一、Jedis
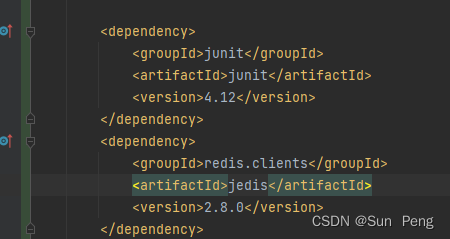
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>2.8.0</version>
</dependency>
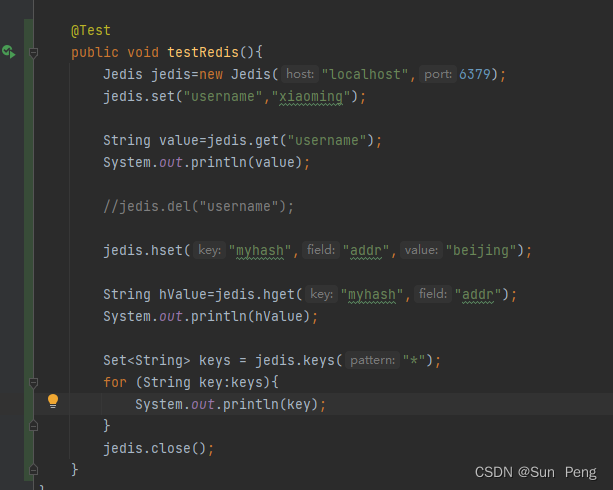
二、Spring Data Redis(常用)
【1】pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
【2】application.yml
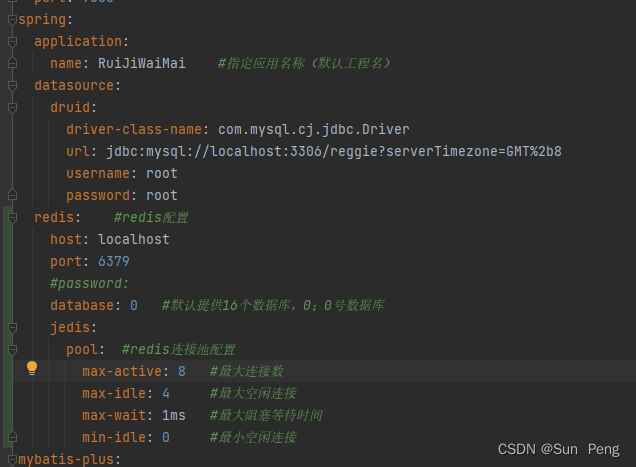
spring:
redis:
host: localhost
port: 6379
database: 0
jedis:
pool:
max-active: 8
max-idle: 4
max-wait: 1ms
min-idle: 0
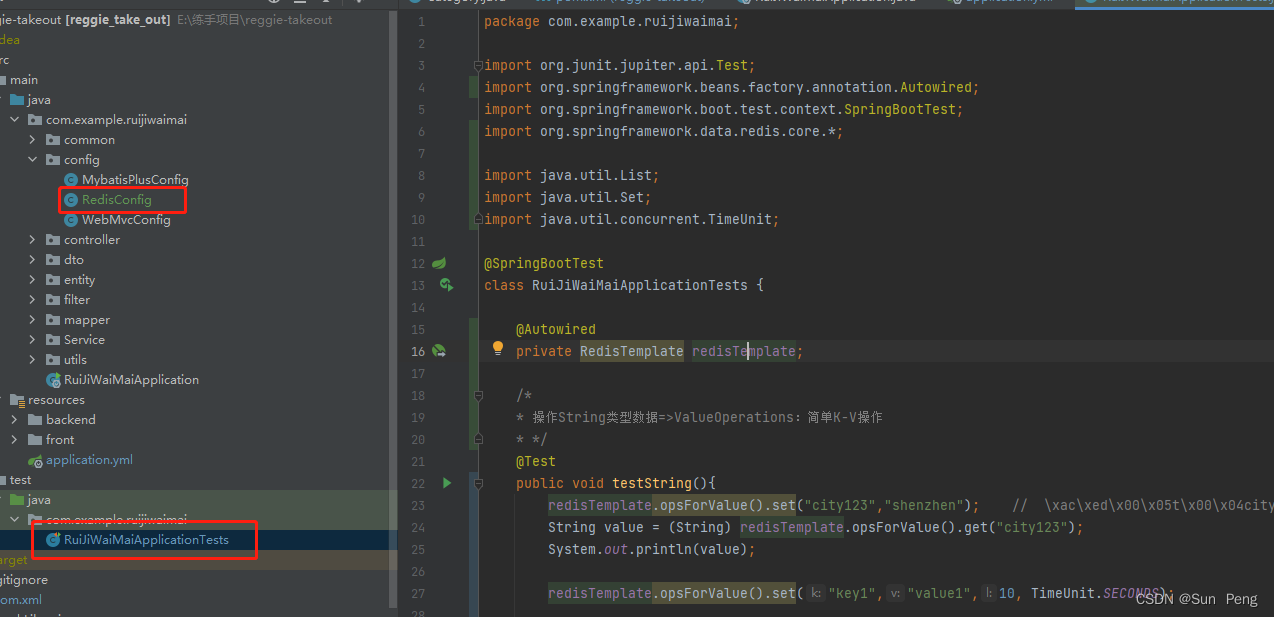
【3】RedisConfig
package com.example.ruijiwaimai.config;
import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisTemplate<Object,Object> redisTemplate(RedisConnectionFactory connectionFactory){
RedisTemplate<Object,Object> redisTemplate=new RedisTemplate<>();
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setHashKeySerializer(new StringRedisSerializer());
redisTemplate.setConnectionFactory(connectionFactory);
return redisTemplate;
}
}
【4】RuiJiWaiMaiApplicationTests
package com.example.ruijiwaimai;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.connection.DataType;
import org.springframework.data.redis.core.*;
import java.util.List;
import java.util.Set;
import java.util.concurrent.TimeUnit;
@SpringBootTest
class RuiJiWaiMaiApplicationTests {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void testString() {
redisTemplate.opsForValue().set("city123", "shenzhen");
String value = (String) redisTemplate.opsForValue().get("city123");
System.out.println(value);
redisTemplate.opsForValue().set("key1", "value1", 10, TimeUnit.SECONDS);
Boolean aBoolean = redisTemplate.opsForValue().setIfAbsent("city1234", "nanjing");
System.out.println(aBoolean);
}
@Test
public void testHash() {
