在一般的http请求中,post请求是一个绕不过的方法类型,主要是这类请求,可以传输更多更大的参数,甚至是文件。
一般的参数是属于键值对形式,这种普通的参数每一个都有各自的名字和值,这是最简单的form表单形式,后端在取值的时候,需要根据一个一个参数名来获取参数值。
还有一种参数是以body请求体方式传递到后台的,一般可以是一个数组,也可以是一个对象,当作为一个对象的时候,需要封装为json格式,后台就通过一个对象来接收,而不用一个一个参数名来接收所有的参数。这个时候,我们后台代码,使用参数注解就是@RequestBody。
springboot提供了RestTemplate这种http client请求客户端,可以很方便的使用,而不用考虑连接池、重连机制。
===============================================================
使用springboot做一个简单的控制类,分别实现两个方法,一个是form表单提交,一个是body参数提交,来演示今天的主题,并对比两者的不同。
UserController.java
package com.xxx.webapp.controller;
import java.util.HashMap;
import java.util.Map;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.xxx.webapp.entity.User;
@RestController
@RequestMapping(value = "/user")
public class UserController {
@PostMapping("/formSave")
public Map<String, Object> formSave(@RequestParam("username")String username,@RequestParam("password")String password){
Map<String, Object> result = new HashMap<>();
User user = new User();
user.setUsername(username);
user.setPassword(password);
System.out.println("form parameter method run...");
result.put("code", 200);
result.put("data", user);
return result;
}
@PostMapping("/jsonSave")
public Map<String, Object> jsonSave(@RequestBody User user){
Map<String, Object> result = new HashMap<>();
System.out.println("json parameter method run...");
result.put("code", 200);
result.put("data", user);
return result;
}
}
启动程序,使用postman测试两个方法:
1、form表单提
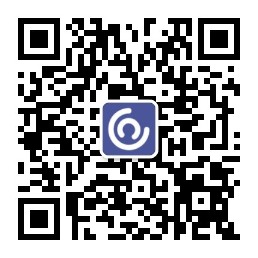
2、json体提交
json体方式提交,参数不能使用form表单了,而是字符串形式的json格式,而且我们要声明header参数Content-Type:application/json。如果不指定ContentType为json格式,那么后台默认会认为他们是普通的文本而抱错:
Content type 'text/plain;charset=UTF-8' not supported
所以一定要做如下所示的请求头设置:
关于这个问题,在后面的代码中还会提到。
============================================================
以上只是做了一些准备工作,准备了两个post方法,可以供我们在后面的代码中测试,还没有到今天的主题,RestTemplate,它对以前的httpclient做了一些封装,本质没有发生变化。
package com.xxx.springboot;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
@SpringBootApplication
//@EnableScheduling
public class App implements CommandLineRunner{
public static final String url = "http://localhost:9000/user/jsonSave";
public static void main( String[] args ){
SpringApplication.run(App.class, args);
}
public static String postForBody() {
String result = "";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON_UTF8);
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode params = objectMapper.createObjectNode();
params.put("username", "buejee2");
params.put("password", "123456");
HttpEntity<String> request = new HttpEntity<String>(params.toString(),headers);
//
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.postForEntity(url, request, String.class);
if(response.getStatusCodeValue()==200) {
result= response.getBody();
}
return result;
}
@Override
public void run(String... args) throws Exception {
String res = postForBody();
System.out.println(res);
}
}
运行程序,打印信息如下:
2021-08-14 11:57:15.091 INFO 18919 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8000 (http) with context path '/springboot'
2021-08-14 11:57:15.207 INFO 18919 --- [ main] com.xxx.springboot.App : Started App in 4.38 seconds (JVM running for 5.256)
{"code":200,"data":{"username":"buejee2","password":"123456"}}
这里因为请求发送的是json体的body参数,所以请求头也要设置Content-Type,另外,不能再使用键值对(form表单)的那种形式传参数。
这里也可以给出一个示例,就是按照form表单形式传递参数。
public static String postForForm() {
String result = "";
HttpHeaders headers = new HttpHeaders();
LinkedMultiValueMap<String, Object> params= new LinkedMultiValueMap<>();
params.add("username", "buejee");
params.add("password", "123456");
HttpEntity<MultiValueMap<String, Object>> request = new HttpEntity<MultiValueMap<String, Object>>(params,headers);
//
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.postForEntity(url, request, String.class);
if(response.getStatusCodeValue()==200) {
result= response.getBody();
}
return result;
}
运行结果就不贴了,这两种方式基本涵盖了post请求的用法。如果参数使用不当,后台接口会报错,媒体类型不支持。这个时候就要排查是不是没有设置正确的请求头和请求体。