意:把一个excel文件看做一个数据库,一个sheet看做一张表。语法 "SELECT * FROM [sheet1$]",表单要使用"[]"和"$" // 1、HDR表示要把第一行作为数据还是作为列名,作为数据用HDR=no,作为列名用HDR=yes; // 2、通过IMEX=1来把混合型作为文本型读取,避免null值。 // 3、判断是xls还是xlsx string[] sArray = filepath.Split('.'); if (sArray[1].ToString() == "xls") { // strConn = "Provider=Microsoft.Ace.OleDb.12.0;" + "data source=" + filepath + ";Extended Properties='Excel 12.0; HDR=Yes; IMEX=1';"; strConn = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" + filepath + ";Extended Properties='Excel 12.0;HDR=NO;IMEX=1';"; // Office 07及以上版本 不能出现多余的空格 而且分号注意 //dt = HRManageXls(strConn, excelName, SheetType, fileNameWithoutExtension, rowValue, ColumnValue); } else if (sArray[1].ToString() == "xlsx") { strConn = "Provider=Microsoft.Ace.OleDb.12.0;data source='" + filepath + "';Extended Properties='Excel 12.0; HDR=NO; IMEX=1';"; HRManageXlsX(strConn, excelName, SheetType, fileNameWithoutExtension, rowValue, ColumnValue); } }
3)读取excel文档中的数据 转换为datatable
public static void HRManageXlsX(string strConn, string excelName,string SheetType,string fileNameWithoutExtension, string rowValue, string ColumnValue) { DataTable dt = new DataTable(); dt=GetCsvToDataTable(strConn, excelName, SheetType,rowValue, ColumnValue);//获取excel数据 //指定源列和目标列之间的对应关系 int row = dt.Rows.Count;//exce数据总行 int col = dt.Columns.Count;//excel数据总列 //生成两张txt for (int i = 0; i < row; i++) { if (i >= 2) { if (!string.IsNullOrEmpty(dt.Rows[i][0].ToString())) { //总公司QuotaSQl.txt string QuotaSQl = "INSERT INTO dbo.TB_HR_SatisfactionEvaluation_Quota(IId,EvaluationYear , EvaluationType , LocationName ,DescVal ,DeptName ,EvaluationDesc ,enable ,createby ,CreateTime ,updateby ,updateTime)VALUES (" + dt.Rows[i][4].ToString() + ",'2018', '" + dt.Rows[i][0].ToString() + "','" + dt.Rows[i][0].ToString() + "', '" + dt.Rows[i][2].ToString() + "','" + dt.Rows[i][1].ToString() + "','" + dt.Rows[i][3].ToString().Replace("\n", "").Replace(" ", "").Replace("\t", "").Replace("\r", "") + "', 1,'',GETDATE(),'' ,GETDATE());"; WriteLog(QuotaSQl, "总公司QuotaSQl", fileNameWithoutExtension); } if (!string.IsNullOrEmpty(dt.Rows[i][4].ToString())) { for (int j = 5; j < col; j++) { if (!string.IsNullOrEmpty(dt.Rows[i][j].ToString())) { //总公司UserSQl.txt string UserSQl = "INSERT INTO dbo.TB_HR_SatisfactionEvaluation_Quota_User( EvaluationYear ,QuotaID ,QuotoDept ,Quoter ,enable ,createby , CreateTime ,updateby ,updateTime ,Dept)VALUES ('2018','" + dt.Rows[i][4].ToString() + "','" + dt.Rows[0][j].ToString() + "','" + dt.Rows[i][j].ToString() + "',1,'sa',GETDATE(),'',GETDATE(),'');"; WriteLog(UserSQl, "总公司UserSQl", fileNameWithoutExtension); } } } } } MessageBox.Show("读取信息完毕,已导出txt文件;路径为"+ fileNameWithoutExtension + "", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information); }
4)关键方法
public static DataTable GetCsvToDataTable(string strConn, string excelName, string FillName, string rowValue, string ColumnValue) { System.Data.OleDb.OleDbConnection conn = new System.Data.OleDb.OleDbConnection(strConn); conn.Open(); DataSet ds = new DataSet(); System.Data.OleDb.OleDbDataAdapter odda = new System.Data.OleDb.OleDbDataAdapter(string.Format("select * from [" + excelName + "$"+ rowValue + ":"+ ColumnValue + "]"), conn); //这里的表名参数,就是 CSV的完整文件名 odda.Fill(ds, FillName); conn.Close(); return ds.Tables[0]; }
5)写入txt
/// <summary> /// 写入日志 /// </summary> /// <param name="logstr">写入的内容</param> public static void WriteLog(string logstr,string Txtname,string fileNameWithoutExtension) { string strFileName = fileNameWithoutExtension+"/"+Txtname+ ".txt"; using (FileStream fs = new FileStream(strFileName, FileMode.Append)) { using (StreamWriter sw = new StreamWriter(fs, Encoding.Default)) { //声明数据流文件写入方法 sw.WriteLine(logstr); sw.Close(); fs.Close(); } } }
扫描二维码关注公众号,回复:
1664728 查看本文章
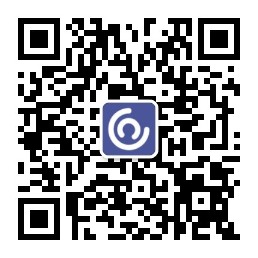