HashOperations hashOperations = redisTemplate.opsForHash();
hashOperations.put("002", "name", "xiaoming");
hashOperations.put("002", "age", "20");
String age = (String) hashOperations.get("002", "age");
System.out.println(age);
Set keys = hashOperations.keys("002");
for (Object key : keys) {
System.out.println(key);
}
List values = hashOperations.values("002");
for (Object value : values) {
System.out.println(value);
}
}
@Test
public void testList() {
ListOperations listOperations = redisTemplate.opsForList();
listOperations.leftPush("mylist", "a");
listOperations.leftPushAll("mylist", "b", "c", "d");
List<String> mylist = listOperations.range("mylist", 0, -1);
for (String value : mylist) {
System.out.println(value);
}
Long size = listOperations.size("mylist");
int lSize = size.intValue();
for (int i = 0; i < lSize; i++) {
Object element = listOperations.rightPop("mylist");
System.out.println("出队列:" + element);
}
}
@Test
public void testSet() {
SetOperations setOperations = redisTemplate.opsForSet();
setOperations.add("myset", "a", "b", "c", "a");
Set<String> myset = setOperations.members("myset");
for (String o : myset) {
System.out.println(o);
}
setOperations.remove("myset", "a", "b");
myset = setOperations.members("myset");
for (String o : myset) {
System.out.println("删除后的数据:" + o);
}
}
@Test
public void testZSet() {
ZSetOperations zSetOperations = redisTemplate.opsForZSet();
zSetOperations.add("myZset", "a", 10.0);
zSetOperations.add("myZset", "b", 11.0);
zSetOperations.add("myZset", "c", 12.0);
zSetOperations.add("myZset", "a", 13.0);
Set<String> myZet = zSetOperations.range("myZset", 0, -1);
for (String s : myZet) {
System.out.println(s);
}
zSetOperations.incrementScore("myZset", "b", 20.0);
