首先需要配置Android studio对protobuf的支持
参考资料
https://blog.csdn.net/Dream_go888/article/details/80092991
https://plugins.gradle.org/plugin/com.google.protobuf#new-plugin-mechanism-info-body
https://github.com/google/protobuf-gradle-plugin
第一步,添加protobuf-gradle-plugin插件
参考https://github.com/google/protobuf-gradle-plugin,在项目根目录build.gradle里添加
buildscript { repositories { mavenCentral() } dependencies { classpath 'com.google.protobuf:protobuf-gradle-plugin:0.8.5' } }
完整示例如下
// Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { repositories { google() jcenter() //yuyong-->https://github.com/google/protobuf-gradle-plugin mavenCentral() } dependencies { classpath 'com.android.tools.build:gradle:3.1.2' // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files // yuyong-->https://github.com/google/protobuf-gradle-plugin classpath 'com.google.protobuf:protobuf-gradle-plugin:0.8.5' } } allprojects { repositories { google() jcenter() } } task clean(type: Delete) { delete rootProject.buildDir }
同步项目
第二步,对app模块进行配置,使之支持protobuf
在app模块下build.gradle里添加:
插件配置
apply plugin: 'com.google.protobuf'
指明proto文件位置
sourceSets { main { proto { srcDir 'src/main/proto' } } }
定义protoc编译(实际上就是根据proto生成java或者C++代码文件)规则
protobuf { protoc { artifact = 'com.google.protobuf:protoc:3.5.1-1' } generateProtoTasks { all().each { task -> task.builtins { remove java } task.builtins { java {} // Add cpp output without any option. // DO NOT omit the braces if you want this builtin to be added. cpp {} } } } generatedFilesBaseDir = "$projectDir/src/generated" }
添加相关Java库支持(如果需要支持C++,需要添加C++库的支持)
扫描二维码关注公众号,回复:
1570298 查看本文章
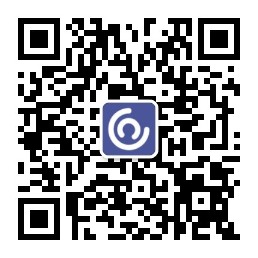
implementation 'com.google.protobuf:protobuf-java:3.5.1' implementation 'com.google.protobuf:protoc:3.5.1-1'
完整示例如下
apply plugin: 'com.android.application' apply plugin: 'com.google.protobuf' android { compileSdkVersion 27 defaultConfig { applicationId "com.thinking.client" minSdkVersion 15 targetSdkVersion 27 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } sourceSets { main { proto { srcDir 'src/main/proto' } } } } protobuf { protoc { artifact = 'com.google.protobuf:protoc:3.5.1-1' } generateProtoTasks { all().each { task -> task.builtins { remove java } task.builtins { java {} // Add cpp output without any option. // DO NOT omit the braces if you want this builtin to be added. cpp {} } } } generatedFilesBaseDir = "$projectDir/src/generated" } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:27.1.1' implementation 'com.android.support.constraint:constraint-layout:1.1.0' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' implementation 'com.google.protobuf:protobuf-java:3.5.1' implementation 'com.google.protobuf:protoc:3.5.1-1' }
同步,rebuild
至此,Android Studio完整了对protobuf的支持。
下面参考https://grpc.io/docs/quickstart/android.html创建一个test.proto文件
// Copyright 2015, gRPC Authors // All rights reserved. // // Licensed under the Apache License, Version 2.0 (the "License"); // you may not use this file except in compliance with the License. // You may obtain a copy of the License at // // http://www.apache.org/licenses/LICENSE-2.0 // // Unless required by applicable law or agreed to in writing, software // distributed under the License is distributed on an "AS IS" BASIS, // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. // See the License for the specific language governing permissions and // limitations under the License. syntax = "proto3"; option java_multiple_files = true; option java_package = "com.thinking.client"; option java_outer_classname = "HelloWorldProto"; option objc_class_prefix = "HLW"; package helloworld; // The greeting service definition. service Greeter { // Sends a greeting rpc SayHello (HelloRequest) returns (HelloReply) {} } // The request message containing the user's name. message HelloRequest { string name = 1; } // The response message containing the greetings message HelloReply { string message = 1; }
rebuild
可以看到在$projectDir/src/generated下可以看到由test.proto文件生成的Java和C++代码文件。