面向对象编程三大特征介绍
关于面向对象编程基础可参见 https://blog.csdn.net/cnds123/article/details/128998309
面向对象三大特征
封装(Encapsulation)
将属性和方法写到类的里面的操作,或者说封装是将数据(属性)和操作(方法)封装在类中。
通过封装,可以隐藏内部的实现细节,只暴露必要的接口给其他对象使用。这样可以提高代码的可维护性和重用性。
继承(Inheritance)
继承是指一个类(父类/基类)可以派生出另一个类(子类/派生类),子类可以继承父类的属性和方法。
继承使得代码具有层次结构,可以定义通用的特性和行为,并在子类中进行修改和扩展。继承可以提高代码的可复用性和扩展性。
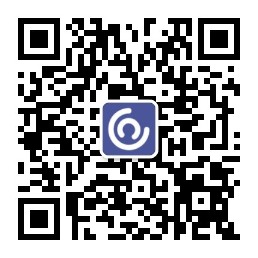
多态(Polymorphism)
多态是一种使用对象的方式,子类重写父类方法,当使用父类的引用调用一个被子类重写的方法时,实际执行的是子类中对该方法的实现。
多态是指同一个类型的对象,在不同的情况下表现出不同的行为。
多态性通过函数重载、函数重写和抽象类/接口等机制来实现,为编程带来了更高的灵活性和可扩展性。
特别示例解释:当我们使用父类的引用调用一个被子类重写(override)的方法时,实际执行的是子类中对该方法的实现。假设我们有一个父类 Animal 和两个子类 Cat 和 Dog,它们都继承自 Animal 类,并且都重写了一个名为 makeSound() 的方法。如果我们使用父类 Animal 的引用或指针来指向一个子类对象,并调用 makeSound() 方法,实际执行的是该子类中对 makeSound() 方法的具体实现。
★ Java示例源码:
class Animal {
public void makeSound() {
System.out.println("Animal is making a sound");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Cat is meowing");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Dog is barking");
}
}
public class Main {
public static void main(String[] args) {
Animal animal1 = new Cat();
Animal animal2 = new Dog();
animal1.makeSound(); // 实际执行的是 Cat 类中的 makeSound() 方法
animal2.makeSound(); // 实际执行的是 Dog 类中的 makeSound() 方法
}
}
C++ 示例源码:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void makeSound() {
cout << "Animal is making a sound" << endl;
}
};
class Cat : public Animal {
public:
void makeSound() override {
cout << "Cat is meowing" << endl;
}
};
class Dog : public Animal {
public:
void makeSound() override {
cout << "Dog is barking" << endl;
}
};
int main() {
Animal* animal1 = new Cat();
Animal* animal2 = new Dog();
animal1->makeSound(); // 实际执行的是 Cat 类中的 makeSound() 方法
animal2->makeSound(); // 实际执行的是 Dog 类中的 makeSound() 方法
delete animal1;
delete animal2;
return 0;
}
★ Python 示例源码:
class Animal:
def makeSound(self):
print("Animal is making a sound")
class Cat(Animal):
def makeSound(self):
print("Cat is meowing")
class Dog(Animal):
def makeSound(self):
print("Dog is barking")
animal1 = Cat()
animal2 = Dog()
animal1.makeSound() # 实际执行的是 Cat 类中的 makeSound() 方法
animal2.makeSound() # 实际执行的是 Dog 类中的 makeSound() 方法
★ JavaScript 示例源码:
<script>
class Animal {
makeSound() {
console.log("Animal is making a sound");
}
}
class Cat extends Animal {
makeSound() {
console.log("Cat is meowing");
}
}
class Dog extends Animal {
makeSound() {
console.log("Dog is barking");
}
}
const animal1 = new Cat();
const animal2 = new Dog();
animal1.makeSound(); // 实际执行的是 Cat 类中的 makeSound() 方法
animal2.makeSound(); // 实际执行的是 Dog 类中的 makeSound() 方法
</script>
在上述代码中,使用多种语言演示了通过子类重写父类方法,在具体对象上调用该方法时,实际执行的是子类中对应的方法实现。具体说来,使用 Animal 类型的引用 animal1 和 animal2 分别指向 Cat 和 Dog 类的对象,当我们调用 makeSound() 方法时,实际执行的是每个对象所属的子类中的 makeSound() 方法。