一、前期准备
案例效果图
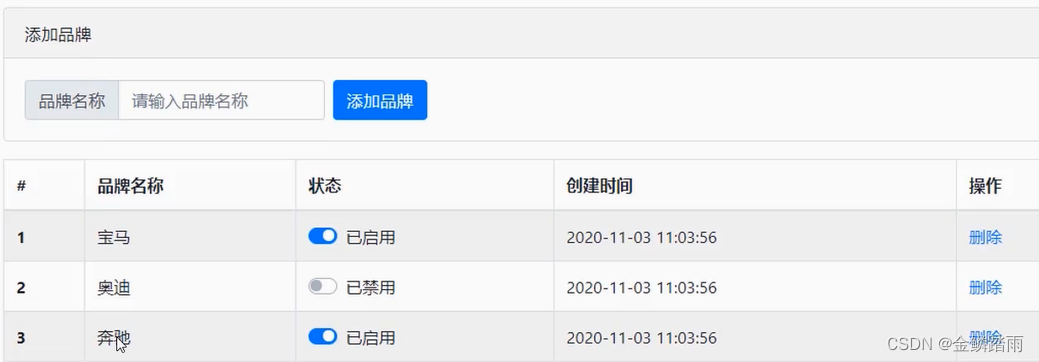
技术点分析
使用bootstrap4.x相关的技术,例如:卡片、表单、按钮、表格。
Vue指令与过滤器相关的知识点。
二、案例实现
实现步骤
创建基本的 vue 实例
基于 vue 渲染表格数据
实现添加品牌的功能
实现删除品牌的功能
实现修改品牌状态的功能
代码实现
完整代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8"/>
<meta content="width=device-width, initial-scale=1.0" name="viewport"/>
<title>Document</title>
<link href="../../lib/bootstrap.css" rel="stylesheet"/>
<style>
:root {
font-size: 13px;
}
body {
padding: 8px;
}
</style>
</head>
<body>
<div id="app">
<!-- 卡片区域 -->
<div class="card">
<h5 class="card-header">添加品牌</h5>
<div class="card-body">
<!-- 添加品牌的表单 -->
<form @submit.prevent class="form-inline">
<div class="input-group mb-2 mr-sm-2">
<div class="input-group-prepend">
<div class="input-group-text">品牌名称</div>
</div>
<!-- 文本输入框 -->
<input @keyup.esc="brandname = ''" class="form-control" type="text" v-model.trim="brandname"/>
</div>
<!-- 提交表单的按钮 -->
<button @click="addNewBrand" class="btn btn-primary mb-2" type="submit">添加品牌</button>
</form>
</div>
</div>
<!-- 品牌列表 -->
<table class="table table-bordered table-striped mt-2">
<thead>
<tr>
<th>#</th>
<th>品牌名称</th>
<th>状态</th>
<th>创建时间</th>
<th>操作</th>
</tr>
</thead>
<!-- 表格的主体区域 -->
<tbody>
<!-- 循环渲染表格的每一行数据 -->
<tr :key="item.id" v-for="(item, index) in brandlist">
<td>{
{index + 1}}</td>
<td>{
{item.brandname}}</td>
<td>
<div class="custom-control custom-switch">
<input :id="item.id" class="custom-control-input" type="checkbox" v-model="item.state"/>
<label :for="item.id" class="custom-control-label" v-if="item.state">已启用</label>
<label :for="item.id" class="custom-control-label" v-else>已禁用</label>
</div>
</td>
<td>{
{item.addtime | dateFormat}}</td>
<td>
<a @click.prevent="removeBrand(item.id)" href="#">删除</a>
</td>
</tr>
</tbody>
</table>
</div>
<script src="../../lib/vue-2.6.12.js"></script>
<script>
// 创建全局的过滤器
Vue.filter('dateFormat', (dateStr) => {
const dt = new Date(dateStr)
const y = dt.getFullYear()
const m = padZero(dt.getMonth() + 1)
const d = padZero(dt.getDate())
const hh = padZero(dt.getHours())
const mm = padZero(dt.getMinutes())
const ss = padZero(dt.getSeconds())
return `${y}-${m}-${d} ${hh}:${mm}:${ss}`
})
// 补零的函数
function padZero(n) {
return n > 9 ? n : '0' + n
}
// 创建 vue 的实例对象
const vm = new Vue({
el: '#app',
data: {
brandname: '',
nextId: 4,
brandlist: [
{id: 1, brandname: '宝马', state: true, addtime: new Date()},
{id: 2, brandname: '奥迪', state: false, addtime: new Date()},
{id: 3, brandname: '奔驰', state: true, addtime: new Date()},
],
},
methods: {
// 添加新的品牌数据
addNewBrand() {
// 判断品牌名称是否为空
if (!this.brandname) return alert('品牌名称不能为空!')
