前言
本游戏纯属搞笑,你可以把你朋友的照片设置成贪吃蛇的蛇头,你们看最终结果就知道啦
相关文件
大家可以关注小编的公众号:Python日志
里面会不定时的发布一下Python小知识和一些高校有的源码的
源码获取可以在公众号里面回复:搞笑贪吃蛇 一定不要回复贪吃蛇不然就不是这个源码啦
Python编程小知识教学,入门到精通视频+源码+课件+学习解答加群:773162165
开发环境
Python3.6
模块
Pygame
Config
random
效果展示
恶搞Python版本的贪吃蛇小游戏!笑不活了!!
代码实现
MemeGame类
class MemeGame:
def __init__(self):
pygame.init()
self.config = Config()
pygame.display.set_caption(self.config.game_title)
self.screen = pygame.display.set_mode((
self.config.screen_width,
self.config.screen_height
), pygame.FULLSCREEN)
self.meme = Meme(self)
self.food = Food(self)
self.running = True
def _listen_event(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_DOWN:
if self.meme.direction != 'j':
self.meme.direction = 'k'
elif event.key == pygame.K_UP:
if self.meme.direction != 'k':
self.meme.direction = 'j'
elif event.key == pygame.K_LEFT:
if self.meme.direction != 'l':
self.meme.direction = 'h'
elif event.key == pygame.K_RIGHT:
if self.meme.direction != 'h':
self.meme.direction = 'l'
elif event.key == pygame.K_SPACE:
self.running = True
def _update_screen(self):
self.screen.fill(self.config.screen_bg_color)
def run_game(self):
while True:
pygame.time.delay(100)
self._listen_event()
self._update_screen()
if self.running:
self.meme.blit_meme()
self.food.blit_food()
self.meme.move()
self.meme.eat_food(self.food)
self._show_score(self.meme.len)
if self.meme.is_hit_the_self() or self.meme.is_hit_the_wall():
# die
self.running = False
if self.running == False:
self.meme.reset()
self._show_game_over()
pygame.display.flip()
def _show_score(self, score):
score = score - 3
text_color = (0, 0, 0)
font = pygame.font.SysFont('pingfang', 50)
score_text = font.render(f'你吃了{
score}口热乎的', True, text_color)
self.screen.blit(score_text, (20, 20))
def _show_game_over(self):
text_color = (0, 0, 0)
font = pygame.font.SysFont('pingfang', 50)
text = font.render('游戏结束,按空格键再来一把', True, text_color)
text_rect = text.get_rect()
text_rect.center = self.screen.get_rect().center
self.screen.blit(text, text_rect)
def play_sound(self, sound_name):
sound = pygame.mixer.Sound(f'sound/{
sound_name}.mp3')
pygame.mixer.Sound.play(sound)
Meme类
class Meme():
def __init__(self, meme_game):
self.screen = meme_game.screen
self.config = meme_game.config
self.game = meme_game
self.head = pygame.image.load(self.config.meme_head_image)
self.head = pygame.transform.scale(self.head, self.config.meme_head_size)
self.head_rect = self.head.get_rect()
self.body_rect = pygame.Rect(
0,
0,
self.config.meme_body_block_size,
self.config.meme_body_block_size
)
self.len = 3
self.body = [(100, 200), (130, 200), (160, 200)]
self.head_rect.x = 160 + 15
self.head_rect.y = 200 - 30
self.direction = 'k' # jkhl ---> 下上左右
def blit_meme(self):
for item in self.body:
self.body_rect.x = item[0]
self.body_rect.y = item[1]
pygame.draw.rect(self.screen, self.config.meme_body_color, self.body_rect)
self.screen.blit(self.head, self.head_rect)
def move(self):
head = self.body[0]
if self.direction == 'k':
now_head = (head[0], head[1] + 30)
self.body.insert(0, now_head)
self.head_rect.x = now_head[0] - 15
self.head_rect.y = now_head[1] + 15
elif self.direction == 'j':
now_head = (head[0], head[1] - 30)
self.body.insert(0, now_head)
self.head_rect.x = now_head[0] - 15
self.head_rect.y = now_head[1] - 30 - 30
elif self.direction == 'h':
now_head = (head[0] - 30, head[1])
self.body.insert(0, now_head)
self.head_rect.x = now_head[0] - 30
self.head_rect.y = now_head[1] -30
elif self.direction == 'l':
now_head = (head[0] + 30, head[1])
self.body.insert(0, now_head)
self.head_rect.x = now_head[0] + 15
self.head_rect.y = now_head[1] - 30
if self.len < len(self.body):
self.body.pop()
def eat_food(self, food):
if self.head_rect.colliderect(food.food_rect):
self.len += 1
self.game.play_sound('zhenxiang')
food.rand_food()
def is_hit_the_self(self):
return self.body[0] in self.body[1:]
def is_hit_the_wall(self):
if self.screen.get_rect().collidepoint((self.head_rect.x, self.head_rect.y)):
return False
else:
return True
def reset(self):
self.len = 3
self.direction = 'k'
self.body = [(100, 200), (130, 200), (160, 200)]
Food类
class Food:
def __init__(self, meme_game):
self.config = meme_game.config
self.screen = meme_game.screen
self.game = meme_game
self.food = pygame.image.load(self.config.food_image)
self.food = pygame.transform.scale(self.food, self.config.food_size)
self.food_rect = self.food.get_rect()
self.food_rect.x = 585
self.food_rect.y = 30
def blit_food(self):
self.screen.blit(self.food, self.food_rect)
def rand_food(self):
screen_rect = self.screen.get_rect()
x_num = screen_rect.width // 30
y_num = screen_rect.height // 30
self.food_rect.x = random.randint(0, x_num-1) * 30
self.food_rect.y = random.randint(0, y_num-1) * 30
self.game.play_sound('chishi')
Config类
class Config:
def __init__(self):
self.game_title = '吃shi啦你'
self.screen_width = 0
self.screen_height = 0
self.screen_bg_color = (255, 255, 255)
# meme
self.meme_head_size = (60, 60)
self.meme_head_image = 'images/head.png'
self.meme_body_block_size = 30
self.meme_body_color = (0, 0, 0)
# food
self.food_image = 'images/food.png'
self.food_size = (30, 30)
总结
本游戏是一个纯属搞笑类型的游戏,大家可以学习一下,比较还是比较偏基础的一个Python案例,新手小白比较适合学习
扫描二维码关注公众号,回复:
14139663 查看本文章
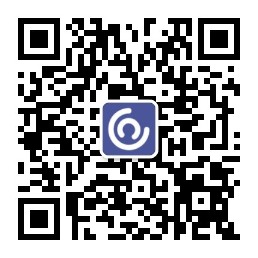
大家可以关注小编的公众号:Python日志
源码获取可以在公众号里面回复:搞笑贪吃蛇 一定不要回复贪吃蛇不然就不是这个源码啦
Python编程小知识教学,入门到精通视频+源码+课件+学习解答加群:773162165