委托
一般委托
小理解
怎么样才能把需要存储Calculator类的成员函数到一个变量中去,int(数据类型)num=12;Calculator(数据类型) method=方法;
委托是一个类,它定义了方法的类型,该类型用于存储方法。
语法:访问修饰符 delegate 返回值;类型 委托名(参数列表);如果不声明访问修饰符默认是public。
练习
//定义计算机类
class Calculator
{//无返回值无参数
public void Report()
{
Console.WriteLine("No report");
}
public int Abs(int v)
{
return Math.Abs(v);//0<=x<=value
}
}
//定义无返回值无参数的委托
public delegate void NoRetParFun();
//定义有返回值有参数的委托
public delegate int RetParfun(int v);
internal class Program
{
//定义一个无返回值无参数的函数
public static void Print()
{
Console.WriteLine("program.print");
}
static void Main(string[] args)
{
Calculator calculator = new Calculator();
//创建无返回值的委托类型对象
NoRetParFun noRetParFun01 = new NoRetParFun(calculator.Report);//把计算机类里的report方法传入noretparfun01里面
//调用方式一
noRetParFun01();
//创建委托类型对象
NoRetParFun noRetParFun02=calculator.Report;//把计算机类里的report方法传入noretparfun02里面
//调用方法二
noRetParFun02.Invoke();
//创建有返回值的委托类型
RetParfun retparfun =calculator.Abs;
Console.WriteLine(retparfun(-10));//10
Console.WriteLine(retparfun.Invoke(-20));//20
Console.WriteLine("-----");
//应用,可以进行函数的运算
noRetParFun01 += Print;
noRetParFun01();
Console.WriteLine("----");
noRetParFun01 -= calculator.Report;
noRetParFun01.Invoke();
//验证委托是一个;类
Console.WriteLine("------");
Console.WriteLine(typeof(Calculator).IsClass);//true
Console.WriteLine(typeof(int).IsClass);//false
Console.WriteLine(typeof(NoRetParFun).IsClass);//true
Console.WriteLine(typeof(RetParfun).IsClass);//true
Console.ReadKey();
系统委托
Action
//无返回值无参数
public static void Print() {
Console.WriteLine("print.");
}
//无返回值,有参数
public static void Say(string name)
{
Console.WriteLine($"{name}-");
}
//系统委托就是系统定义好的委托,可以直接使用
//action系统委托是没有返回值可以有参数列表的委托
//public delegate void Action();
//public delegate void Action<in T>(T obj);
Action action01 = Print;
action01();
Action<string> action02 = Say;
action02.Invoke("peter");
Func
//有返回值,无参数
public static string Hellow()
{
return "hellow";
}
//有返回值有参数
public static string Join(bool b,int num)
{
return b + "-" + num;
}
//Func系统委托是有参数有返回值的委托
//public delegate Tresult Func<out Tresult>();
//public delegate Tresult func<in T1,in T2,out Tresult>(T1 arg1,T2 srg2);
Func<string> func = Hellow;
//只是返回值,需要打印,所以。。。
Console.WriteLine(func());
Func<bool,int,string> func01=Join;
Console.WriteLine(func01.Invoke(false,1));
事件
小理解
事件是基于委托的,是委托的一种安全包裹,简单的理解为事件一种更加安全的委托
语法:访问修饰符 event 委托类型 事件名;
特点:事件的初始化必须在定义事件的类中进行,事件的调用必须在定义事件的类中进行,在其他类中可以进行+=,-=操作。
练习
class Text {
//普通委托字段
public Action action01;
//事件(安全委托)
public event Action action02 = Program.Print;
public void CallEvent()
{
action02();
}
}
internal class Program
{
public static void Print()
{
Console.WriteLine("program.print");
}
public static void Show()
{
Console.WriteLine("program.show");
}
static void Main(string[] args)
{
Text text = new Text();
//普通委托
text.action01 = Print;
text.action01();
//事件
Text text2 = new Text();
//报错只能进行+=,事件不能在其他类中进行初始化
/*text2.action02 = Print*/;
//报错只能进行+=,事件不能在其他类中进行调用
//text2.action02();
//只能通过定义事件类中的函数进行事件的间接调用
text2.CallEvent();
