一、语法介绍
1、什么是面向对象编程
2、什么是类
3、类的定义
4、类中字段和方法的声明
扫描二维码关注公众号,回复:
1661457 查看本文章
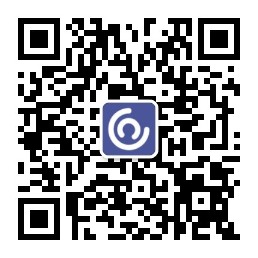
5、构造函数
6、属性的定义
二、语法实践
//工具类 using System; namespace 面向对象编程 { /// <summary> /// 顾客类 /// </summary> class Customer { //数据成员 private string name; private string addreess; private int age; private string buyTime; private int price; public string telNum; private float x; private float y; private float z; /// <summary> /// 无参构造函数 /// </summary> public Customer() { Console.WriteLine("调用了无参构造函数"); } /// <summary> /// 带参构造函数 /// </summary> /// <param name="x"></param> /// <param name="y"></param> /// <param name="z"></param> public Customer(float x, float y, float z) { this.x = x; this.y = y; this.z = z; Console.WriteLine("调用了带参构造函数,初始化赋值 x=" + x + " y=" + y + " z=" + z); } //通过接收外部传值来修改内部数据 public void SetName(string name) { this.name = name; } public void SetAddress(string address) { this.addreess = address; } public void SetAge(int age) { this.age = age; } public void SetBuyTime(string buyTime) { this.buyTime = buyTime; } public void SetPrice(int price) { this.price = price; } //输出顾客的购买信息 public void Show() { Console.WriteLine("姓名:" + name); Console.WriteLine("地址:" + addreess); Console.WriteLine("年龄:" + age); Console.WriteLine("购买时间:" + buyTime); Console.WriteLine("价格:" + price); Console.WriteLine("电话号码:" + telNum); } private int num; /// <summary> /// 属性封装 get 和 set前面也可以添加访问修饰符来控制属性的公开性 /// </summary> public int Num { get { Console.WriteLine("调用属性的get块值:" + num); return num; } set { Console.WriteLine("设置属性的set块值:" + value); num = value; } } /// <summary> /// 简写属性封装,编译器会自动补全 /// </summary> public int Count { get; set; } } }
//实现类 using System; namespace 面向对象编程 { class Program { static void Main(string[] args) { //声明要使用的类,使用关键字new调用无参构造函数初始化 Customer custom = new Customer(); //传值修改类内部数据 custom.SetName("王豆豆"); custom.SetAddress("月球"); custom.SetAge(18); custom.SetBuyTime(DateTime.Now.ToString()); custom.SetPrice(-1000); //直接赋值修改类公开的数据 custom.telNum = "10101010110"; //调用类的显示方法 custom.Show(); //声明要使用的类,使用关键字new调用带参构造函数 Customer custom1 = new Customer(3, 4, 5); custom1.Num = 100;//set设置属性 int tempNum = custom1.Num;//get获取属性 //匿名类型 var:未初始化之前可以是任何类型,初始化之后类型确定 var a = 10; var b = ""; var c = new int[10]; Console.ReadKey(); } } }