一、
1.掌握Hbase在Hadoop集群体系结构中发挥的作用和使过程。
2.掌握安装和配置HBase基本方法。
3.掌握HBase shell的常用命令。
4.使用HBase shell命令进行表的创建,增加删除修改操作。
5.使用Java API进行表的创建,增加删除修改操作。
二、
1.完成Zookeeper和HBase的搭建。
2.使用HBase shell命令进行表的创建,增加删除修改操作。
3.使用Java API进行表的创建,增加删除修改操作。
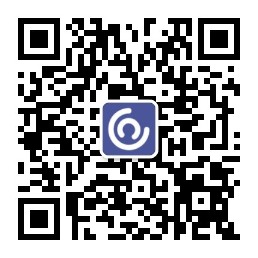
一:
- 掌握Hbase在Hadoop集群体系结构中发挥的作用和使过程。
- 掌握安装和配置HBase基本方法。
Hbase的安装与配置已在实验一上传
- 掌握HBase shell的常用命令。
4.使用HBase shell命令进行表的创建,增加删除修改操作。
1. HBase Shell基本操作
(1)使用HBase Shell创建表、查看表结构、增加列族、删除列族;
(2)使用HBase Shell添加数据、查看数据、查看表中的记录总数;
(3)使用HBase Shell删除数据、清空表里的内容、删除表;
(4)使用HBase Shell对表的列族可配置参数进行调整,使其可以查看多个版本的数据。
status 查询服务器状态
version 查看版本
(1)使用HBase Shell创建表、查看表结构、增加列族、删除列族;
创建表
create ‘scores’,’grade’,’course’
查看表结构
desc ‘scores’
增加列族
disable ‘scores’
Alter ‘scores’,{NAME=>’school’}
删除列族(disable后alter)
disable ‘scores’
alter 'scores',{NAME=>'school',METHOD=>'delete'}
(2)使用HBase Shell添加数据、查看数据、查看表中的记录总数;
1、添加数据
put ‘表名’,’行键’,’列族:列限定符’,’值’
put 'scores','Tom','grade:','5'
put 'scores','Tom','course:math','97'
put 'scores','Tom','course:art','87'
put 'scores','Tom','course:english','80'
put 'scores','Jim','grade:','4'
put 'scores','Jim','course:chinese','89'
put 'scores','Jim','course:english','80'
2、查看数据
scan ‘scores’
3、查看表的记录总数
count ‘scores’
(3)使用HBase Shell删除数据、清空表里的内容、删除表;
1、删除数据
- 删除一个单元格
delete 'scores','Jim','grade:'
delete 'scores','Jim','course:chinese'
或:
deleteall 'scores','Tom','course:english'
b.删除一行
deleteall 'scores','Tom'
2、清空表内容
表的内容清空,但表的结构还在
truncate 'scores'
scan 'scores' 内容清空
desc 'scores' 结构存在
3、删除表
删除一个表(先禁用表,再删除表)
disable 'scores'
drop 'scores'
- 使用Java API进行表的创建,增加删除修改操作。
package putfile;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellUtil;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.KeyValue;
import org.apache.hadoop.hbase.MasterNotRunningException;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.ZooKeeperConnectionException;
import org.apache.hadoop.hbase.client.Delete;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import org.apache.hadoop.hbase.client.HTable;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.protobuf.generated.ClientProtos.Column;
public class Hbase {
static Configuration conf = null;
static{
conf = HBaseConfiguration.create();
conf.set("hbase.rootdir", "hdfs://10.49.23.127:9000/hbase");
conf.set("hbase.master", "hdfs://10.49.23.127:60000");
conf.set("hbase.zookeeper.property.clientPort", "2181");
conf.set("hbase.zookeeper.quorum", "master,slave1,slave2");
}
//实现了list功能
public static List<String> getAllTables() throws MasterNotRunningException, ZooKeeperConnectionException, IOException{
List<String> list = null;
//HBaseAdmin可以创建表、删除表、查看表
HBaseAdmin admin = new HBaseAdmin(conf);
if(admin != null){
//HTableDescriptor表的相关信息
HTableDescriptor[] tables = admin.listTables();
for(HTableDescriptor table : tables){
System.out.println(table.getNameAsString());
list = new ArrayList<String>();
list.add(table.getNameAsString());
}
}
admin.close();
return list;
}
public static int createTabble(String tableName, String[] family) throws MasterNotRunningException, ZooKeeperConnectionException, IOException{
HBaseAdmin admin = new HBaseAdmin(conf);
HTableDescriptor table = new HTableDescriptor(TableName.valueOf(tableName));
// HTableDescriptor table = new HTableDescriptor(tableName);
//HColumnDescriptor列的相关信息
for(String str : family){
HColumnDescriptor cloumn = new HColumnDescriptor(str);
cloumn.setMaxVersions(3);
table.addFamily(cloumn);
}
if(admin.tableExists(tableName)){
System.out.println(tableName + "已经存在");
return -1;
}
admin.createTable(table);
admin.close();
return 1;
}
public static void deleteTable(String tableName) throws MasterNotRunningException, ZooKeeperConnectionException, IOException{
HBaseAdmin admin = new HBaseAdmin(conf);
admin.disableTable(tableName);
admin.deleteTable(tableName);
System.out.println(tableName + "成功删除");
admin.close();
}
public static void insert(String tableName, String rowkey, String family, String column, String cell) throws IOException{
Put put = new Put(rowkey.getBytes());
//HTable负责表的get put delete操作
HTable table = new HTable(conf, tableName);
put.add(family.getBytes(), column.getBytes(), cell.getBytes());
table.put(put);
}
public static Result queryByRow(String tableName, String rowkey) throws IOException{
Get get = new Get(rowkey.getBytes());
HTable table = new HTable(conf, tableName);
return table.get(get);
}