// 添加新的品牌数据
this.brandlist.push({
id: this.nextId,
brandname: this.brandname,
state: true,
addtime: new Date(),
})
// 重置文本框的值
this.brandname = ''
// 让 nextId 自增 +1
this.nextId++
},
// 根据 Id 删除对应的数据
removeBrand(id) {
this.brandlist = this.brandlist.filter((x) => x.id !== id)
},
},
})
</script>
</body>
</html>
运行结果
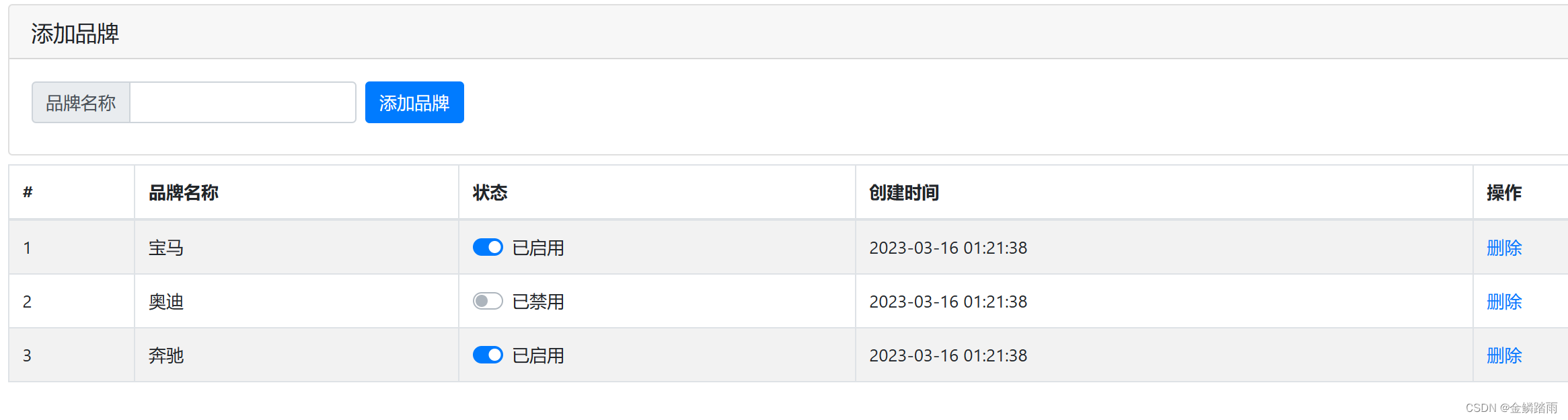
功能点分析
使用bs4主键设置“状态”样式
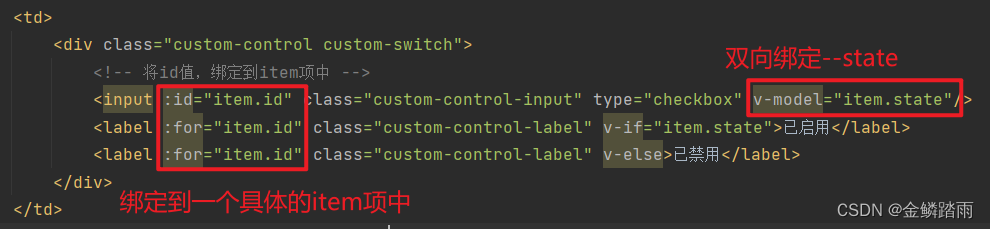
使用全局过滤器对时间进行格式化
<td>{
{item.addtime | dateFormat}}</td>
定义处理函数dateFormat,处理时间字符串
添加品牌
监听 input 输入框的 keyup事件,通过 .esc 按键修饰符快速清空文本框中的内容
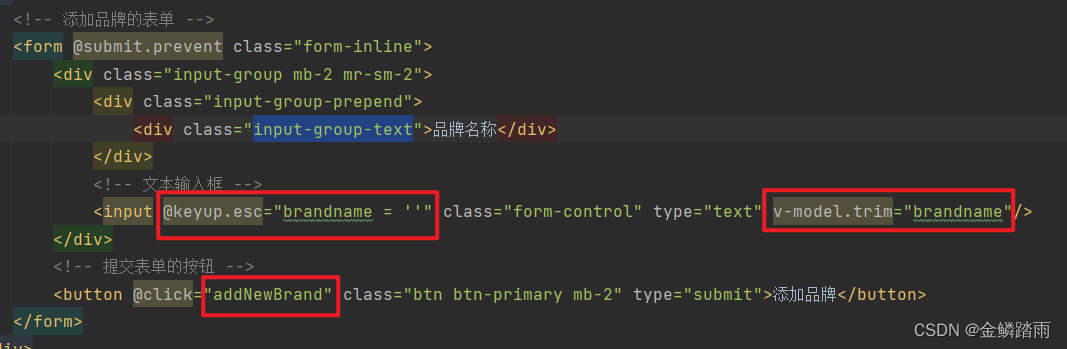
addNewBrand() {
// 判断品牌名称是否为空
if (!this.brandname) return alert('品牌名称不能为空!')
// 添加新的品牌数据
this.brandlist.push({
id: this.nextId,
brandname: this.brandname,
state: true,
addtime: new Date(),
})
// 重置文本框的值
this.brandname = ''
// 让 nextId 自增 +1
this.nextId++
}
删除品牌
为删除的a 链接绑定 click 点击事件处理函数,并阻止其默认行为
<td>
<a @click.prevent="removeBrand(item.id)" href="#">删除</a>
</td>
removeBrand()
removeBrand(id) {
this.brandlist = this.brandlist.filter((x) => x.id !== id)
}