JavaScript是一门灵活且强大的编程语言,其特有的原型链和继承机制是其核心特性之一。在本文中,我们将深入探讨JavaScript中的原型链和继承机制,帮助读者更好地理解这一重要概念。
什么是原型链?
在JavaScript中,每个对象都有一个内部链接到另一个对象的引用,这个对象被称为原型(prototype)。原型对象可以包含属性和方法,并且其他对象可以继承这些属性和方法。当我们访问一个对象的属性或方法时,JavaScript会首先在对象本身查找,如果找不到,它会沿着原型链向上查找,直到找到该属性或方法或到达原型链的顶端。
在上面提到的代码中,我们定义了两个类Person
和Student
,并使用extends
关键字创建了Student
类的子类Teacher
。我们可以通过原型链实现方法的继承,确保子类可以访问父类的方法。
创建对象的原型链
每个JavaScript对象都有一个内置的属性__proto__
,它指向了创建该对象的构造函数的原型对象。这个链接就是原型链的关键。让我们通过示例来理解这一点:
function Animal() {}
const dog = new Animal();
console.log(dog.__proto__); // 输出:Animal {}
console.log(Animal.prototype); // 输出:Animal {}
console.log(dog.__proto__ === Animal.prototype); // 输出:true
在上面的例子中,我们创建了一个空的Animal
构造函数,然后使用new
关键字创建了一个名为dog
的对象。dog.__proto__
指向了Animal.prototype
,这样dog
就可以继承Animal.prototype
上的属性和方法。
原型链继承与方法重写
在JavaScript中,子类可以继承父类的方法,也可以重写继承的方法。让我们通过上面给出的代码示例来说明:
class Person{
constructor(name) {
this.name=name;
}
introduce(){
console.log(`Hello, I am ${this.name}`);
}
drink(){
console.log('I am drinking');
}
}
class Student extends Person{
constructor(name,score) {
super(name)
this.score= score;
}
introduce(){
console.log(`Hello, I am ${this.name}.and ${this.score}is my score`);
}
}
const student =new Student('John', 100);
student.introduce();
class Teacher extends Person{
constructor(name,subject){
super(name)
this.subject=subject;
}
introduce(){
console.log(`Hello, I am ${this.name}.and ${this.subject}is my subject`);
}
}
const teacher =new Teacher('John', 'Math');
teacher.introduce();
console.log(student)
teacher.drink();
在上面的例子中,Student
类继承了Person
类,并重写了introduce()
方法。当我们调用student.introduce()
时,它会执行Student
类中的introduce()
方法,而不是Person
类中的方法。
继承与super关键字
在子类中,我们可以使用super
关键字来调用父类的构造函数和方法。这样可以保持子类与父类的关联,并在子类中添加额外的功能。让我们继续上面的例子,为Teacher
类添加一个新方法并调用父类的构造函数:
在上面的例子中,我们通过super(name)
调用了Person
构造函数,确保Teacher
类实例化时也会调用父类的构造函数进行初始化。
class Teacher extends Person {
constructor(name, subject) {
super(name);
this.subject = subject;
}
introduce() {
console.log(`Hello, I am ${this.name}. and ${this.subject} is my subject`);
}
teach() {
console.log('I am teaching');
}
}
const teacher = new Teacher('John', 'Math');
teacher.introduce(); // 输出:Hello, I am John. and Math is my subject
teacher.teach(); // 输出:I am teaching
总结
JavaScript的原型链和继承机制是其强大的特性之一。通过原型链,对象可以继承其他对象的属性和方法,实现代码的复用和组织。在子类中,我们可以通过extends
关键字继承父类,并使用super
关键字调用父类的构造函数和方法。这样,我们可以创建更加灵活和可扩展的代码结构。
希望本文对您理解JavaScript的原型链和继承机制有所帮助。深入理解这一概念将使您在编写JavaScript代码时更加得心应手。Happy coding!
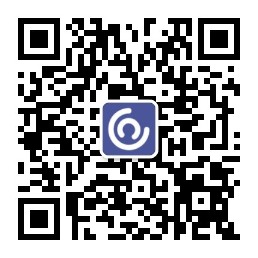