public static Result queryByColumn(String tableName, String rowkey, String family, String column) throws IOException{
Get get = new Get(rowkey.getBytes());
HTable table = new HTable(conf, tableName);
get.addColumn(family.getBytes(), column.getBytes());
return table.get(get);
}
public static Result queryByRowByVersions(String tableName, String rowkey) throws IOException{
Get get = new Get(rowkey.getBytes());
get.setMaxVersions(3);
HTable table = new HTable(conf, tableName);
return table.get(get);
}
public static ResultScanner queryByScan(String tableName) throws IOException{
Scan scan = new Scan();
// scan.setStartRow(startRow);
// scan.setStopRow(stopRow);
// scan.addColumn(family, qualifier);
HTable table = new HTable(conf, tableName);
return table.getScanner(scan);
}
public static void deleteByColumn(String tableName, String rowkey, String family, String column) throws IOException{
Delete delete = new Delete(rowkey.getBytes());
HTable table = new HTable(conf, tableName);
delete.deleteColumn(family.getBytes(), column.getBytes());
table.delete(delete);
System.out.println("成功删除");
}
public static void deleteByRow(String tableName, String rowkey) throws IOException{
Delete delete = new Delete(rowkey.getBytes());
HTable table = new HTable(conf, tableName);
table.delete(delete);
System.out.println("成功删除");
}
public static void main(String[] args) throws MasterNotRunningException, ZooKeeperConnectionException, IOException {
getAllTables();
//1.创建book表
// createTabble("book", new String[]{"author", "info"});
// getAllTables();
//2.删除book表
// deleteTable("book");
// getAllTables();
//3.创建book表
// createTabble("book", new String[]{"author", "info"});
// getAllTables();
//4.book表插入数据
// insert("book", "rw001", "author", "name", "zhangsan");
// insert("book", "rw001", "author", "age", "65");
// insert("book", "rw001", "info", "name", "database");
// insert("book", "rw001", "info", "price", "35.6");
//5.按行查询数据(旧方法)
// Result result = queryByRow("book", "rw001");
// List<KeyValue> values = result.list();
// System.out.println("COLUMN\t\t\tCELL ");
// for(KeyValue value : values){
// System.out.print(new String(value.getFamily()) + ":");
// System.out.print(new String(value.getQualifier()) + "\t\t");
// System.out.print("value = " + new String(value.getValue()) + ",");
// System.out.println("timestamp = " + value.getTimestamp());
// }
//5.按行查询数据(新方法)
// Result result = queryByRow("book", "rw001");
// Cell[] cells = result.rawCells();
// System.out.println("COLUMN\t\t\tCELL ");
// for(Cell cell : cells){
// System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
// System.out.print(new String(CellUtil.cloneQualifier(cell)) + "\t\t");
// System.out.print("value = " + new String(CellUtil.cloneValue(cell)) + ",");
// System.out.println("timestamp = " + cell.getTimestamp());
// }
//6.按列查询数据(新方法)
// Result result = queryByColumn("book", "rw001", "author", "name");
// Cell[] cells = result.rawCells();
// System.out.println("COLUMN\t\t\tCELL ");
// for(Cell cell : cells){
// System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
// System.out.print(new String(CellUtil.cloneQualifier(cell)) + "\t\t");
// System.out.print("value = " + new String(CellUtil.cloneValue(cell)) + ",");
// System.out.println("timestamp = " + cell.getTimestamp());
// }
//7.scan
// ResultScanner scanner = queryByScan("book");
// System.out.println("COLUMN\t\t\tCELL ");
// for(Result result : scanner){
// Cell[] cells = result.rawCells();
//
// for(Cell cell : cells){
// System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
// System.out.print(new String(CellUtil.cloneQualifier(cell)) + "\t\t");
// System.out.print("value = " + new String(CellUtil.cloneValue(cell)) + ",");
// System.out.println("timestamp = " + cell.getTimestamp());
// }
// }
//8.删除某一列
// deleteByColumn("book", "rw001", "author", "name");
//9.删除某一行数据
// deleteByRow("book", "rw001");
//10. 按行查询多版本数据(新方法)
// Result result = queryByRowByVersions("book", "rw001");
// Cell[] cells = result.rawCells();
// System.out.println("COLUMN\t\t\tCELL ");
// for(Cell cell : cells){
// System.out.print(new String(CellUtil.cloneFamily(cell)) + ":");
// System.out.print(new String(CellUtil.cloneQualifier(cell)) + "\t\t");
// System.out.print("value = " + new String(CellUtil.cloneValue(cell)) + ",");
// System.out.println("timestamp = " + cell.getTimestamp());
// }
}
}