Console.WriteLine("------");
text2.action02 += Show;
text2.CallEvent();
text2.action02 -= Show;
text2.CallEvent();
Console.ReadKey();
匿名函数
小理解
定义:匿名函数就是没有名字的函数,主要配合委托和事件一起使用;没有委托和事件匿名函数就没有意义。
作用:定义匿名函数的主要作用是进行赋值。
练习
//一个普通委托调用
public static Action action = Print;
public static void Print()
{
Console.WriteLine("print");
}
static void Main(string[] args)
{
action();
Console.ReadKey();
//匿名函数的
public static Action action = delegate(){
Console.WriteLine("匿名的");
};
static void Main(string[] args)
{
action();
Console.ReadKey();
}
//定义一个action委托参数列表,用匿名函数
public static Action<string, int> GetAction = delegate (string s, int num)
{
Console.WriteLine($"{ s}-{num}-");
};
static void Main(string[] args)
{
GetAction("peter", 12);
Console.ReadKey();
//定义一个事件,用匿名函数
public static event Func<int, string> func = delegate (int num)
{
return num + "";
};
static void Main(string[] args)
{
Console.WriteLine(func(12));
//查看类型
Console.WriteLine(func(12).GetType());
Console.ReadKey();
//匿名函数还可以进行参数传参
public static void Test(Action action)
{
action();
}
static void Main(string[] args)
{
Test(delegate ()
{
Console.WriteLine("匿名函数还可以进行参数传参");
});
Lambda表达式
小理解
Lambda表达式是一种用于简化匿名函数定义的表达式。
作用:简化匿名表达式函数的定义
语法:(参数列表)=>函数体{}
要点:函数体只有一条语句可以省略{},函数只有一条语句且是return语句的时候{}和return都可以省略,属性。
练习
//属性的时候
private string name;
public string Name{get=>name;set=>name=value;}
//
Test(()=> Console.WriteLine("匿名函数还可以进行参数传参"));
//
public static event Func<int, string> func =(int num)=>num + "";
多线程
小理解
多线程是给程序开辟多个执行的路径使得程序可以同时执行不同的业务逻辑
语法:创建Thread类对象(对象),业务逻辑函数(路径中要执行的业务逻辑),启动线程
练习
//创建线程对象1
Thread thread = new Thread(LiFile);
thread.Start();
//创建线程对象2
Thread thread01 = new Thread(FilFile);
thread01.Start();
//主线程
int second = 20;
string name = "主线程";
while (true)
{
Thread.Sleep(1 * 1000);
Console.WriteLine($"{name}-{second--}--");
if (second == 0)
{
Console.WriteLine("主线程结束喽");
return;
}
}
}
//扫描文件线程
public static void LiFile() {
int second = 15;
string name = "文件喽";
while (true)
{
Thread.Sleep(1*1000);
Console.WriteLine($"{name}-扫描开始了-{second--}");
if (second == 0) {
Console.WriteLine($"扫描停止了{name}");
return;
}
}
}
public static void FilFile()
{
int second = 10;
string name = "fil文件喽";
while (true)
{
Thread.Sleep(1 * 1000);
Console.WriteLine($"{name}-扫描开始了-{second--}");
if (second == 0)
{
Console.WriteLine($"扫描停止了{name}");
return;
}
}
}
}
}
迭代器
小理解
IEnumerator该接口就是一个迭代器,主要提供了可以用使用foreach进行循环遍历容器的相关方法。IEnumerable该接口主要用于对外可以使用的迭代器。
练习
//首先定义一个集合类用foreach进行迭代
class CustomArray<T>: IEnumerable,IEnumerator//IEnumerable报错?需要导入using System.Collections;再导入接口
{
private T[] array;//想要存储任意数据类型,改用什么,泛型
public CustomArray(T[] array)
{
this.array = array;
}
//定义一个索引值
public int index = -1;
//IEnumerator提供的用于返回当前数据的属性
object IEnumerator.Current =>this.array[index];//lambda表达式
//IEnumerable提供的可以用于返回迭代器的方法
public IEnumerator GetEnumerator()
{
return this;
}
//IEnumerator提供的用于移动索引的方法
bool IEnumerator.MoveNext()
{
index++;
return this.index < this.array.Length;
}
//IEnumerator提供的用于复位索引的方法
void IEnumerator.Reset()
