STL中的队列
先进先出队列(FIFO)
主要方法
-
(1)
T front()
:访问队列的对头元素,并不删除对头元素 -
(2)
T back()
:访问队列的末尾元素,并不删除末尾元素 -
(3)
void pop()
:删除对头元素。 -
(4)
void push(T)
:元素入队
代码示例
#include <iostream>
#include <queue>
using namespace std;
int main()
{
std::queue<int> myqueue;
myqueue.push(1); //入队
myqueue.push(2);
myqueue.push(3);
cout<<"队列末尾元素:"<<myqueue.back( <<endl;
cout<<"队列元素出队顺序如下:";
while(!myqueue.empty()) //判空
{
cout<<myqueue.front()<<" "; //访问队列头元素
myqueue.pop(); //队列头元素出对
}
return 0;
}
输出
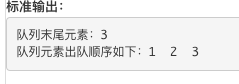
优先级队列
priority_queue
就是优先级队列在STL中的模版,在优先队列中,优先级高的元素先出队列,内部使用堆实现(大顶堆,同Top K问题)。
模版原型
p r i o r i t y _ q u e u e < T , S e q u e n c e , C o m p a r e > priority\_queue<T,Sequence,Compare> priority_queue<T,Sequence,Compare>
-
T T T : 存放容器的元素类型
-
S e q u e n c e Sequence Sequence : 实现优先级队列的底层容器,默认是
vector<T>
-
C o m p a r e Compare Compare : 用于实现优先级的比较函数,默认是functional中的
less<T>
初始化:
#include<bits/stdc++.h>
using namespace std;
int main(){
priority_queue<int,vector<int>,less<int>> maxx; // 大顶堆 数据元素从大往小进行排序
priority_queue<int,vector<int>,greater<int>> minn; //小顶堆 数据元素从小往大进行排序
}
主要对方法有
-
(1)
T top()
:访问队列的对头元素,并不删除对头元素 -
(2)
void pop()
:删除对头元素。 -
(3)
void push(T)
:元素入队
代码示例
int的大顶堆
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<int> mypq;
mypq.push(2); //入队
mypq.push(4);
mypq.push(1);
mypq.push(8);
cout << "Popping out elements...";
while (!mypq.empty())
{
cout << ' ' << mypq.top(); //读取队列头元素
mypq.pop(); //对头元素出队列
}
cout << '\n';
return 0;
}
运行结果
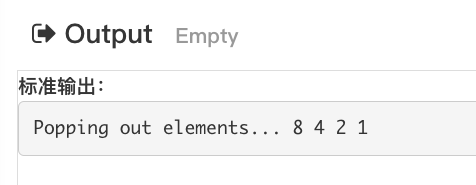
int的小顶堆
#include <iostream>
#include <queue>
using namespace std;
int main()
{
priority_queue<int,std::vector<int>,greater<int>> mypq;
mypq.push(2); //入队
mypq.push(4);
mypq.push(1);
mypq.push(8);
cout << "Popping out elements...";
while (!mypq.empty())
{
cout << ' ' << mypq.top(); //读取队列头元素
mypq.pop(); //对头元素出队列
}
cout << '\n';
return 0;
}
运行结果
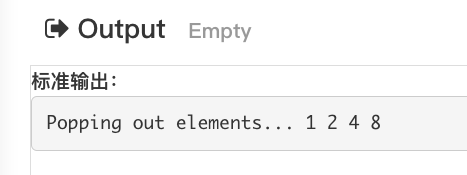
使用自定义的比较函数的优先队列
标准库默认使用元素类型的<操作符
来确定它们之间的优先级关系,大的优先级高,优先输出。
我们只要重写<操作符就可以啦。
代码示例
#include <bits/stdc++.h>
using namespace std;
typedef long long ll;