myZet = zSetOperations.range("myZset", 0, -1);
for (String s : myZet) {
System.out.println("修改分数: " + s);
}
zSetOperations.remove("myZset", "a", "b");
myZet = zSetOperations.range("myZset", 0, -1);
for (String s : myZet) {
System.out.println("删除后的成员: " + s);
}
}
@Test
public void testCommon() {
Set keys = redisTemplate.keys("*");
for (Object key : keys) {
System.out.println(key);
}
Boolean itcast = redisTemplate.hasKey("itcast");
System.out.println("判断某个key是否存在:"+itcast);
redisTemplate.delete("myZset");
DataType dataType = redisTemplate.type("001");
System.out.println("获取指定key对应的value的数据类型:"+dataType.name());
}
}
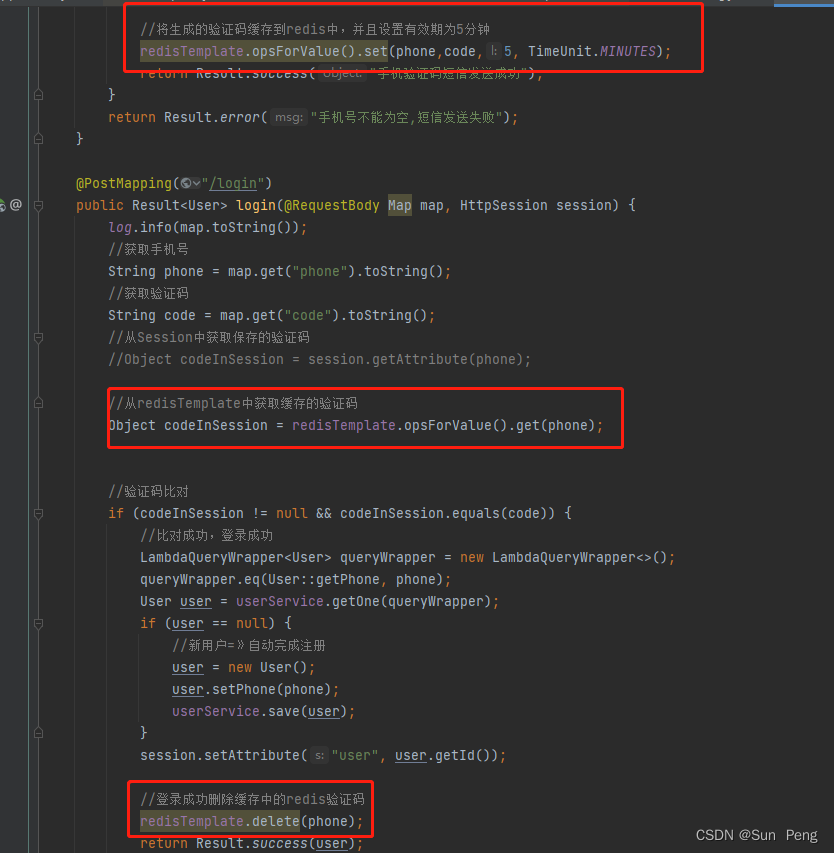
三、Spring Cache
【1】常用注解:
注解 |
说明 |
@EnableCaching |
开启缓存注解功能 |
@Cacheable |
判断是否有缓存数据,有=》返回缓存数据;没有=》放到缓存中 |
@CachePut |
将方法的返回值放到缓存中 |
@CacheEvict |
将一条或多条数据从缓存中删除 |
【2】使用案例
package com.itheima.controller;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.itheima.entity.User;
import com.itheima.service.UserService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("/user")
@Slf4j
public class UserController {
@Autowired
private CacheManager cacheManager;
@Autowired
private UserService userService;
@CachePut(value = "userCache",key = "#user.id")
@PostMapping
public User save(User user){
userService.save(user);
return user;
}
@CacheEvict(value = "userCache",key = "#p0")
@DeleteMapping("/{id}")
public void delete(@PathVariable Long id){
userService.removeById(id);
}
@CacheEvict(value = "userCache",key = "#result.id")
@PutMapping
public User update(User user){
userService.updateById(user);
return user;
}
@Cacheable(value = "userCache",key = "#id",unless = "#result == null")
@GetMapping("/{id}")
