0x00 题目
完全
二叉树是每一层
都是完全填充
除最后一层外
即,节点
数达到最大
的
并且所有的节点都尽可能地集中在左侧
设计一种算法
将一个新
节点插入到一个完整的二叉树中
并在插入后保持其完整
实现 CBTInserter
类:
CBTInserter(TreeNode root)
使用头节点为 root
的给定树初始化该数据结构
CBTInserter.insert(int v)
向树中插入一个值为 Node.val == val
的新节点 TreeNode
使树保持完全二叉树的状态,并返回插入节点 TreeNode
的父节点
的值
CBTInserter.get_root()
将返回树的头节点
0x01 思路
因为是完全
二叉树
可以使用数组
来存储节点
使用层序
遍历方式来遍历与存储节点
子节点与父节点的索引关系如下
left = parent * 2
right = parent * 2 + 1
添加新节点后
可以计算出它的父节点
进一步考虑
由于节点的左右
节点不
为空
时
这个节点已经满
了
不
会再插入
新节点
所以可以从数组中移除
数组中只保留左
、右
节点为空的节点即可
0x02 解法
语言:Swift
树节点:TreeNode
public class TreeNode {
public var val: Int
public var left: TreeNode?
public var right: TreeNode?
public init() { self.val = 0; self.left = nil; self.right = nil; }
public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
self.val = val
self.left = left
self.right = right
}
}
解法:
扫描二维码关注公众号,回复:
14288412 查看本文章
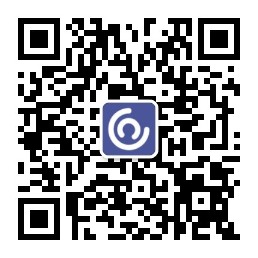
class CBTInserter {
private let root: TreeNode?
private var queue: [TreeNode] = []
init(_ root: TreeNode?) {
self.root = root
if root != nil {
queue.append(root!)
}
while !queue.isEmpty {
let node = queue.first
if let l = node?.left {
queue.append(l)
}
if let r = node?.right {
queue.append(r)
}
if node?.left == nil || node?.right == nil {
break
}else{
// 当 node 左右都不为空时,移除
queue.removeFirst()
}
}
}
func insert(_ val: Int) -> Int {
guard let c = queue.first else { return -1 }
let node = TreeNode(val)
if c.left == nil {
c.left = node
}else if c.right == nil {
c.right = node
}
queue.append(node)
// 右子树不为空,则说明满了
if c.right != nil {
queue.removeFirst()
}
let res = c.val
return res
}
func get_root() -> TreeNode? {
return root
}
}
0x03 我的作品
欢迎体验我的作品之一:小五笔小程序
五笔学习好帮手!
微信搜索 XWubi
即可~