struct Student
{
string name;
int score;
};
class cmp{
public:
bool operator()(Student &a, Student &b){
return a.score<b.score; //成绩高者优先
}
};
void xianshi(Student a){
cout<<"成绩最高者为:"<<a.name<<' '<<a.score<<endl;
}
int main()
{
priority_queue<Student,vector<Student>,cmp> sq;
// 利用优先队列可以快速的获得自己想要的优先级最高的数据
Student stu1,stu2,stu3,stu4;
stu1.name = "小明"; stu1.score = 59;
sq.push(stu1);
xianshi(sq.top());
stu2.name = "小花"; stu2.score = 88;
sq.push(stu2);
xianshi(sq.top());
stu3.name = "小黑"; stu3.score = 99;
sq.push(stu3);
xianshi(sq.top());
stu4.name = "Bob"; stu4.score = 55;
sq.push(stu4);
xianshi(sq.top());
return 0;
}
运行结果
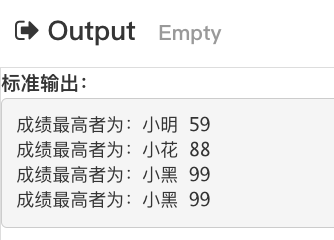
双端队列
双端队列就是一个两端都是结尾的队列。队列的每一端都可以插入数据项和移除数据项。deque是STL中双端队列模版。
主要方法
-
(1)
void pop_front()
:从队列头部删除元素 -
(2)
void pop_back()
:从队列删除元素 -
(3)
void push_front(T)
:从队列头部插入元素 -
(4)
void push_back(T)
:从队列尾部插入元素 -
(5)
T front()
:读取队列头部元素 -
(6)
T back(T)
:读取队列尾部元素
示例
#include <iostream>
#include <deque>
using namespace std;
int main ()
{
deque<int> mydeque;
mydeque.push_back (2); //队列尾部插入
mydeque.push_back (4);
mydeque.push_back (3);
mydeque.push_front(1); //队列头部插入
cout << "Popping out the elements in mydeque:";
while (!mydeque.empty())
{
cout << ' ' << mydeque.front();
mydeque.pop_front(); //删除队列头部
}
cout << "\nThe final size of mydeque is " << int(mydeque.size()) << '\n';
return 0;
}
运行结果
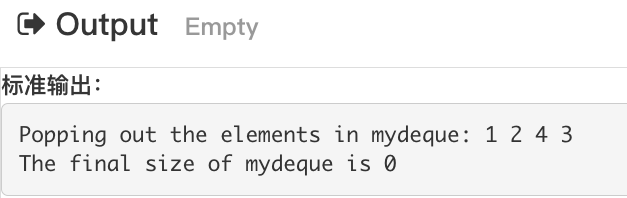
有关双端队列的题目
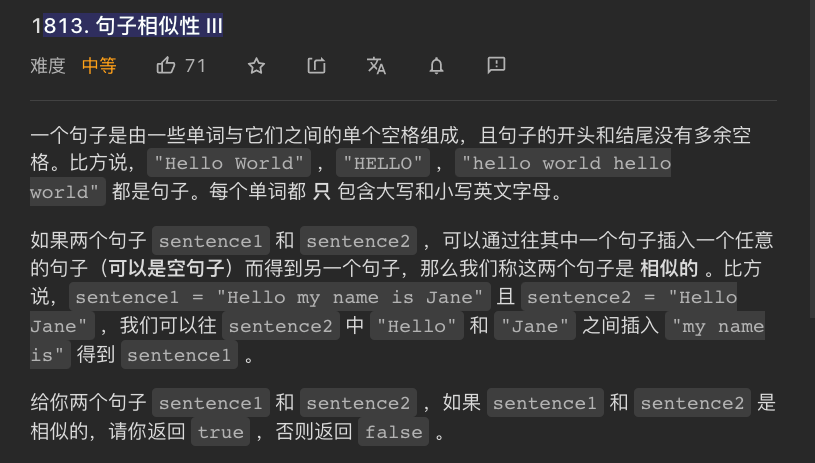
class Solution {
public:
bool areSentencesSimilar(string sentence1, string sentence2) {
deque<string> s1,s2;
stringstream ss1(sentence1);
stringstream ss2(sentence2);
string t;
while(ss1>>t){
s1.push_back(t);}
while(ss2>>t){
s2.push_back(t);}
bool ans = 0;
while(1){
bool f1 = 1,f2 = 1;
if(s1.empty()==1||s2.empty()==1){
ans = 1;break;
}
if(s1[0]==s2[0]){
s1.pop_front();
s2.pop_front();
}else{
f1 = 0;}
if(s1.empty()==1||s2.empty()==1){
ans = 1;break;
}
if(s1[s1.size()-1]==s2[s2.size()-1]){
s1.pop_back();
s2.pop_back();
}else{
f2 = 0;}
if(f1==0&&f2==0){
ans = 0;break;
}
}
return ans;
}
};