- [哈希表]
Given a non-empty array of non-negative integers nums
, the degree of this array is defined as the maximum frequency of any one of its elements.
Your task is to find the smallest possible length of a (contiguous) subarray of nums
, that has the same degree as nums
.
Example 1:
Input: nums = [1,2,2,3,1]
Output: 2
Explanation:
- The input array has a degree of 2 because both elements 1 and 2 appear twice.
- Of the subarrays that have the same degree:
[1, 2, 2, 3, 1], [1, 2, 2, 3], [2, 2, 3, 1], [1, 2, 2], [2, 2, 3], [2, 2]
- The shortest length is 2. So return 2.
Example 2:
Input: nums = [1,2,2,3,1,4,2]
Output: 6
Explanation:
- The degree is 3 because the element 2 is repeated 3 times.
- So [2,2,3,1,4,2] is the shortest subarray, therefore returning 6.
Constraints:
nums.length will be between 1 and 50,000.
nums[i] will be an integer between 0 and 49,999.
Thought
- 在一定时间内,没有理解题目意思
- 直接看的题解^ ~ ^
AC
/*
* @lc app=leetcode.cn id=697 lang=cpp
*
* [697] 数组的度
*/
// @lc code=start
class Solution {
public:
int findShortestSubArray(vector<int>& nums) {
unordered_map<int, vector<int>> mp;
int n = nums.size();
for(int i = 0; i < n; i++)
{
if(mp.count(nums[i]))
{
mp[nums[i]][0]++;
mp[nums[i]][2] = i;
}
else
{
mp[nums[i]] = {
1, i, i};
}
}
int maxNum = 0, minLen = 0;
for(auto& [_, vec] : mp)
{
if(maxNum < vec[0])
{
maxNum = vec[0];
minLen = vec[2] - vec[1] + 1;
}
else if(maxNum == vec[0])
{
if(minLen > vec[2] - vec[1] + 1)
{
minLen = vec[2] - vec[1] + 1;
}
}
}
return minLen;
}
};
// @lc code=end
- 将数组元素出现的次数,出现某一数值的初始位置和结束位置存放到一个3列的哈希表中
- 循环遍历,通过最大出现次数将最小长度取出。
Notice
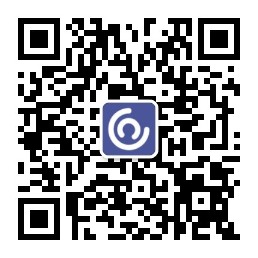
这是C++17引入的一种新的循环形式,被称为范围基于循环(range-based for loop)
或结构化绑定循环(structured binding loop)
。
在给定的循环中,map
是一个容器,auto& [_, vec]
是循环的迭代变量,用于迭代容器中的元素。这里使用了结构化绑定(structured binding)的语法来同时绑定键和值。
具体来说,每次迭代时,_
(下划线)代表键,vec
代表值。由于循环中使用了引用,所以对 vec
的修改会反映到原始容器中。
这种循环形式可以用于遍历关联容器(如 std::map
、std::unordered_map
等)的键值对。通过使用结构化绑定,我们可以方便地访问容器中的键和值,而不需要使用迭代器或 .first
和 .second
成员函数。
请注意,由于在循环中使用了 _
,这表示我们在迭代过程中不关心键的值。这是一种常见的做法,用于避免编译器警告或命名冲突。