{
this.index = -1;
}
}
internal class Program
{
static void Main(string[] args)
{
CustomArray<int> customArray=new CustomArray<int>(new int[] { 1, 2, 3 ,4});
foreach (int item in customArray)//报错?不包含GetEnumerator的公共实例或扩展定义
{
Console.WriteLine(item);
}
CustomArray<string> customArray01 = new CustomArray<string>(new string[] { "a", "b", "c", "d" });
foreach (string item in customArray01)//报错?不包含GetEnumerator的公共实例或扩展定义
{
Console.WriteLine(item);
}
Console.ReadKey();
//步骤,太多,简化一些
//不使用IEnumerator接口
public IEnumerator GetEnumerator()
{
//直接写for循环
for (int i = 0; i < this.array.Length; i++)
{
yield return this.array[i];//这是就需要yield
}
}
//自己定义了一个类里面的方法,只不过和IEnumerable同名的方法
public IEnumerator GetEnumerator()
{
//直接写for循环
for (int i = 0; i < this.array.Length; i++)
{
yield return this.array[i];//这是就需要yield
}
}
视图
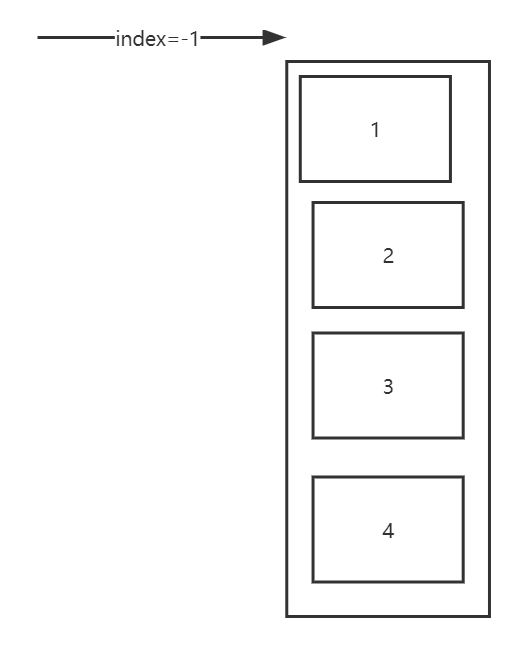
var
练习
//该数据类型定义的变量可以存储任何类型的数据
int[] nums=new int[]{2,3,4,5}
foreach(var item in nums){
Console.WriteLine(item);}
//var?
var data="peer";
Console.WriteLine(data is string);
//一旦赋值就不能在赋值其他数据类型的值
//var只能修饰局部变量不能修饰成员变量;
元组
练习
//元组就是计算机用于存储数据的一种简化格式
//语法:(数据类型 字段名,数据类型 字段名。。。) 元组名=(值,值)
(double a, int b) t1 = (12.3, 2);
Console.WriteLine($"a={t1.a},b={t1.b}");
//定义一个匿名数组,那么怎么显示值呢
(string, int) t2 = ("peter", 29);
Console.WriteLine($"{t2.Item1}-{t2.Item2}");//important
//定义一些其他格式呢
var t3 = (ID: 11, Name: "jack", Data: 12);
Console.WriteLine($"id={t3.ID},naem={t3.Name},data={t3.Data}");
//匿名元组
var t4 = (11, "lucky", 14);
Console.WriteLine($"id:{t4.Item1},name={t4.Item2},data={t4.Item3}");
Console.WriteLine(MinAndMax<int>(new int[] { 1, 2, 20, 10 }));
Console.WriteLine(MinAndMax<int>(new int[] { 1, 11, 22 }).Min);
Console.WriteLine(MinAndMax<int>(new int[] { 1, 11, 22 }).Max);
//元组是值类型还是引用类型
(int,int) a=(1,11);
(short,short) b=(1,11);
Console.WriteLine(a == b);
Console.WriteLine(b != a);
var c = b;
c = (20, 11);
Console.WriteLine(c);
Console.WriteLine(b);
Console.ReadKey();
}
//定义一个函数返回数组中的最小值和最大值(1,10)
public static (double Min,double Max) MinAndMax<T>(T[] array)
{
return (Min: 10, Max: 20);
}
}
{}和?
练习
class Player {
public int id;
public string name;
}
static void Main(string[] args)
{
//{}可以用来初始化对象
//数组的形式
int[] nums = { 1, 2, 3, 4, 5, };
Console.WriteLine(nums[1]);
//集合的形式
List<string> list = new List<string>() { "peter","lucky","banner"};
Console.WriteLine(list[1]);
//对象的形式
Player player = new Player() { id=11,name="lucjy"};
Console.WriteLine($"id={player.id},name={player.name}");
//自定义对象
var obj = new { id = 111, name = "lucky", data = 11 };
Console.WriteLine($"id={obj.id},naem={obj.name},data={obj.name}");
//?关于值类型,代表该值类型变量可以存储null值
int? data = null;
Console.WriteLine(data == null);//true
Console.WriteLine(data != null);//false
Console.WriteLine(data.HasValue);//false
data = 11;
Console.WriteLine(data.HasValue);//true
//引用变量?代表的是判断不为null后调用相关方法
Player player1 = null;
//判断不为null在调用其方法
player1?.ToString();
string[] name = null;
//判断不为null后再访问下标
Console.WriteLine(name?[3]);
Console.ReadKey();