Background:
The value 30
is used as the upper bound of the loop because an integer in C++ has 32 bits
, and the highest bit is the sign bit, which is not used for
representing the magnitude of the number. Therefore, the largest positive number that can be represented with 31 bits is 2^30 - 1
. So, if the input number n is greater than or equal to 2^30
, then all the bits of n except the sign bit are already set to 1, and taking the bitwise complement will yield 0.
In other words, for any input value n
greater than or equal to 2^30
, the bitwise complement of n
is 0
. Therefore, there is no need to set any more bits in the mask, and the mask can be set to 0x7fffffff
, which is the largest positive integer that can be represented with 31 bits
.
If the input number n is less than 2^30
, then the loop will break before setting the mask to 0x7fffffff
, and the mask will be set to (1 << (highbit + 1)) - 1
, which is a bitmask with all bits set to 1 up to the position of the highest bit in n. This bitmask will be used to take the bitwise complement of n by XORing it with n.
The complement of an integer is the integer you get when you flip all the 0
’s to 1
’s and all the 1
’s to 0
’s in its binary representation.
- For example, The integer
5
is “101
” in binary and its complement is “010
” which is the integer2
.
Given an integern
, return its complement.
Example 1:
Input: n = 5
Output: 2
Explanation:
5 is "101" in binary, with complement "010" in binary, which is 2 in base-10.
Example 2:
Input: n = 7
Output: 0
Explanation:
7 is "111" in binary, with complement "000" in binary, which is 0 in base-10.
Example 3
:
Input: n = 10
Output: 5
Explanation:
10 is "1010" in binary, with complement "0101" in binary, which is 5 in base-10.
Constraints:
0 <= n < 109
Note:
- This question is the same as 476
AC:
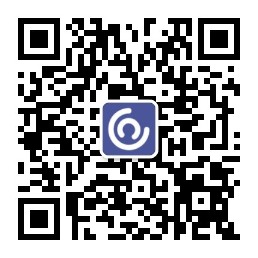
/*
* @lc app=leetcode.cn id=1009 lang=cpp
*
* [1009] 十进制整数的反码
*/
// @lc code=start
class Solution {
public:
int bitwiseComplement(int n) {
int highbit = 0;
// 寻找n的最高位
for(int i = 1; i <= 30; i++)
{
if(n >= (1 << i))
{
highbit = i;
}
else
{
break;
}
}
// 计算掩码
int mask = (highbit == 30 ? 0x7fffffff : (1 << (highbit + 1)) - 1);
// 返回n的按位补码
return n ^ mask;
}
};
// @lc code=end
Supplement:
0x7fffffff
是一个32位十六进制数,它的二进制表示为01111111111111111111111111111111
。这个二进制数是一个有符号32
位整数的最大正值。
在网络编程中,它通常用作一个掩码,用来屏蔽最高位,以便将一个有符号值转换为无符号值。0x7fffffff
是一个32位十六进制数,它的二进制表示为01111111111111111111111111111111
。这个二进制数是一个有符号32位整数的最大正值。在网络编程中,它通常用作一个掩码,用来屏蔽最高位,以便将一个有符号值转换为无符号值。
- 按位与(&)的使用技巧:
按位与操作是对两个二进制数进行位运算,只有当两个二进制数对应位都为1时,结果才为1,否则为0。对于C++
中的按位与操作符"&
",其使用技巧如下:
1)用按位与操作实现位的读取和修改:
使用按位与操作时,将要读取、修改的位数设为1,其余位数设为0,即可实现位的读取和修改。
例如,若要获取某个int
类型变量的第二位二进制数的值,可以使用以下代码:
int a = 0b100101;
int b = a & 0b10; // b = 0b10
若要将其修改为1,可以使用以下代码:
a |= 0b10; // a = 0b100111
2)用按位与操作实现多个二进制数的取交集:
使用按位与操作时,将多个二进制数进行按位与操作,即可取得它们的交集。
例如,若要求两个int类型变量a、b的二进制数的交集,可以使用以下代码:
int c = a & b;
- 按位或(|)的使用技巧:
按位或(|)操作是对两个二进制数进行位运算,只要两个二进制数对应位其中一个为1时,结果就为1,否则为0。对于C++中的按位或操作符"|",其使用技巧如下:
1)用按位或操作实现多个二进制数的取并集:
使用按位或操作时,将多个二进制数进行按位或操作,即可取得它们的并集。
例如,若要求两个int类型变量a、b的二进制数的并集,可以使用以下代码:
int c = a | b;
2)用按位或操作实现位的设置:
使用按位或操作时,将需要设置的位数设为1,其余位数设为0,即可实现位的设置。
例如,若要将某个int类型变量的第二位二进制数设置为1,可以使用以下代码:
int a = 0b100101;
a |= 0b10; // a = 0b1001111. 按位与(&)的使用技巧:
按位与操作是对两个二进制数进行位运算,只有当两个二进制数对应位都为1时,结果才为1,否则为0。对于C++
中的按位与操作符"&
",其使用技巧如下:
1)用按位与操作实现位的读取和修改:
使用按位与操作时,将要读取、修改的位数设为1,其余位数设为0,即可实现位的读取和修改。
例如,若要获取某个int类型变量的第二位二进制数的值,可以使用以下代码:
int a = 0b100101;
int b = a & 0b10; // b = 0b10
若要将其修改为1,可以使用以下代码:
a |= 0b10; // a = 0b100111
2)用按位与操作实现多个二进制数的取交集:
使用按位与操作时,将多个二进制数进行按位与操作,即可取得它们的交集。
例如,若要求两个int类型变量a、b的二进制数的交集,可以使用以下代码:
int c = a & b;
- 按位或(|)的使用技巧:
按位或(|)操作是对两个二进制数进行位运算,只要两个二进制数对应位其中一个为1时,结果就为1,否则为0。对于C++
中的按位或操作符"|
",其使用技巧如下:
1)用按位或操作实现多个二进制数的取并集:
使用按位或操作时,将多个二进制数进行按位或操作,即可取得它们的并集。
例如,若要求两个int类型变量a、b的二进制数的并集,可以使用以下代码:
int c = a | b;
2)用按位或操作实现位的设置:
使用按位或操作时,将需要设置的位数设为1,其余位数设为0,即可实现位的设置。
例如,若要将某个int类型变量的第二位二进制数设置为1,可以使用以下代码:
int a = 0b100101;
a |= 0b10; // a = 0b100111