【LeetCode】697. Degree of an Array 数组的度(Easy)(JAVA)
题目地址: https://leetcode.com/problems/degree-of-an-array/
题目描述:
Given a non-empty array of non-negative integers nums, the degree of this array is defined as the maximum frequency of any one of its elements.
Your task is to find the smallest possible length of a (contiguous) subarray of nums, that has the same degree as nums.
Example 1:
Input: nums = [1,2,2,3,1]
Output: 2
Explanation:
The input array has a degree of 2 because both elements 1 and 2 appear twice.
Of the subarrays that have the same degree:
[1, 2, 2, 3, 1], [1, 2, 2, 3], [2, 2, 3, 1], [1, 2, 2], [2, 2, 3], [2, 2]
The shortest length is 2. So return 2.
Example 2:
Input: nums = [1,2,2,3,1,4,2]
Output: 6
Explanation:
The degree is 3 because the element 2 is repeated 3 times.
So [2,2,3,1,4,2] is the shortest subarray, therefore returning 6.
Constraints:
- nums.length will be between 1 and 50,000.
- nums[i] will be an integer between 0 and 49,999.
题目大意
给定一个非空且只包含非负数的整数数组 nums,数组的度的定义是指数组里任一元素出现频数的最大值。
你的任务是在 nums 中找到与 nums 拥有相同大小的度的最短连续子数组,返回其长度。
解题方法
- 要找出数组的度,可以用一个 map 把每个元素的度都记录下来
- 再遍历 map 把出现次数最多而且度最小的找出来就是结果
class Solution {
public int findShortestSubArray(int[] nums) {
if (nums.length <= 0) return 0;
Map<Integer, int[]> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int[] pre = map.get(nums[i]);
if (pre != null) {
map.put(nums[i], new int[]{pre[0] + 1, pre[1], i});
} else {
map.put(nums[i], new int[]{1, i, i});
}
}
int min = 0;
int maxCount = 0;
for (Map.Entry<Integer, int[]> entry : map.entrySet()) {
int[] temp = entry.getValue();
if (temp[0] > maxCount) {
maxCount = temp[0];
min = temp[2] - temp[1] + 1;
} else if (maxCount == temp[0]) {
min = Math.min(min, temp[2] - temp[1] + 1);
}
}
return min;
}
}
执行耗时:17 ms,击败了84.89% 的Java用户
内存消耗:44 MB,击败了7.13% 的Java用户
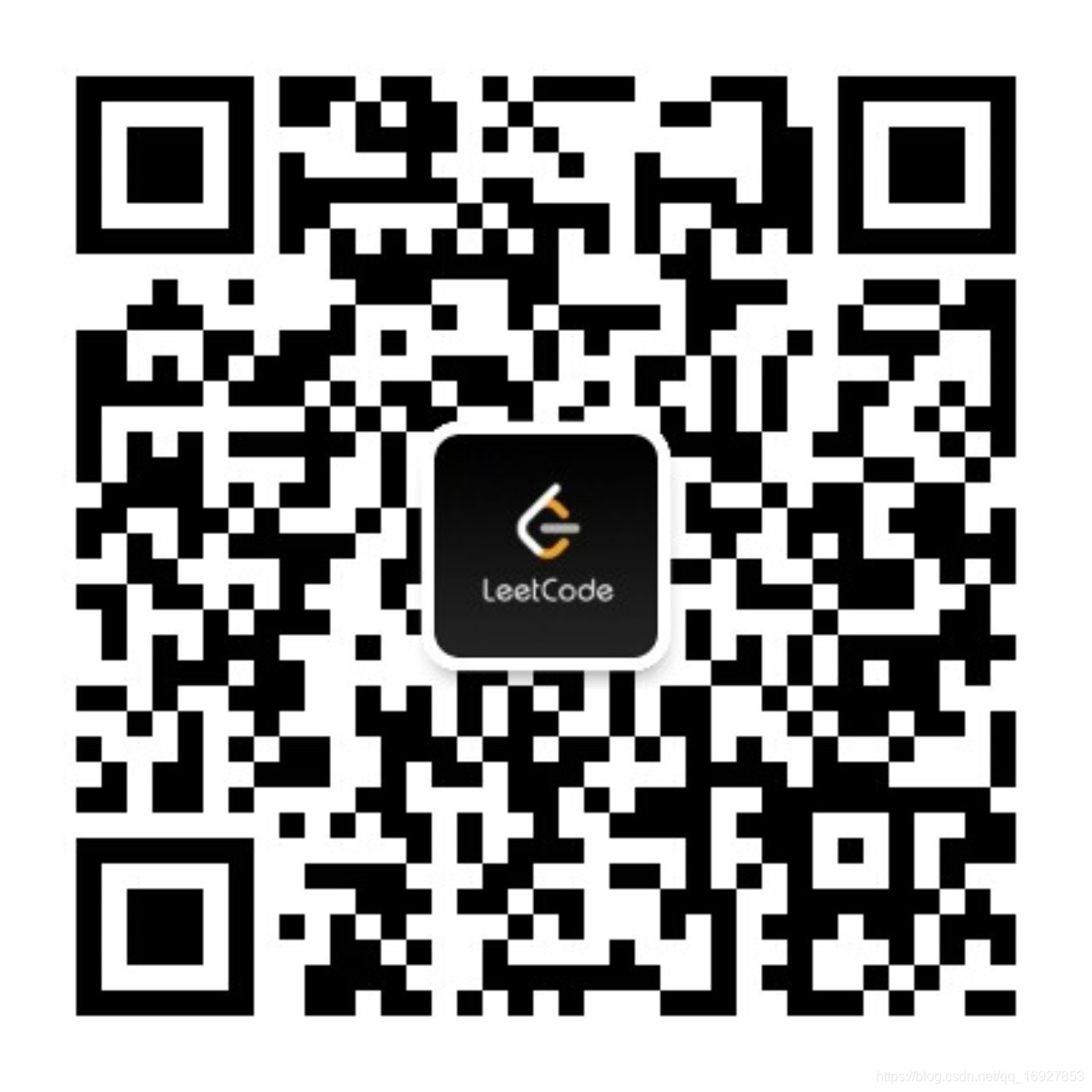