1.The main purpose of lambda expression is to allow you to write inline and anonymous functions.
lambda 函数的作用就是让你在一行里面写出匿名的函数
2.so what are inline anonymous functions?
answer: if you are familiar with the regular functions you know that the main purpose of a function is to allow you to write the code once put it in a function and then whenever you need that code you would just invoke that function. So u do not need to retype the code again.
an inline function in other hand is a function has a very small definition. inline function is usually simple and they are often used for small snippets of code that are very simple and they are not going to be reused like regular functions. so they are not even need to be renamed.
下面的代码是调用algorithm库里面的for_each()functions来实现对于向量里面元素的输出到console:
The outputs:
PS C:\Users\Desktop\C++> & '.\t(1).exe'
2
3
7
14
23
the corresponding codes:
#include <iostream>
#include <stdio.h>
#include <vector>
#include <algorithm>
//there is a for_each() function in algorithm to do thing in every element of
int main(int argc, char **argv)
{
// the shape of the lambda function [](){}
//(angled brackets,parenthesses in square brackets-curly brackets-curly brackets-curly brackets-curly brackets)
//the angled brackets are called capture clause
// these parenthesses are used in order to pass parameters
//the curly brackets are used to contain your function definition
std::vector <int> v{2, 3, 7, 14, 23};
struct{
void operator()(int x){
std::cout << x << std::endl;
}
}something;
std::for_each(v.begin(), v.end(),something);
system("pause > nul");
return 0;
}
上面代码的最大问题,只要一个输出的效果,但是这个对应函数的结构体写的代码比较多,我们可以借用lambda expression to simplfied its shape.
the following codes could realize the same funcion like the code in above.
#include <iostream>
#include <stdio.h>
#include <vector>
#include <algorithm>
//there is a for_each() function in algorithm to do thing in every element of
int main(int argc, char **argv)
{
// the shape of the lambda function [](){}
//(angled brackets,parenthesses in square brackets-curly brackets-curly brackets-curly brackets-curly brackets)
//the angled brackets are called capture clause
// these parenthesses are used in order to pass parameters
//the curly brackets are used to contain your function definition
std::vector <int> v{2, 3, 7, 14, 23};
/*struct{
void operator()(int x){
std::cout << x << std::endl;
}
}something; */
std::for_each(v.begin(), v.end(),[](int x){std::cout << x << std::endl;}); //lambda
//function
system("pause > nul");
return 0;
}
use the lambda function to identify the elemes in vector where is even or odd
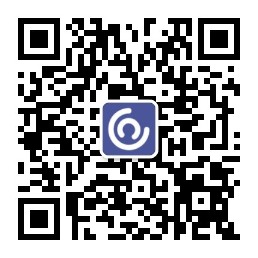
The outputs:
PS C:\Users\Desktop\C++> & '.\t(1).exe'
2is even number
3is odd number
7is odd number
14is even number
23is odd number
the corresponding codes :
#include <iostream>
#include <stdio.h>
#include <vector>
#include <algorithm>
//there is a for_each() function in algorithm to do thing in every element of
int main(int argc, char **argv)
{
// the shape of the lambda function [](){}
//(angled brackets,parenthesses in square brackets-curly brackets-curly brackets-curly brackets-curly brackets)
//the angled brackets are called capture clause
// these parenthesses are used in order to pass parameters
//the curly brackets are used to contain your function definition
std::vector <int> v{2, 3, 7, 14, 23};
/*struct{
void operator()(int x){
std::cout << x << std::endl;
}
}something; */
std::for_each(v.begin(), v.end(),[](int x){
if (x % 2 == 0)
std::cout << x << "is even number" << std::endl;
else
std::cout << x << "is odd number" << std::endl;
});
system("pause > nul");
return 0;
}
The function of content in angled brackets:
The outputs:
PS C:\Users\Desktop\C++> & '.\t(1).exe'
2 could not be divisible by 3
3 could be divisible by 3
7 could not be divisible by 3
14 could not be divisible by 3
23 could not be divisible by 3
The corresponding codes
#include <iostream>
#include <stdio.h>
#include <vector>
#include <algorithm>
//there is a for_each() function in algorithm to do thing in every element of
int main(int argc, char **argv)
{
// the shape of the lambda function [](){}
//(angled brackets,parenthesses in square brackets-curly brackets-curly brackets-curly brackets-curly brackets)
//the angled brackets are called capture clause
// these parenthesses are used in order to pass parameters
//the curly brackets are used to contain your function definition
std::vector <int> v{2, 3, 7, 14, 23};
/*struct{
void operator()(int x){
std::cout << x << std::endl;
}
}something; */
int d = 3;
std::for_each(v.begin(), v.end(),[d](int x){
if (x % d == 0)
std::cout << x << " could be divisible by " << d << std::endl;
else
std::cout << x << " could not be divisible by " << d << std::endl;
});
return 0;
}
how to change the pass value in lambda expressions:
PS C:\Users\Desktop\C++> & '.\t(1).exe'
2 could not be divisible by 3
d = 10
3 could not be divisible by 10
d = 10
7 could not be divisible by 10
d = 10
14 could not be divisible by 10
d = 10
23 could not be divisible by 10
d = 10