public User getById(@PathVariable Long id){
User user = userService.getById(id);
return user;
}
@Cacheable(value = "userCache",key = "#user.id + '_' + #user.name")
@GetMapping("/list")
public List<User> list(User user){
LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(user.getId() != null,User::getId,user.getId());
queryWrapper.eq(user.getName() != null,User::getName,user.getName());
List<User> list = userService.list(queryWrapper);
return list;
}
}
【3】底层不使用redis,重启服务,内存丢失=>解决:
pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
application.yml
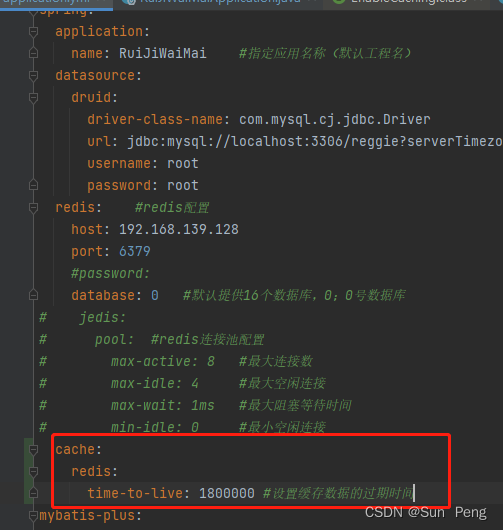
redis:
host: 192.168.139.128
port: 6379
database: 0
cache:
redis:
time-to-live: 1800000
启动类:
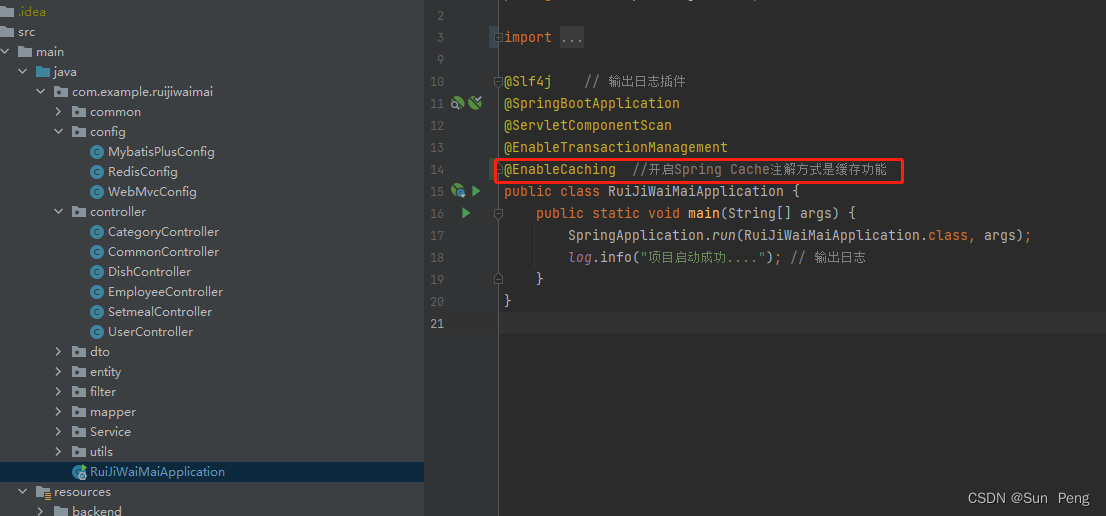
Result:

注解使用:
@PostMapping()
@CacheEvict(value = "setmealCache",allEntries = true)
public Result<String> save(@RequestBody SetmealDto setmealDto) {
log.info("套餐信息:{}", setmealDto);
setmealService.saveWithDish(setmealDto);
return null;
}
@DeleteMapping()
@CacheEvict(value = "setmealCache",allEntries = true)
public Result<String> delete(@RequestParam List<Long> ids){
setmealService.removeWithDish(ids);
return Result.success("套餐数据删除成功");
}
@GetMapping("/list")
@Cacheable(value = "setmealCache",key = "#setmeal.categoryId + '_' + #setmeal.status")
public Result<List<Setmeal>> list(Setmeal setmeal){
LambdaQueryWrapper<Setmeal> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(setmeal.getCategoryId() != null,Setmeal::getCategoryId,setmeal.getCategoryId());
queryWrapper.eq(setmeal.getStatus() != null,Setmeal::getStatus,setmeal.getStatus());
queryWrapper.orderByDesc(Setmeal::getUpdateTime);
List<Setmeal> list = setmealService.list(queryWrapper);
return Result.success(list);
}